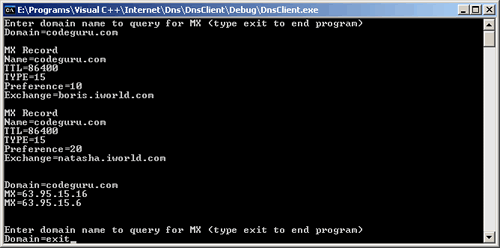
Introduction
I searched for a DNS Resolver for quite a long time. I got the solution, but it was too uncomfortable to use it. Thus, I made my own DNS Resolver; it's is very compact in size, and is free from the exceptions and errors we usually encounter with CSocket
.
DNS Protocol Explanation (RFC 1035)
To query any type of domain name (for example, MX for mail exchange), the request is sent in the form of a binary record having a predefined bits and bytes pattern. Then, the server sends a response. If the server has the answer, it sends answers in the response records; otherwise, it sends other name servers that you then can query and get the answer from them. If other name servers don't have an answer, they in turn will again send other name servers. This process is endless, so we have to query recursively only up to a certain depth (I have chosen a depth of 10).
Library Source Code
The Dns.h file contains all DNS-related source code; it uses other libraries, such as SocketEx.h, SocketClient.h, and NeuroBuffer.h.
Library File Name | Description |
CSocketEx | Socket functions wrapper class (CSocket is very heavy and unreliable if you don't have the exact idea of how it works). All the functions are of the same name as CSocket . You can use this class directly. |
CSocketClient | Derived from CSocketEx and throws proper exceptions with details of Winsock errors. It defines two operators, >> and << , for easy send and receive. It also changes network to host and host to network order of bytes, if required. |
CNeuroBuffer | Because DNS response-request cycles are in binary form, a bytes buffer is used here. |
CDnsBuffer | DNS responses contain domain names; these domain names are compressed with an LZW type of compression. It means that, rather than repeating names, it just gives a pointer to the previous name that occurred in the buffer. So, to extract, it's very important to follow this protocol. The CDnsBuffer class manages this decompression and gives you an expanded domain name. |
** CDnsClient | This is the main class; you can either create an object of CDnsClient to query a single name server without any recursion, or you can call its static members Get and GetMX . It's better that you call static members only. |
CDnsRR | This is the base class of response of the DNS request, which then is derived further for particular types. |
CDnsRRMX | This is the response record of Type MX. It has the Exchange and Preference fields used for MX. |
CDnsRRA | Response record for type A. |
CDnsRRNS | Response record of type NS. |
CDnsRRDefault | I have not programmed all classes of DNS here. I have programmed only particular classes, the rest of which are not programmed. You will find it in the CDnsRRDefault class; it stores the length of the buffer and the data buffer pointer. |
CDnsRRPtrList | When you interact with CDnsClient , the responses are returned in CDnsRRPtrList , which is a CList of the CDnsRR* pointer. You have to be careful while accessing the data of pointer. When CDnsRRPtrList gets destroyed, it automatically deletes all pointers!! So, you must keep a copy of the response record's data, which you need. Because these pointers you get are in the form of CDnsRR* only, you will need to cast according to Type. If you query for MX, you must cast CDnsRRMX * pMX = (CDnsRR*) pRR , and so forth. And then, access data from pMX . You can find the type in the Type field of the response record: "pRR->Type ". |
Note: I don't usually program in the form of different .h and .cpp files because using them somewhere else the next time is a big problem. You have to move both files here and there, so I put all of my code in the .h file only; I don't write to the .cpp file unless it's required. You need only to copy the SocketEx.h, SocketClient.h, NeuroBuffer.h, and Dns.h files into your project's directory, and add the line:
#include "<FONT color=#ff0000>dns.h</FONT>"
after your
#if !defined(.....
and so forth code of your Visual Studio-generated file. If you put it above this, you will get n number of errors.
Here's the main body of code:
[
#include "Dns.h"
void main(int nArgs,char * pcArgs[])
{
CStringList MXs;
CString Log;
Log = CDnsClient::GetMX("codeguru.com",MXs);
while(!MXs.IsEmpty())
{
printf("MX=%s\n",(LPCTSTR)MXs.RemoveHead());
}
CDnsRRPtrList RRs,Additionals;
CStringList NSTried;
int nTries = 10;
BOOL bResult =
CDnsClient::Get(
NULL,
"codeguru.com",
"MX",
RRs,
Additionals,
NSTried,
Log,
nTries
)
POSITION Pos = RRs.GetHeadPosition();
while(Pos)
{
CDnsRR * pRR = RRs.GetNext(Pos);
if(pRR->Type!=CDnsQueryType::GetType("MX"))
continue;
CDnsRRMX * pRRMX = (CDnsRRMX*) pRR;
printf("MX=%s\n",(LPCTSTR)MXs.RemoveHead());
}
}
]
More about me.