Introduction
Finally I found some free time to make ECG_1 more useful. So now, ECG_1 application is running on Windows NT, XP, and 2000 operating systems, and can collect data in real time. To accomplish this, you must download and install free driver for low level in/out ports access. But functions are predefined. Because this is not the end version, I made it for friends who have to convert data from medical records in to TXT format.
And, let’s go… If you have questions, refer to my first article, ECG recording, storing, filtering and recognition.
The new things are: drvECG_1.dll and ECG_1.exe with capabilities to store data in a TXT file. First download and compile source files, or just download a demo project. To run NT Windows support you will need first to download a “DriverLINX Port I/O Driver for Win95 and WinNT” – it is free, and can be done after a registration here.
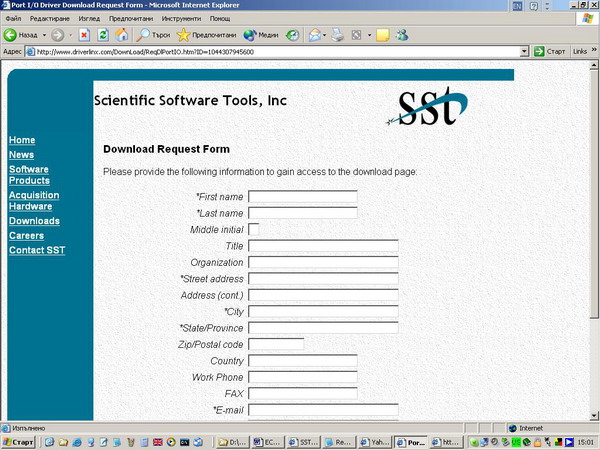
After this, you may need to install the driver, simply click on just downloaded file. In future I’m planning to build my own driver, but for this moment this one works well. Follow the instructions and confirm installation by clicking on Next button.
Do not change anything, just click Next.

After installing the port access driver, you need to restart the PC, this will be done automatically. After restarting the PC, you can start ECG_1 application by clicking on its icon - ECG_1.exe.
To use the program, you may need to open files with data. So use the download link to get an data.ecg file. After this, use the menu File->Open to open the open file name dialog.

Select a file data.ecg and click OK or Open. After this, you will be asked to confirm the record, so do this.

After this operation, the data.ecg file will be opened and you can move inside the records:

To save all records in to TXT format you need to use: File->ExportData->Export All Records to TXT, or if you want to export only the selected record to TXT file, use: File->ExportData->Export Current Record to TXT.

If you use Export Current Record, the program will show you the exact number of the selected records, so click OK.

After this, Save File dialog will be opened to ask you to specify the file name and location on the HDD.

So write a test file name and click OK to save the current record, or all the records to a TXT file. The separator between each point in the record is “ ; ”. Note this when opening TXT file. Now you can open the just saved TXT file to view it in notepad.

You can see the separators – “ ; “.
Now to use your data in Microsoft Excel program to perform some extra calculations on it, open MS Excel program and click on File->Open and select your just saved test.txt data file from ECG_1 program and follow the pictures. Because this TXT is a text file, and MS Excel does not support such files directly. You may need to specify in the Open File dialog that you need to see all files, so do it. After this, find on your HDD just saved data.txt file and click Open.

You need to go on a little procedure to accommodate TXT file in to Excel file. So click OK on the upper screen.

Check the Delimiter properties and click Next button.

Select the check box Other and in the input field write a “ ; “. Click Finish to accomplish the procedure.

Now your Excel will contain a single row of values. To make a graph from them go in the Insert Menu and select Chart.

Select chart type as it is shown on the upper picture, then you will need to click next and specify the data to draw.

To do this, click one tile in the data range field, and after this, click before row 1 to select all records in the first row. Click Finish.

Now you can make additional calculations on your file. Because Excel do not support larger data fields, you may need to use Math Cad to use TXT data for additional calculations. I’m not going to discuss this now. But try it. Now is time for some code. To see the full explanations, on ECG_1 code search for my first article in CodeProject. In ECG_1, I added some new functions to support data.TXT file saving.
void CECG_Data::SaveDataTXT(CString File_Name)
{
CFile sFile(File_Name,CFile::modeCreate|CFile::modeWrite);
CArchive dArchive(&sFile,CArchive::store);
Serialize_DATA_TXT(&dArchive);
}
This function uses the next one function which is created to make the conversion to CString
from CWordArray DATA
array that is used in ECG.exe to store the medical records data.
void CECG_Data::Serialize_DATA_TXT(CArchive *ar)
{
int i=0;
CString str;
CString stmp;
DATA_TXT.RemoveAll();
str = "Start from ECG_1 data file;";
for(int j=0;j<DATA.GetSize();j++)
{
stmp.Empty();
char ch[5];
i = int(short(DATA.GetAt(j)));
_itoa(i, ch, 10 );
for(int k=0;k<4;k++)
{
stmp = stmp + ch[k];
}
stmp = stmp + ";";
str = str + stmp;
}
DATA_TXT.Add(str);
DATA_TXT.Serialize(*ar);
}
Important: This loop can take a lot of time!
This one converts CWordArray DATA
array into one CStringArray DATA_TXT
array. I’m using CStringArray
instead of a single CString
variable, because it doesn’t support Serialize
function directly but CStringArray
support it. One additional function is:
void CECG_Data::SaveCurDataRecTXT(CString File_Name)
{
CFile sFile(File_Name,CFile::modeCreate|CFile::modeWrite);
CArchive dArchive(&sFile,CArchive::store);
Serialize_Cor_DATA_TXT(&dArchive);
}
It saves the selected records only! But before using it, we set the current position in the data field – selected record.
void CECG_Data::SetCurSaveTXTPos(int pos)
{
save_to_txt_cur_rec_pos = pos;
}
Now we can serialize data in to TXT format. This function saves only the selected medical records into TXT file, so it takes much shorter time than the upper one.
void CECG_Data::Serialize_Cor_DATA_TXT(CArchive *dArchive)
{
if(save_to_txt_cur_rec_pos+2500<=GetAllDataLenght())
{
int i=0;
CString str;
CString stmp;
DATA_TXT.RemoveAll();
str = "Start from ECG_1 data file;";
for(int j=save_to_txt_cur_rec_pos;j<
save_to_txt_cur_rec_pos+2500;j++)
{
stmp.Empty();
char ch[5];
i = int(short(DATA.GetAt(j)));
_itoa(i, ch, 10 );
for(int k=0;k<4;k++)
{
stmp = stmp + ch[k];
}
stmp = stmp + ";";
str = str + stmp;
}
DATA_TXT.Add(str);
DATA_TXT.Serialize(*dArchive);
}
else
MessageBox(NULL,"Selected record to save out of data lenth.",
NULL,MB_OK);
}
Calculations on TXT files take a lot of time, so I made this feature in ECG.exe to support some of you with the ability to use my data files in Mat Lab, Mat Cad, Excel and other programs that you may use every day. But my opinion is that txt files are not a good choice for storing data. Many programs offer this capabilities and I found it useful to make this feature in ECG.exe.
History