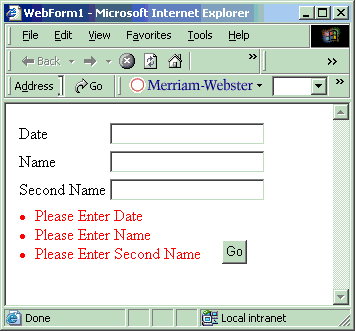
Introduction
Initially, I was impressed by ASP.NET Validation controls. But as I started using these controls, I got annoyed by the fact that we can not use the same validation control for more than one control. So if you have more "required fields", you will end up with more number of RequiredFieldValidator
s. The second problem was we can not mimic the old day JavaScript validation which will alert the user for specific field and set the focus on it. So, I decided to create one custom Validation Control which can serve both purposes.
Using the code
This control is very easy to use, much similar to normal RequiredFieldValidator
control.
If you have only one required field, this control will behave similar to the RequiredFieldValidator
control.
If you have a group of controls to validate, then use AddControlToValidate (TextBox, ErrorMessageToBeDisplay)
on Page_Load
. You can set alert type also. By default, it will popup alert message to user and set the focus to the required field.
private void Page_Load(object sender, System.EventArgs e)
{
GroupReqValidator1.AddControlToValidate(TextBox1, "Please Enter Date ");
GroupReqValidator1.AddControlToValidate(TextBox2, "Please Enter Name");
GroupReqValidator1.AddControlToValidate(TextBox3, "Please Enter Surname");
}
Points of Interest
So, let's start unwrapping the magic behind this control. It's very simple. As I want to build a group RequiredFieldValidator control, I inherited my class from System.Web.UI.WebControls.RequiredFieldValidator
, so that we will get all basic functions implemented.
First of all, we require some kind of collection to store the references for the control(s) to be validated and the appropriate message to be displayed. So, I used ArrayList
, and a variable to store the display style.
private System.Collections.ArrayList objList=
new System.Collections.ArrayList();
private System.Collections.ArrayList arrErrorMessage=
new System.Collections.ArrayList();
private int iAlertType=0;
Next, create a function to add control and message to this ArrayList
.
public void AddControlToValidate(TextBox objCtl,string strErrorMessage)
{
objList.Add(objCtl);
arrErrorMessage.Add(strErrorMessage);
}
Next, we need to generate client script to validate all controls and display alert if needed. I did this job in OnPreRender
function.
System.Text.StringBuilder strJavaScript=new System.Text.StringBuilder();
strJavaScript.Append(@"<script JavaScript?? langauge="">");
strJavaScript.Append(@"function ValidateGroupControl(){var msg='<ui>';");
for(int i=0;i<objList.Count;i++)
{
strJavaScript.Append(@"if( document.all['"+
((TextBox)objList[i]).ID+"'].value==''){");
if(iAlertType==0)
{
strJavaScript.Append(@"alert('"+ (string)arrErrorMessage[i] +
"');document.all['"+((TextBox)objList[i]).ID+
"'].focus();return false;}");
}
else
{
strJavaScript.Append(@"msg=msg+'<li>"+
(string)arrErrorMessage[i]+"</li>';}");
}
}
if(iAlertType==1)
{
strJavaScript.Append(@"if(msg!=''){document.all['"+
this.ID+"'].innerHTML=msg+'</ui>';return false;}");
}
strJavaScript.Append(@"return true;}</script>");
Page.RegisterClientScriptBlock("funValidate",strJavaScript.ToString());
Once script is registered, we need to call this script when the Form is submitted. That we can do using RegisterOnSubmitStatement
function to register.
protected override void OnInit(EventArgs e)
{
base.OnInit (e);
Page.RegisterOnSubmitStatement("GroupValidation",
"return ValidateGroupControl();");
}
So far so good. Now, client side validation is done. But what if JavaScript is disabled? So, we have to implement server side validation, too. We can do this by implementing EvaluateIsValid
.
protected override bool EvaluateIsValid()
{
if(objList.Count==0)
{
string controlValue = GetControlValidationValue(ControlToValidate);
if (controlValue == null)
{
return true;
}
return !controlValue.Trim().Equals(InitialValue.Trim()) ;
}
else
{
bool result=true;
string strmsg="";
for(int i=0;i < objList.Count;i++)
{
if(((TextBox)objList[i]).Text.Trim().Equals(InitialValue.Trim()))
{
strmsg+="\n"+(string)arrErrorMessage[i];
result=false;
}
}
this.ErrorMessage = strmsg;
return result;
}
}
Here you go.. Build this control library, add reference to your project, and enjoy validating. Do drop me comments ..please.
History
- Created on 4th October, 2004.