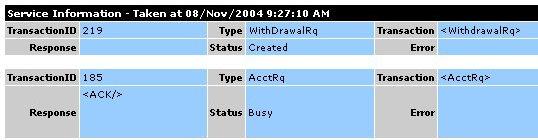
Introduction
This article is about exposing .NET Windows Service status information.
Background
In the old C++ ATL Service days, you could expose a COM interface and add properties and methods accessible by any application. To reproduce this concept in a .NET Windows Service, I started looking at all kinds of custom methods and solutions. I was writing an MSMQ transaction system, and my customer wanted to know what the status of the queues were, i.e., what transactions were pending, what had completed, and number of transactions processed. All of which could have been solved with data stored in a database, but I found a simpler way, WMI. I created a status class that kept track of information I wanted to store about the Queue my Windows service was managing.
Using the code
There are restrictions to using the Instrumentation
class. Basically, anything you want to expose must be a public property of the class and the type that is exposed must be serializable. Read MSDN about the Instrumentation class. Read the WMI SDK for more information about WMI.
To expose information to WMI, the instrumentation utility allows you to create a namespace:
[assembly:Instrumented("root/MyService")]
namespace WindowsService
{ ...
}
This is the namespace used to perform WMI queries against.
[ InstrumentationClass(InstrumentationType.Instance) ]
public class ServiceContext
{ ....
}
This will be the class that exposes its public properties to WMI. Your WMI queries will select against this class name. The attached source code contains an ASP page showing an example of this selection.
public enum eStatus
{
Created,
Populated,
Sent,
Received,
Completed,
NoRecord,
Error
}
[ InstrumentationClass(InstrumentationType.Instance) ]
public class ServiceContext
{
private string m_ResponseDetails = String.Empty;
private eStatus m_Status = eStatus.Created;
[XmlElement]
public string TransactionType;
[XmlElement]
public string TransactionXML;
[XmlElement]
public string ResponseXML
{
set
{
if (value!=null)
PopulateResponse((string)value);
}
get
{
return m_ResponseDetails;
}
}
[XmlElement]
public string ErrorSource;
[XmlElement]
public string ErrorDescription = String.Empty;
[XmlElement]
public string ContextID = String.Empty;
public int Status
{
get { return (int)m_Status; }
set { UpdateStatus((eStatus)value); }
}
.
.
.
}
The class above contains the fuller listing of the class and the work around I did for an enum
type. The final piece to the puzzle is publishing the class to WMI Instrumentation.Publish(oContext);
, and releasing the class from WMI Instrumentation.Revoke(oContext);
.
This can be done in your constructor and inheriting from IDisposable
in your destructor.
My problem was that I only wanted the class removed from the WMI list after a result had been returned from MSMQ, so my publish and revoke calls were made in a Queue managing class which works well from the user's point of view.
Points of Interest
If Microsoft implemented a WMI method call and made the public properties writable, they would have completed the gaps between an ATL Service and a .NET Windows Service. But alas! This is not the case. Hopefully, a future implementation of the framework will address this, but for the time being, I'll see if I can solve it.
I studied IT in 1987 and have been working ever since in on projects that range from coding animation in pascal and assembler to writing secure internet banking web sites.
I live in South Africa and I think because of it's size we're required to do more for less which makes highly skilled in a wide range of skills and technologies.
I have the bonus of living in a coastal town so time away from computers is spent around the sea and in sunshine and nature.