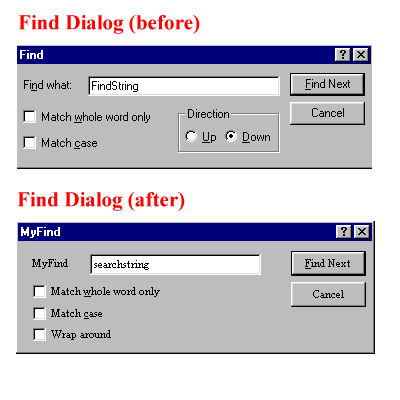
Introduction
This article explains how to customize the standard Find/Replace Dialog in RichEdit view. The logic is same for Edit view or any other generic views with slight changes. Customizing the Find/Replace Dialog involves modifying Windows common Find/Replace dialog template which allows you to add any new controls or remove the existing one. Please note, none of the controls in the original Find/Replace dialog template should be deleted instead, you can disable or hide those unwanted controls!
Find/Replace common Dialog customization
Customizing Find/Replace common dialog involves the following steps:
Step 1
Copy the Find/Replace dialog template from common dialog .RC to the application's .RC file. This dialog template resides in the file include\findtext.dlg
Step 2
Make any necessary changes to the copied dialog template and note again none of the controls in the original Find/Replace dialog template should be deleted, instead you can disable or hide those unwanted controls.
The demo application which is included with this article demonstrates the following:
How to hide a control?
Direction (up/down) control is hidden
How to add a new control?
New control "[ ] Wrap around" is added
How to change its properties?
Dialog's default font has been changed
Step 3
Use Classwizard to add a C++ class for our new Find/Replace template (say, CMyFindDlg
). Drive this new class from CDialog
as the base class. Then change all reference from CDialog
to CFindReplaceDialog
in both header and implementation file of the newly created class.
class CMyFindDlg : public CFindReplaceDialog
{
public:
CMyFindDlg(CWnd* pParent = NULL);
enum { IDD = IDD_MYFINDDLG };
protected:
virtual void DoDataExchange(CDataExchange* pDX);
protected:
afx_msg void OnCheck1();
DECLARE_MESSAGE_MAP()
};
Step 4
Change the constructor of CFindReplaceDialog
which differs from the CDialog
's constructor.
CMyFindDlg::CMyFindDlg(CWnd* pParent )
: CFindReplaceDialog()
{
}
Step 5
Create a new Menu item and a Toolbar button for our Find and add a handler function for command message (say, OnMyFind
). While creating the Find dialog we can hide the unwanted controls.
void CMyFindView::OnMyFind()
{
m_pMyFindDialog= new CMyFindDlg();
m_pMyFindDialog->m_fr.lpTemplateName=MAKEINTRESOURCE(IDD_MYFINDDLG);
m_pMyFindDialog->Create(TRUE,NULL,NULL,
FR_ENABLETEMPLATE|FR_HIDEUPDOWN,this);
m_pMyFindDialog->SetActiveWindow();
m_pMyFindDialog->ShowWindow(TRUE);
}
Where m_pMyFindDialog
is defined in MyFindView.h
CMyFindDlg* m_pMyFindDialog;
Step 6
When Find/Replace dialog is opened the user can edit or type the search string, change the check marks of controls or press any buttons. So we should process those requests and necessary action to be taken. Actually CFindReplaceDialog
sends a message to its parent and you must specify the FINDMSGSTRING
control in a call to the RegisterWindowMessage
function to get the identifier to the message, sent by the FindReplace dialog box. Also add an ON_REGISTER_MESSAGE
entry for message handler.
static UINT FindReplaceDialogMessage =
::RegisterWindowMessage(FINDMSGSTRING);
ON_REGISTERED_MESSAGE(FindReplaceDialogMessage, OnFindReplaceMessage)
Here is the Find/Replace dialog message handler function:
LRESULT CMyFindView::OnFindReplaceMessage(WPARAM wParam, LPARAM lParam)
{
CFindReplaceDialog* pFindReplace =
CFindReplaceDialog::GetNotifier(lParam);
ASSERT(pFindReplace != NULL);
if (pFindReplace->IsTerminating())
{
pFindReplace = NULL;
}
else if (pFindReplace->FindNext())
{
if(FindText(pFindReplace->GetFindString(),FALSE,FALSE))
AdjustDialogPosition(pFindReplace);
else
TextNotFound(pFindReplace->GetFindString());
}
return 0;
}
Conclusion
The purpose of this article is to demonstrate how to customize the standard Find/Replace dialog in a MFC application and how to handle the Find/Replace dialog box messages. A sample application with source files is also included with this article.
Working as an Senoir Engineering Manager in a California/USA based company and having 15 years of design and development experience on UNIX/Windows based platforms. Proficient in C, C++, Visual C++, MFC, Web based development and networking.