Table of Contents
First of all I want to thank Sean Ewington for his great initiative to write Beginner's Walk for Web Development article. I have decided to write some articles on state management There are a few article on Code project on State Management, basically on Session, Caching, Cookies, etc. Though all are very good article, still I have planned for write some article on state management. and I believe that should definitely helps to all the Beginners. And I have organized the content in a way that it would be helpful to not only beginners also to advance user also.
In this article, I will cover the fundamentals of State Management and Details of View State.
Web is Stateless
. It means a new instance of the web page class is re-created each time the page is posted to the server. As we all know HTTP
is a stateless protocol, its can't holds the client information on page. As for example , if we enter a text and client on submit button, text does not appear after post back , only because of page is recreated on its round trip.
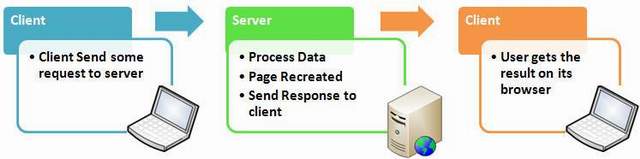
As given in above pages, page is recreated before its comes to clients and happened for each and every request. So it is a big issue to maintain the state of the page and information for a web application. That is the reason to start concept of State Management
. To overcome this problem ASP.NET 2.0 Provides some features like View State, Cookies, Session, Application objects
etc. to manage the state of page.
There are some few selection criteria to selected proper way to maintain the state, as there are many way to do that. Those criteria are:
- How much information do you need to store?
- Does the client accept persistent or in-memory cookies?
- Do you want to store the information on the client or on the server?
- Is the information sensitive?
- What performance and bandwidth criteria do you have for your application?
- What are the capabilities of the browsers and devices that you are targeting?
- Do you need to store information per user?
- How long do you need to store the information?
- Do you have a Web farm (multiple servers), a Web garden (multiple processes on one machine), or a single process that serves the application?
So, when ever you start to think about state management, you should think about above criteria. based on that you can choose the best approaches for manages state for your web application.
There are two different types of state management:
- Client Side State Management
View State
Hidden Field
Cookies
Control State
- Server Side State Management
Session
Application Object
Caching
Database
Client Side state management does not use any server resource , it store information using client side option. Server Side state management use server side resource for store data. Selection of client side and server side state management should be based on your requirements and the selection criteria that are already given.
View State
is one of the most important and useful client side state management mechanism. It can store the page value at the time of post back (Sending and Receiving information from Server)
of your page. ASP.NET pages provide the ViewState
property as a built-in structure for automatically storing values between multiple requests for the same page.
Example:
If you want to add one variable in View State,
ViewState["Var"]=Count;
For Retrieving information from View State
string Test=ViewState["TestVal"];
Sometimes you may need to typecast ViewState Value to retreive. As I give an Example to strore and retreive object in view state in the last of this article.
This are the main advantage of using View State:
- Easy to implement
- No server resources are required
- Enhanced security features ,like it can be encoded and compressed.
This are the main disadvantages of using View State:
- It can be performance overhead if we are going to store larger amount of data , because it is associated with page only.
- Its stored in a hidden filed in hashed format (which I have discussed later) still it can be easily trapped.
- It does not have any support on mobile devices.
I already describe the criteria of selecting State management. A few point you should remember when you select view state for maintain your page state.
- Size of data should be small , because data are bind with page controls , so for larger amount of data it can be cause of performance overhead.
- Try to avoid storing secure data in view state

You won't need view state for a control for following cases,
- The control never change
- The control is repopulated on every postback
- The control is an input control and it changes only of user actions.
View State stored the value of page controls as a string which is hashed and encoded in some hashing and encoding technology. It only contain information about page and its controls. Its does not have any interaction with server. It stays along with the page in the Client Browser. View State use Hidden
field to store its information in a encoding format.
Suppose you have written a simple code , to store a value of control:
ViewState["Value"] = MyControl.Text;
Now, Run you application, In Browser, RighClick
> View
Source
, You will get the following section of code

Fig : View state stored in hidden field
Now , look at the value. looks likes a encrypted string, This is Base64 Encoded string, this is not a encoded string. So it can easily be decoded. Base64 makes a string suitable for HTTP transfer plus it makes it a little hard to read . Read More about Base64 Encoding . Any body can decode that string and read the original value. so be careful about that. There is a security lack
of view state.
We can store an object easily as we can store string or integer type variable. But what we need ? we need to convert it into stream of byte. because as I already said , view state store information in hidden filed in the page. So we need to use Serialization
. If object which we are trying to store in view state ,are not serializable , then we will get a error message .
Just take as example,
[Serializable]
public class student
{
public int Roll;
public string Name;
public void AddStudent(int intRoll,int strName)
{
this.Roll=intRoll;
this.Name=strName;
}
}
Now we will try to store object of "Student
" Class in a view state.
student _objStudent = new student();
_objStudent.AddStudent(2, "Abhijit");
ViewState["StudentObject"] = _objStudent;
student _objStudent;
_objStudent = (student)ViewState["StudentObject"];
If you want to trace your view state information, by just enable "Trace
" option of Page Directive

Now Run your web application, You can view the details of View State Size along with control ID in Control Tree
Section. Don't worry about "Render Size Byte
" , this only the size of rendered control.

Fig : View State Details
You can enable and disable View state for a single control as well as at page level also. To turnoff view state for a single control , set EnableViewState
Property of that control to false. e.g.:
TextBox1.EnableViewState =false;
To turnoff the view state of entire page, we need to set EnableViewState
to false of Page Directive as shown bellow.

Even you disable view state for the entire page , you will see the hidden view state tag with a small amount of information, ASP.NET always store the controls hierarchy for the page at minimum , even if view state is disabled.
For enabling the same, you have to use the same property just set them as True
as for example, for a single control we can enabled view state in following way,
TextBox1.EnableViewState =true;
and for a page level,

As I already discuss View state information is stored in a hidden filed in a form of Base64 Encoding
String, and it looks like:

Fig : View state stored in hidden field
Many of ASP.NET Programmers assume that this is an Encrypted format
, but I am saying it again, that this is not a encrypted string. It can be break easily. To make your view state secure, There are two option for that,

A hash code
, is a cryptographically strong checksum
, which is calculated by ASP.NET and its added with the view state content and stored in hidden filed. At the time of next post back, the checksum data again verified , if there are some mismatch, Post back will be rejected. we can set this property to web.config file also.

It ViewStateEncryptionMode
has three different options to set:
Always
, mean encrypt the view state always, Never
means, Never encrypt the view state data and Auto
Says , encrypt if any control request specially for encryption. For auto , control must call Page.RegisterRequiresViewStateEncryption()
method for request encryption.
we can set the Setting for "EnableViewStateMAC"
and ViewStateEncryptionMode"
in web.config also.

Note :
Try to avoid View State Encryption if not necessary , because it cause the performance issue.
Questions | Answer |
Client Side or Server Side ? | Client Side |
Use Server Resource ? | No |
Easy to implement ? | Yes |
Cause Performance Issue ? | For heavy data and case of encryption & decryption |
Support Encryption Decryption? | Yes |
Can store objects ? | Yes, but you need to serialize the class. |
Timeout | No |
That's all for view state. Hope you have enjoyed this article, please don't forget to give me your valuable suggestions. If anything need to update or changed please post your comments and please give me suggestion.
Reference
History
Written on Saturday, 29th November, 2008
Small Correction on Monday December 2008