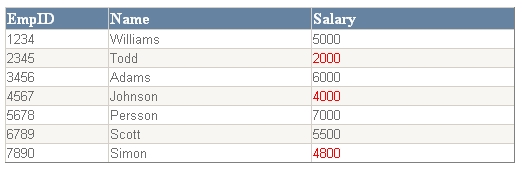
I've searched the internet for examples of datagrids and how to change one or more cell properties to another background or font color. A lot of people in forums said it isn't possible, but finally it seems quite simple. Combining different articles and examples gave me the solution I wanted.
Introduction
This article describes how to create a datagrid and change the color of one or more cells based upon one or more conditions. This article contains very simple code of ASP.NET.
Using the code
What we want is a datagrid with employees. Employees with a salary less than 5000 must be displayed in red. We use a XML-file to display in the datagrid.
First we create a datagrid on our aspx page.
<asp:DataGrid id="dgEmployees" runat="server" AutoGenerateColumns="False" OnItemDataBound="dgBoundItems">
<AlternatingItemStyle CssClass="datagridAltItem">
<ItemStyle CssClass="datagridItem">
<HeaderStyle CssClass="datagridHeader">
</asp:DataGrid>
Then we load the XML-file into the dataset in the pageload. Then we bound the columns into the datagrid in de code behind page, so we can set some properties.
public DataSet ds = new DataSet();
private void Page_Load(object sender, System.EventArgs e)
{
ds.ReadXml(MapPath("employees.xml"));
DataView dv1 = new DataView();
dv1.Table = ds.Tables[0];
BoundColumn datagridcol;
datagridcol = new BoundColumn();
datagridcol.HeaderText = "EmpID";
datagridcol.HeaderStyle.Width = Unit.Pixel(100);
datagridcol.ItemStyle.Width = Unit.Pixel(100);
datagridcol.DataField = "ID";
dgEmployees.Columns.Add(datagridcol);
datagridcol = new BoundColumn();
datagridcol.HeaderText = "Name";
datagridcol.HeaderStyle.Width = Unit.Pixel(200);
datagridcol.ItemStyle.Width = Unit.Pixel(200);
datagridcol.DataField = "Name";
dgEmployees.Columns.Add(datagridcol);
datagridcol = new BoundColumn();
datagridcol.HeaderText = "Salary";
datagridcol.HeaderStyle.Width = Unit.Pixel(200);
datagridcol.ItemStyle.Width = Unit.Pixel(200);
datagridcol.DataField = "Salary";
dgEmployees.Columns.Add(datagridcol);
dgEmployees.DataSource = dv1;
dgEmployees.DataBind();
dgEmployees.Visible = true;
}
So now the datgrid is filled with the information from the XML-File. Now we want the salaries displayed in red when they are less than 5000. We can do this in the OnItemDataBound. When binding the datagrid the procedure dgBoundItems is called. Here we can check every cell of the table that will be created. With GetCellIndex
we get the columnnumber of the table. We only have to check the third column, so GetCellIndex
must be equal to 2. The DataSetIndex
gives the rownumber. Rownumber 0 is the header. We want to start after the header, so DataSetIndex
must be greater than 0. Finally we check if the salary is less than 5000. We change the cell fore-color into red.
public void dgBoundItems(object sender, DataGridItemEventArgs e)
{
foreach(TableCell cell in e.Item.Cells)
{
if (e.Item.Cells.GetCellIndex(cell) == 2 && e.Item.DataSetIndex > 0)
{
if (Convert.ToInt32(cell.Text.Trim()) < 5000)
{
cell.ForeColor = Color.Red;
}
}
}
}
Conclusion
A simple way to change the text-color in a cell. With this example in mind it is possible to change a lot of properties of a single cell. Background-color, mouse-over actions, etc.
Hope my example helps a lot of other people.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.