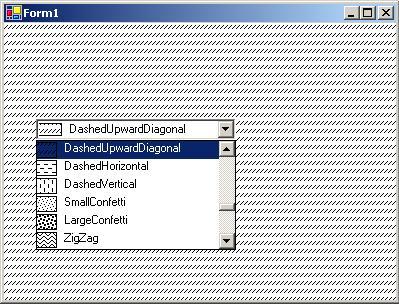
Introduction
In this article we will see how to write a HatchStyle
dropdown. Typically, Windows allows us to draw the items ourselves in the ComboBox. We can use DrawItem
and MeasureItem
events to provide the ability to override the automatic drawing and we use Drawmode
property to draw the items ourselves by setting this property to OwnerDrawVariable
. Double-buffering prevents flicker caused by the redrawing of the control. To fully enable double-buffering, you must also set the UserPaint
and AllPaintingInWmPaint
bits to true
.
public HSComboBox(): base()
{
this.DrawMode = DrawMode.OwnerDrawVariable;
this.SetStyle(ControlStyles.DoubleBuffer, true);
this.InitializeDropDown();
}
By doing this, Windows will send us DrawItem
and MeasureItem
events for each item added to the ComboBox.
protected override void OnDrawItem(System.Windows.Forms.DrawItemEventArgs e)
{
e.DrawBackground();
if (e.Index != -1)
{
Graphics g = e.Graphics;
Rectangle r = e.Bounds;
Rectangle rd = r;
rd.Width = rd.Left + 25;
Rectangle rt = r;
r.X = rd.Right;
string displayText = this.Items[e.Index].ToString();
HatchStyle hs = (HatchStyle)
Enum.Parse(typeof(HatchStyle),displayText, true);;
HatchBrush b = new HatchBrush(hs, Color.Black, e.BackColor);
g.DrawRectangle(new Pen(Color.Black, 2), rd.X + 3,
rd.Y + 2, rd.Width - 4, rd.Height - 4);
g.FillRectangle(b, new Rectangle(rd.X + 3, rd.Y + 2,
rd.Width - 4, rd.Height - 4));
StringFormat sf = new StringFormat();
sf.Alignment = StringAlignment.Near;
if((e.State & DrawItemState.Focus)==0)
{
e.Graphics.FillRectangle(new
SolidBrush(SystemColors.Window), r);
e.Graphics.DrawString(displayText, this.Font,
new SolidBrush(SystemColors.WindowText), r, sf);
}
else
{
e.Graphics.FillRectangle(new
SolidBrush(SystemColors.Highlight), r);
e.Graphics.DrawString(displayText, this.Font,
new SolidBrush(SystemColors.HighlightText), r, sf);
}
}
e.DrawFocusRectangle();
}
protected override void
OnMeasureItem(System.Windows.Forms.MeasureItemEventArgs e)
{
string displayText = this.Items[e.Index].ToString();
SizeF stringSize=e.Graphics.MeasureString(displayText, this.Font);
stringSize.Height += 5;
e.ItemHeight = (int)stringSize.Height;
e.ItemWidth = (int)stringSize.Width;
}
Hatch style Dropdown
The HatchStyle
enumeration specifies the hatch pattern used by a brush of type HatchBrush.
The hatch pattern consists of a solid background color and lines drawn over the background. The iteration below inserts all the hatch patterns into the dropdown:
protected void InitializeDropDown()
{
foreach (string styleName in Enum.GetNames(typeof(HatchStyle)))
{
this.Items.Add(styleName);
}
}
We start by creating a Windows application. Add HatchStyle
ComboBox to the form. Add the following lines to the Paint
event of the form. The Invalidate
method of the form invalidates a specific region of the form and causes a Paint message to be sent to the form.
private void Form1_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
if (hsComboBox1.SelectedItem != null)
{
Graphics g = e.Graphics;
HatchStyle hs = (HatchStyle)Enum.Parse(typeof(HatchStyle),
hsComboBox1.SelectedItem.ToString(), true);
HatchBrush b = new HatchBrush(hs, Color.Black, this.BackColor);
g.FillRectangle(b, new Rectangle (0,0,this.Width,this.Height));
}
}
private void hsComboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
if (hsComboBox1.SelectedItem != null)
{
this.Invalidate();
}
}
That's it!