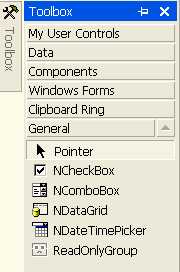
Figure 1 - Noogen.WinForms.dll added to the Toolbox.
Introduction
This article is for those who are still working in .NET 1.1 and are in need of:
- An auto-complete
ComboBox
.
- A working
DataGridComboBoxColumn
, not the one that is inherited from the existing buggy DataGridTextBoxColumn
.
- A
DataGridCheckBoxColumn
that supports single click.
- Bindable
CheckBox
.
- Bindable
DateTimePicker
.
- To be able to make any control
ReadOnly
like the existing property on a TextBox
without having to inherit every single existing control (Decorator pattern).
- Designer support for the newly created
DataGridColumnStyles
.
First of all, I understand that .NET 2.0 will most likely make 90% of these controls obsolete. I was motivated to code this because my company is in immediate need of these controls in .NET 1.1, they won't run production materials on .NET 2.0 beta, and would rather wait for the final release of .NET 2.0 than purchase third party products.
Features
NCheckBox
- is a bindable CheckBox
.
NComboBox
AutoComplete
- enable hint during input.
ShowDropDownDuringInput
- force DropDown to display during input.
ClearSelection
- Clear text selection when the control is not in focus.
DisableEntryNotInList
- disable entry if the value is not in list. (Including disable of paste - by ContextMenu
and Ctrl+V.)
CharacterCasing
- provide the missing CharacterCasing
property as seen in existing TextBox
control.
- Bug fix - allow reset
SelectionIndex
to -1 when the Text is empty.
NDateTimePicker
- provide a BindableValue
property and allow null
to bind as MinDate
.
ReadOnlyGroup
- Simulate
ReadOnly
property on any existing control. This is because the Enabled
property makes it hard to read with gray text.
- Allow
Color
to indicate control in ReadOnly
state.
NDataGrid
- provide rich designer support for the newly created
DataGridColumnStyles
columns.
- Allow for double-click with a key combination (default
Keys.Control
).
DataGridTextBoxColumnEx
- Extended the existing DataGridTextBoxColumn
for bug fixing and custom Color
formatting.
DataGridComboBoxColumn
- Add ComboBox
and auto-complete support by implementing NComboBox
. It was also re-written from scratch by deriving directly from DataGridColumnStyles
.
DataGridCheckBoxColumn
- Allow for single click to toggle CheckState
.
Using the code
You can use these controls like any other control. Download the source, compile, create a Windows application project; browse and add the component to your Toolbox. Figure 1 is the result, after the assembly is added to the Toolbox. I suggest adding it to an empty category such as 'General' or 'My User Controls' so that the controls don't get mixed up with the existing framework controls.
Figure 2 below shows how Noogen.WinForms components would look on a form. You can recognize the ReadOnlyGroup
control by the icon which looks like a square-faced guy wearing eye-glasses. ReadOnlyGroup
implements IExtenderProvider
to provide IsInGroup
property (Figure 3). ReadOnlyGroup
instance contains ReadOnly
property to allow user to dynamically change ReadOnly
states.
Figure 2 - Noogen.WinForms components on a form in design mode:

Notice in Figure 3 that Noogen.WinForms.NCheckBox
allows you to set TrueValue
, FalseValue
, and NullValue
. In this case, it is default to "Y
", "N
", and DBNull.Value
. Other common values are "0", "1", "True", "False" etc... User can set AllowNull
to false
for NCheckBox
to substitute FalseValue
for null
.
Figure 3 - Noogen.WinForms.NCheckBox
properties:

The attached demo starts by generating states, its abbreviations, and some test data (see DemoForm.GenerateData()
method). Strong-typed data binding is made possible through automatic DataSet
generation with the XSD designer.
Figure 4 - Strong-typed definition of DataSet
used in the demo:

Noogen.WinForms.DataGridComboBoxColumn
exposes both DisplayMember
and ValueMember
as seen in Figure 5. I've also set MappingName
to "state_code
" with CityStates table.
Figure 5 - What's new with the DataGridColumnStyle
collection editor?

The demo in action
Figure 6 - AutoComplete ComboBox
demo.

Figure 6 demonstrates the Noogen.WinForms.NComboBox
auto-complete function. Notice that the button has been clicked, which causes a change from "- Disable" to "+ Enable". The button allows you to toggle the ReadOnly
state of the Details
group. ReadOnly BackColor of ReadOnlyGroup
can also be configured (gray as seen in Figure 6).
private void btnEnableToggle_Click(object sender,
System.EventArgs e)
{
if (this.readOnlyGroup1.ReadOnly)
{
this.btnEnableToggle.Text = "- Disable";
this.readOnlyGroup1.ReadOnly = false;
}
else
{
this.btnEnableToggle.Text = "+ Enable";
this.readOnlyGroup1.ReadOnly = true;
}
}
Figure 7 - ContextMenu
on NDateTimePicker

Figure 7 shows how you can quickly set the value of Noogen.WinForms.NDateTimePicker
control with the help of ContextMenu
. NDateTimePicker
exposes BindableValue
property to return DBNull.Value
if current value is a MinDate
.
Figure 8 - Single click DataGridCheckBoxColumn
and CellPainting
event.

Notice that the CheckBox
on the DataGrid
is checked, but it is not so in the Details
group. The DataGrid
cell is active and so it's in edit mode. The data wouldn't change until the cell looses focus. Also notice the yellow background of HOUSTON. This is possible with the CellPainting
event explained in the code below. I have also added CellEditing
and CellCommitting
events allowing you to dynamically cancel/accept edit or commit. All three DataGridComboBoxColumn
, DataGridTextBoxColumnEx
, and DataGridCheckBoxColumn
contain all the events just mentioned.
this.dataGridTextBoxColumnEx1.CellPainting +=
new Noogen.WinForms.DataGridCellPaintEventHandler(
dataGridTextBoxColumnEx1_CellPainting);
private void dataGridTextBoxColumnEx1_CellPainting(
object sender, Noogen.WinForms.DataGridCellPaintEventArgs e)
{
if (e.CellValue.Equals("HOUSTON"))
e.BackBrush = new SolidBrush(Color.Yellow);
}
You've got to try it to really get the feel of it. If you haven't downloaded it yet, go check out the demo. Also take a look at its source to see how little code you are required to write. Almost everything is designable.
Points of interest
ReadOnlyGroup
was made possible with the use of [DllImport] SetWindowLong
and WS_DISABLED
. This may not be the best way in .NET, but it's the easiest. Another way is to use NativeWindow to subclass WndProc
and filter out all Keyboard and mouse input there. If that doesn't work, there is always the [DllImport]
of SetWindowHook
.
Known problem(s)
- Sometimes, when the UI is slow, you must keep the mouse in the area of
DataGridCheckBoxColumn
for about half a second after the first click in-order for the cell to recognize the click. Inspect the DataGridCheckBoxColumn.Edit
and IsCursorInside(bounds)
and you will see what I mean.
History
- 26th July - Version 1.0.0 uploaded.
- 28th July - Article updated.