Table of Contents
This article is the summation of my notes collected during my attempt to learn jQuery through various books and websites. As such this is still a work in progress and will be updated based on my understanding and study of this beautiful library and will add simple uses cases of jquery with asp.net/mvc.
jQuery is a javascript library with rich API to manipulate DOM, event handling, animation and ajax interactions. The following are the essential features of jQuery that makes it so appealing for client side scripting.
- jQuery is Cross-Browser
- jQuery supports Ajax
- Rich Selectors
- DOM Manipulation
- Animation
- Rich UI
The jQuery architecture revolves around the following areas.
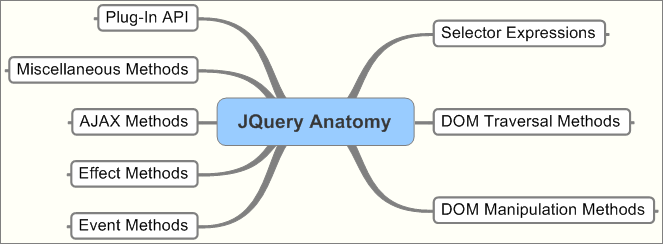
jQuery lets you run your code when the page elements have been loaded. This is more efficient than the browser onload() functions, which is called only after all images have been loaded. To run the code when the page is ready you use the following syntax
$(document).ready(function() {
....
});
There's a shorthand as well
$(function() {
....
});
Let's briefly have quick look at jQuery in action.
The $('#id') is used to select elements by id. When you click on the button "Stripe" the stripe() JS method is triggered which toggles the class of the element whose id matches 'third'. On subsequent click the style will be reset.

The code and the markup is displayed below.
<head>
<title>Select a paragraph</title>
<script type="text/javascript" src="jquery-1.3.2.min.js"></script>
<script type="text/javascript">
function stripe( )
{
$('#third').toggleClass('striped');
}
</script>
<style>
p.striped {
background-color: cyan;
}
</style>
</head>
<body>
<h1>Select a paragraph</h1>
<div>
<p>This is paragraph 1.</p>
<p>This is paragraph 2.</p>
<p id="third">This is paragraph 3.</p>
<p>This is paragraph 4.</p>
</div>
<form>
<input type = "button" value="Stripe" onclick="stripe()"> </input>
</form>
</body>
The $("div") selects all div elements. The fadeIn() effect is applied to each div matched.
The div is hidden initially.

On clicking the button the all the div elements fades in slowly.

The code and the markup is displayed below.
<head>
<title>Selecting a set of Elements</title>
<script type="text/javascript" src="Scripts/jquery-1.3.2.min.js"></script>
<script type="text/javascript">
function fade() {
$("div").fadeIn('slow');
}
</script>
</head>
<body>
<h1>Fade</h1>
<div style ="display:none">
This div was hidden.
</div>
<div style ="display:none;border:solid 1px orange">
So as this.
</div>
<form>
<input type = "button" value="Fade Div" onclick="fade()" />
</form>
</body>
The $(p.mark) selects all paragraph elements with the class "mark".

The code and the markup is displayed below.
<head>
<title>Select a paragraph</title>
<script type="text/javascript" src="Scripts/jquery-1.3.2.min.js"></script>
<script type="text/javascript">
function highlight() {
$('p.mark').toggleClass("highlight");
}
</script>
<style type="text/css">
p.mark {
font-weight: normal;
}
p.highlight {
background-color: lightgray;
}
</style>
</head>
<body>
<h1>Select a paragraph</h1>
<div>
<p class = "mark">This is paragraph 1.</p>
<p>This is paragraph 2.</p>
<p class = "mark">This is paragraph 3.</p>
<p>This is paragraph 4.</p>
</div>
<input type = "button" value="Highlight" onclick="highlight()"/>
</body>
The above discussion summed up the very basics of jQuery. The next sections dig deeper into jQuery based on the antomical structure outlined.

The CSS selectors are baed on the CSS 1-3 as outlined by the w3C. For additional information please visit w3.org
All elements selection takes the form of $('T') where 'T' stands for the element tag name.
For e.g.
- $('div') selects all elements with a tag name of div in the document
- $('input') selects all elements with a tag name of input in the document.
jQuery uses the JavaScript's getElementsByTagName() function for tag-name selectors.
This takes the form $('#id') where ID equal to id.
For e.g.
- $('#firstName') selects the unique element with id = 'firstName'.
- $('div#main') selects a single div with an id of 'main'.
If there are more than one element with the same id then jQuery selects the first matched element in the DOM.
This takes the form $('.myclass') where class equal to myclass.
For e.g.
- $('.blink') selects all elements with class = 'blink'.
- $('p.blink') selects all paragraphs that have a class of blink.
- $('.mybold.myblink') selects all elements that have a class of 'mybold' and 'myblink'.
Performance wise the example 2 is preferable as it limits the query to a given tag name.
Matches all elements matched by E2 that are descendants of an element matched by E1.
For e.g.
- $('#menu li') selects all elements matched by <li> that are descendants of an element that has an id of menu.
- $('a img') selects all elements matched by <img> that are descendants of an element matched by <a>
A descendant of an element is any element that is a child, grandchild and so on of that element.
<div id = "menu">
<div id = "item1">
<div id = "item-1.1">
<div id = "item-1.2">
<div id = "item-1.2.1"/>
</div>
</div>
</div>
In the above example "item-1.2.1" is a descendant of "item-1.2", "item-1.1", "item1" and 'menu".
Matches all elements matched by E2 that are children of an element matched by E1.
For e.g.
- $('li > ul') selects all elements matched by <ul> that are children of an element matched by <li>.
- $('p > pre') matches all elements matched by pre that are children of an element matched by<p>.
The child selector works in all modern browser except IE 6 and below. The child combinator is a more specific form of descendant
combinator as it selects only first-level descendants.
Matches all elements by E2 that immediately follow and have the same parent as an element matched by E1.
For e.g.
- $('p + img') selects all elements matched by <img> that immediately follow a sibling element matched by <p>.
The + combinator selects only siblings i.e. elements at the same level as the matched element.
Matches all elements by E2 that follow and have the same parent as an element matched by E1.
For e.g.
- $('p ~ img') selects all elements matched by <img> that follow a sibling element matched by <p>.
The difference between + and ~ is + combinator reaches only to the immediately following sibling element whereas the ~ combinator extends that reach to all following sibling elements as well.
Consider the following HTML.
<ul>
<li class = "1st"></li>
<li class = "2nd"></li>
<li class = "3rd"></li>
</ul>
<ul>
<li class = "4th"></li>
<li class = "5th"></li>
<li class = "6th"></li>
</ul>
$('li.1st ~ li) selects <li class = "2nd"> and <li class = "3rd">.
$(li.1st + li) selects <li class = "2nd">
Selects all elements matched by selector E1, E2 or E3.
For e.g.
- $('div, p, pre') selects all elements matched by <div> or <p> or <pre>.
- $('p bold, .class-name) sleects all elements matched by <bold> that are descendants of <p> as well as all elements that have a class of '.class-name'.
The comma (,) combinator is an efficient way to select disparate elements.
All elements that are the nth child of their parent.
For e.g.
- $('li:nth-child(3)') selects all elements matched by <li> that are the 3rd child of their parent.
The 'n' is 1 based as this is strictly derived from the CSS specification. For all other selectors jQuery follows 0-based counting.
All elements that are the first child of their parent.
For e.g.
- $(li:first-child') selects all elements matched by <li> that are the first child of their parent.
The :first-child pseudo-class is shorthand notation for :nth-child(1).
All elements that are the last child of their parent.
For e.g.
- $(li:last-child') selects all elements matched by <li> that are the last child of their parent.
All elements that are the only child of their parent.
For e.g.
- $(':only-child') selects all elements that are the only child of their parent.
- $('p:only-child') sleects all elements matched by <p> that are the only child of their parent.
All elements that do not match selector 's'.
For e.g.
- $('li:not (.myclass)') selects all elements matched by <li> that do not have class="myclass".
- $('li:not(:last-child)') selects all elements matched by <li> that are not the last child of their parent element.
All elements that have no children (including text nodes).
For e.g.
- $('p:empty') selects all elements matched by <p> that have no children.
- $('empty') selects all elements that have no children.
All elements
For e.g.
- $('*') selects all elements in the document.
- $(p > '*') selects all elements that are children of a paragraph element.
Contains ([E])
All elements that contain an element matched by E.
For e.g.
- $('div[p]') selects all elements matched by <div> that contain an element matched by <p>.
This selector is like the reverse of the descendant selector (either E1 // E2 or E1 E2), in that it selects all elements that have a descendant element matched by E1 instead of all elements matched by E2 that are descendants of some other element.
Attribute selectors begin with @ symbol before jQuery 1.3. After V1.3 remove the @symbol before the attribute name.
All elements that have foo attribute.
For e.g.
- $('a[rel]') selects all elements matched by <a> that have a rel attribute.
- $('div[class]') selects all elements matched by <div> that have a class attribute.
All elements that have the foo attribute with a value exactly equal to bar.
For e.g.
- $('a[rel=nofollow]') selects all elements matched by <a> that have an attribute 'rel' with a value of 'nofollow'.
- $('input[name=first-name]') selects all elements matched by <input> that have a name value exactly equals to first-name.
All elements that do not have a foo attribute with a value exactly equal to bar.
For e.g.
- $('a[rel != nofollow]') selects all elements matched by <a> that do not have an attribute 'rel' with a value of 'nofollow'.
- $('input[name != first-name]') selects all elements matched by <input> that do not have a name attribute with a value exactly equal to first-name.
NOTE: These selectors see attribute value as a single string. So, attributes with values separated by space will not be matched.
To exclude the following match <a rel="nofollow self" href=...>link</a> use the following selector
$('a:not[rel *= nofollow])').
The following is the form selector list
- :input -> selects all form elements (input, select, textarea, button).
- :text -> selects all text fields (type = "text")
- :password -> selects all password fields (type = "password")
- :radio -> selects all radio fields (type = "radio")
- :checkbox -> selects all checkbox fields (type = "checkbox")
- :submit -> selects all submit buttons (type = "submit")
- :image -> selects all form images (type = "image")
- :reset -> selects all reset buttons (type = "reset")
- :button -> selects all other buttons (type = "button")
- :file -> selects all <input type = "file">
:even All elements with an even index
:odd All elements with an odd index
For e.g.
- $('li:even') selects all elements matched by <li> that have an even index value.
- $('tr:odd') selects all elements matched by <tr> that have an odd index value.
As the :even and :odd pseudo-classes match element based on their index, they use JS's native zero-based numbering. So, even selects the first, third (and so on) elements while :odd selects teh second, fourth (and so on) elements.
The element with index value equal to n.
For e.g.
- $('li:eq(2)') selects the third <li> element
- $('p:nth(1)') selects teh second <p> element.
The elements with index greater than N.
For e.g.
- $('li:gt(1)') selects all elements matched by <li> after the second one.
The element with index value less to n.
For e.g.
- $('li:lt(3)') selects all elements matched by <li> before the 4th one, in other words the first three <li> elements.
The first instance of an element.
For e.g.
- $('li:first') selects the first <li> element.
- $('tr:first') selects the first <tr> element.
The last instance of an element.
For e.g.
- $('li:last') selects the last <li> element.
- $('tr:last') selects the last <tr> element.
All elements that are parent of another element including text node.
For e.g.
- $(':parent') selects all elements that are the parent of another element.
- $('td:parent') selects all elements matched by <td> that are the parent of another element.
All elements that contain the specified text.
For e.g.
- $('div:contains(sample div)') selects all elements matched by <div> that contain the text 'sample div'.
- $('li:contains(wrong link)') selects all elements matched by <li> that contain the text 'wrong link'.
The matching text can appear in the selector element or in any of that element's descendants.
All elements that are visible.
For e.g.
- $('div:visible') select all elements matched by <div> that are visible.
The :visible selector includes elements that have a display of block or inline or any other value other than none and a visibility of visible.
All elements that are hidden.
For e.g.
- $('div:hidden') selects all elements matched by <div> that are hidden.
jQuery has a powerful jquery traversal and manipulation methods.
We have used the $() functionto access elements in a document. Let's roll up our sleeves and try doing some trick with the $(). In fact simply inserting a snippet of HTMl code inside the parentheses, we can create an entirely new DOM structure. Isn't this amazing.
The following are the jQuery methods for inserting/appending elements into the DOM.
Inserts content specified by the parameter before each element in the set of matched elements. The return vlaue is the jQuery object for chaining purposes. Consider the following HTML fragment.
<div class = "div1">
This div already exist
</div>
Execute the following jquery script.
$('div.div1').before('<div class="insert">This div is dynamically inserted</div>')
The resulting HTML will be
<div class = "insert">
This div is dynamically inserted
</div>
<div class = "div1">
This div already exist
</div>
Inserts every element in the set of matched elements before the set of elements specified in the parameter. Consider the following HTML fragment.
<div class = "div1">
This div already exist
</div>
Execute the following jquery script.
$('<div class="insert">This div is dynamically inserted</div>').insertBefore('div.div1')
The resulting HTML will be
<div class = "insert">
This div is dynamically inserted
</div>
<div class = "div1">
This div already exist
</div>
There is only structural difference in the way both the above function works.
Inserts content specified by the parameter after each element in the set of matched element. Consider the following HTML fragment.
<div class = "div1">
This div already exist
</div>
Execute the following jquery script.
$('div.div1').after('<div class="insert">This div is dynamically inserted</div>')
The resulting HTML will be
<div class = "div1">
This div already exist
</div>
<div class = "insert">
This div is dynamically inserted
</div>
Inserts every element in the set of matched elements after the set of elements specified in the parameter. Consider the following HTML fragment.
<div class = "div1">
This div already exist
</div>
Execute the following jquery script.
$('<div class="insert">This div is dynamically inserted</div>').insertAfter('div.div1');
The resulting HTML will be
<div class = "div1">
This div already exist
</div>
<div class = "insert">
This div is dynamically inserted
</div>
Coming up soon
Coming up soon
Let's start with a small usecase in action. Let's say you have a use case to add "Comment" to a blog post. The code snippet is writting for the ASP.NET MVC framework, but it should be seamless to modify it to suit it to work with classic asp.net, php, ror or anything you deem fit.
The comment view is very simple. The following is the minimal markup without any styles for adding a comment.
<h2>Add Comment</h2>
<label for ="body">Body</label>
<br />
<%= Html.TextArea("body")%>
<div id = "loading-panel"></div>
<hr />
<input id="comment_submit" type="button" value = "Publish" />
The minimal code for the controller is shown below. When the "Publish" button is clicked we want to make a request to the PostController's action method named "NewComment" in an asynchronous fashion. The code shown is only for demonstration purpose and as such has no meaning apart from the context of this discussion.
public class PostController : BaseController
{
public ActionResult NewComment(Guid postId, string body)
{
var post = Repository.GetPostById(postId);
var comment = new Comment { Body = body, Post = post };
Repository.AddComment(comment);
return Content(body);
}
}
Let's set up the jQuery to enable posting back in an asynchronous way and also show a progress bar on the way.
<script type="text/javascript">
function flashit()
{
flash('#comment-list li:last');
}
function flash(selector)
{
$(selector).fadeOut('slow').fadeIn('show');
}
</script><script type = "text/javascript">
$(function() {
$("#comment_submit").click(function()
{
var dataString = "postId=" + "<%=Model.Post.Id %>" + "&body=" + $('#body').val(); "color: rgba(255, 0, 0, 1)"__^
type: "POST", ^__strong style="color: Red"__^(03)</strong__^
url: "/Post/NewComment", ^__strong style="color: Red"__^(04)
data: dataString, ^__strong style="color: Red"__^ (05)
beforeSend: function(XMLHttpRequest) { ^__strong style="color: Red"__^(06)
$('#loading-panel').empty().html('<img src="http://www.codeproject.com/Content/Images/ajax-loader.gif" />'); ^__strong style="color: Red"__^(07)
},
success: function(msg) { ^__strong style="color: Red"__^ (08)
$('#comment-list').append('<li>' + msg + '</li>'); ^__strong style="color: Red"__^(09)
flashit(); ^__strong style="color: Red"> (10)
},
error: function(msg) { ^__strong style="color: Red"> (11)
$('#comment-list').append(msg);
},
complete: function(XMLHttpRequest, textStatus) { ^__strong style="color: Red"> (12)
$('#loading-panel').empty(); ^__strong style="color: Red"> (13)
}
});
});
});
</script>
In the above code the jQuery factory function $() sets up the "comment_submit" click event handler. Whenever the "submit" button is clicked the click function defined above is executed. The following points highlights what's happening in the above function.
- Line 01 sets up the data to be posted to the controller.
- Line 02 invokes the ajax method with the following parameters.
- Line 03 sets the request type to "POST".
- Line 04 sets up the URL. In this case the URL is Post Controller's action method should be invoked.
- Line 05 assigns the data as part of the request.
- Line 06 sets up the beforeSend() handler. This method is executed just before the ajax method is invoked.
- Line 07 sets up the loading-panel to display a loading image. The image can be downloaded from http://www.ajaxload.info/.
- Line 08 sets up the success() handler. This method is executed if the AJAX request is sucessfully executed.
- Line 09 appends the new message to the comment-list div if the request executed successfully.
- Line 10 invokes the flashit() method which flashes/animates the inserted method.
- Line 11 sets up the error() handler. This method is executed if there are any errors while executing the AJAX request.
- Line 12 sets up the complete() handler. This method is executed after the AJAX request is completed.
- Line 13 empties the loading-panel to clear up the image.
AJAX Options
Coming up soon.
Let's quickly create an automated TOC for our page. Say we have a page with the following structure and we need to create a TOC based on the <h3> heading. We want to insert this TOC in the "header" div. All the TOC contents should link back to the content of the page.
HTML Snippet
<div id="header">
<h1>
Automatic Bookmark Headings Example</h1>
<h2>
Using jQuery to Extract Existing Page Information</h2>
</div>
<div id="Div1">
<h1>
Automatic Bookmark TOC Example</h1>
<h2>
Using jQuery to Extract Existing Page Information</h2>
</div>
<div id="Div2">
<h3>Introduction</h3>
<p>
Lorem ipsum dolor sit amet, consetetur sadipscing elitr, sed diam nonumy eirmod
tempor invidunt ut labore et dolore magna aliquyam erat, sed diam voluptua. At vero
eos et accusam et justo duo dolores et ea rebum. Stet clita kasd gubergren, no sea
takimata sanctus est Lorem ipsum dolor sit amet.
</p>
<a href="#header" title="return to the top of the page">Back to top</a>
</div>
<div id="content">
<h3>Content</h3>
<p>
Lorem ipsum dolor sit amet, consetetur sadipscing elitr, sed diam nonumy eirmod
tempor invidunt ut labore et dolore magna aliquyam erat, sed diam voluptua. At vero
eos et accusam et justo duo dolores et ea rebum. Stet clita kasd gubergren, no sea
takimata sanctus est Lorem ipsum dolor sit amet.
</p>
<a href="#header" title="return to the top of the page">Back to top</a>
</div>
The TOC Generator Script
<script type="text/javascript">
$("document").ready(function() {
createTOC('h3', 'header');
});
function createTOC(tag, container) {
var anchorCount = 0;
var oList = $("<ul id='bookmarksList'>");
$("div:not([id=header]) " + tag).each(function() {
$(this).html("<a name='bookmark" + anchorCount + "'></a>" +
$(this).html());
oList.append($("<li><a href='#bookmark" + anchorCount++ + "'>"
+ $(this).text() + "</a></li>"));
});
$("#" + container).append(oList);
}
</script>
Here the screenshot of this snippet in action

I think the code is well commented to guage the logic.
Lets quickly have a look at how you can limit the entry to textbox and display the character input left to be keyed in.
<script type="text/javascript">
$(function() {
var limit = 250;
$('#dvLimit').text('250 characters left');
$('textarea[id$=txtDemoLimit]').keyup(function() {
var len = $(this).val().length;
if (len > limit) {
this.value = this.value.substring(0, limit);
}
$("#dvLimit").text(limit - len + " characters left");
});
});
</script>
The $() function does the following things on startup.
1. Set a variable to a limit of 250 characters.
2. Assign a default value to the "div" element with id "dvLimit"
3. Find the control that contains the id "txtDemoLimit" and hook up the keyup event.
4. In the keyup event get the length of the current text.
5. If len is greater than the limit set, set the text value as a substring within the specified limit.
6. Update the div with the no. of characters left to be keyed in.
The HTML is shown below.
<div id="dvLimit"></div>
<div>
<asp:TextBox ID="txtDemoLimit" TextMode="MultiLine" Columns = "40" Rows = "10" runat="server"></asp:TextBox>
</div>
The following is the application in action.

You can create your own custom filters that an be used with jQuery selectors. This provides a good way to extend jQuery.
Let's create our own custom filter ":inline" which returns all elements whose display is set to "inline".
(function ($) {
$.expr[':'].inline = function(elem) {
return $(elem).css('display') === 'inline';
};
})(jQuery);
The above filter returns all elements which has a display style set to 'inline'. There are other ways or methods to get his information but filters seem to make the whole experience pretty seamless.
Alternate ways to create the above filter.
$.extend(
jQuery.expr.filters, {
inline: function(elem) {
return $(elem).css('display') === 'inline';
}
}
);
If you take a peek at the jQuery source code you will find that the the "jQuery.expr.filters" is just an alias for jQuery.expr[":"]. The snippet is pasted below for reference.
jQuery.expr[":"] = jQuery.expr.filters;
I first read about this at Dissecting jQuery filters
Hope you can creatively expand on the jQuery filters and share your thoughts.
Coming up soon
As you may be aware by this time that there is more than one way to work with jQuery and the thing we have to try is to use the best method i.e. the most performant method. The following are some of the ways in which you can get maximum performance out of jQuery. Note I am not taking into consideration the browser, operating system, hardware and other things which may have some consequence on performance
-
Latest Version
Get the latest version of jQuery. Since jQuery is in active development there will be plenty of fixes that will come up with each release.
-
Context
Passing a context to the jQuery function can improve the query performance as this will reduce the number of DOM elements that are traversed. In order to do this you need to pass the jQuery function a second parameter which referes to a single DOM element. This element is then used as the starting point for the DOM querying and as a result there will be some performance benefits which the app may get.
In many situation you can use the this as the second parameter. Please do note that in order for a performance gain, you need to pass an actual DOM reference as the 2nd parameter. Passing anything other than a DOM reference still requires a search of the entire document actually may hinder performance.
-
Selector Performance
This is one of the important design decision you need to take. The performance may be directly impacted by the selectors you use. The selector peformance is dependent upon the following points
- Complexity of the selector expression
- Type of selector
- Size and Complexity of the DOM that is being evaluated
Following are some of the important points you can consider while using jQuery
- Prefer ID selector whenever possible.
- The simple the selector the better the performance
- If you have to use class name, always try to use it with other selector as well.
For. e.g. Use $('div.menu') rather than $('.menu') or something similar based on your requirement. - Since Simple DOM are traversed faster the context becomes much more important.
-
Cache elements if they are used frequent
Never duplicate the DOM queries for the same element/elements. Cache the result set and use it. This is more important when you are dealing with loops. Store the wrapped set in a local variable outside the loop so that you avoid querying during each iteration.>
-
Keep DOM changes to minimum
As the previous tip this concept is important when dealing with loops. Avoid updating elements in the loop. Rather, create a string representation of the element in the loop and update the DOM outside the loop.
var list = $('div');
for (i = 1; i <= 1000; i++) {
list.append('Menu ' + i );
}
var list2 = $('div');
var items = '';
for (i = 1; i <= 100; i++) {
items += 'Menu ' + i ;
}
list2.html(items);
NOTE: string concatenation is slow but in this case it will be faster comparted to DOM manipulation. Also there are various
optimized string library that peforms efficient concatenation.
-
Use multiple selectors wherever possible
Prefer
$('#p1, #p2').hide();
Rather than
$('#p1').hide();
$('#p2').hide();
-
Leverage chaining
Use jQuery chaining when it makes sense. It increases the peforamance of the code and avoid needless repeatition.
More to come....
Modify this as per your requiement and enjoy jQuerying !!!
In this update, let's build a simple slide show app. We will keep the API simple to follow and may skip certain best practices for ease of understanding. But I will update the article with my observation of the recommended practices and may change the example to refer that.
Let's begin at the beginning. Here is the HTML markup for your reference. A live demo is available on plunkr at http://plnkr.co/edit/OCnBecXAfhXAKbQExW2v?p=info
Here is the preview of what we will be building.

I have kept the CSS simple as I don't wan't to distract this article from the main topic. Add the latest reference to jQuery and jQuery UI (We will use the jQuery UI for showing the dialog box)
<body>
<div id="board"></div>
</body>
First let's build the API for the slide show module. This will enable us to understand how to design our library.
$(function () {
mySlideShow.title("Build your own slide object V0.1",{elementId: "board", timer: 0})
.slide({
title: "Slide 1",
points: ["Point 1","Point 2", "Point 3", "Point 4","Point 5", "Point 6" ]
})
.slide({
title: "Slide 2",
points: ["Point 3","Point 2"]
})
.slide({
title: "Slide 3",
points: ["Point 3","Point 3"]
})
.slide({
title: "Slide 4",
points: ["Point 4","Point 4"]
})
.show();
});
We haven't yet coded the mySlideShow object. But let's see what we are expecting from this object. We intend to invoke the slide show, by passing in a title, the elementID were the slides will be displayed, and a timer value. If the timer vaue is 0 the slides can be navigated by clicking on the "next" and "prev" button. The timer value is in milliseconds. If you give a timer value of 1000, then after 1000 seconds the slides will automatically changed.
NOTE: This is not a full fledged libray, but has enough information to cover the fundamentals. You can adapt this as per your requirement, style it the way you want it, add images etc.
NOTE: Also, please note this code is only in couple of iterations and as such I am aware of some ways to optimize this. The article may be updated in future but the idea now is get the MVP (Minimum Viable Product) feature ready :).
Note the functions are chained here. We can specify the slides and the points in the slides by using the slide() method which takes an object literal with a title and an array of points.
Now let's see how the library/module is coded. First we wrap the entire library code in an IIFE (Immediately invoked function or also called as self invoking anonymous function). Note we are explicitly passing the global objects like window and jQuery to the function call (This is a best practice to avoid module/naming conflicts)
(function (window, $) {
var mySlideShow = {
};
window.mySlideShow = mySlideShow;
})(window, jQuery);
Here' is the full source code with detailed comment. If any part of the code is not clear please shout at me on the comments below. I will be adding more small examples like this.
(function (window, $) {
var mySlideShow = {
$element: null,
counter: 0,
slides: [],
title: function (text, options){
var elementId = options.elementId;
this.timer = options.timer;
this.$element = $("#" + elementId);
this.$element.attr("title", text);
this.$element.append("<div class='container'></div>");
this.$element.append("<div class='footer'></div>");
var $footer = $(".footer", this.$element);
$footer.append("<input type='button' class='prev' value='prev'>");
$footer.append("<input type='button' class='next' value='next'>");
$(".container").append("<div class='slides'></div>");
this.onInit();
this._next();
this._prev();
return this;
},
onInit: function () {
var that = this;
if (that.timer) {
setInterval(function(){
that.next();
},that.timer);
}
$(window).resize(function () {
$(that.$element).css({
'width': $(window).width(),
'height': $(window).height(),
'left': '0px',
'top':'0px'
});
}).resize();
},
load: function (index) {
var that = this;
var current = that.slides[index];
var $slides = $("div.slides");
$slides.html("");
$slides.append("<h2>" + current.title + "</h2>");
for(var i = 0; i < current.points.length; i++) {
$slides.hide().append("<li>" + current.points[i] + "</li>").slideDown();
}
},
next: function () {
var that = this;
that.counter ++;
if (that.counter >= that.slides.length) {
that.counter = 0;
}
that.load(that.counter);
},
prev: function () {
var that = this;
that.counter --;
if (that.counter < 0) {
that.counter = 0;
}
that.load(that.counter);
},
_next: function () {
var that = this;
$(".next").on("click", function (e) {
that.next();
})
},
_prev: function () {
var that = this;
$(".prev").on("click", function (e) {
that.prev();
})
},
slide: function (options) {
this.slides.push(options);
return this;
},
show: function () {
this.load(0);
this.$element.dialog({
height: $(window).height()-10,
width: $(window).width()-10,
modal: true
});
}
};
window.mySlideShow = mySlideShow;
})(window, jQuery);
Here's some screen shots with pointers to the code and the slide show in action.



P.S: I definitely plan on updating and adding, reviewing, correcting contents based on latest changes.
Assume you the following unordered list of data, which needs to be rendered as a treeview.
<div class="tree">
<ul>
<li><a>First Level</a>
<ul>
<li><a>Second Level</a></li>
<li><a>Second Level</a></li>
<li><a>Second Level</a></li>
</ul>
</li>
<li><a>First Level</a>
<ul>
<li><a>Second Level</a>
<ul>
<li><a>Third Level</a></li>
<li><a>Third Level</a></li>
<li><a>Third Level</a>
<ul>
<li><a>Fourth Level</a></li>
<li><a>Fourth Level</a></li>
<li><a>Fourth Level</a>
<ul>
<li><a>Fifth Level</a></li>
<li><a>Fifth Level</a></li>
<li><a>Fifth Level</a></li>
</ul>
</li>
</ul>
</li>
</ul>
</li>
<li><a>Second Level</a></li>
</ul>
</li>
<li><a>First Level</a>
<ul>
<li><a>Second Level</a></li>
<li><a>Second Level</a></li>
</ul>
</li>
</ul>
</div>
We want to invoke out custom jquery plugin as shown below.
<script>
$(function() {
$(".tree").quickTree({
onNodeClick: onclick
});
})
function onclick(el) {
console.log(el);
}
</script>
We will create a jquery plugin named "quickTree" that can be invoked on any existing
element. For this example I have made it work with a unordered list, but you can modify
the code to suite to your requirement, add images,labels etc.
The code for the jquery plugin is give below and also a working plnkr url is shared
(function( $ ) {
$.fn.quickTree = function(options) {
var settings = $.extend({
color : "#ddffdd",
}, options);
return this.each(function() {
var ele = $(this);
$(ele).find('li' ).each( function() {
if( $( this ).children( 'ul' ).length > 0 ) {
$( this ).addClass( 'parent' );
}
});
$(ele).find('li > a' ).click( function( ) {
if ($(this).parent().hasClass("parent")) {
$( this ).parent().toggleClass( 'active' );
$( this ).parent().children( 'ul' ).slideToggle( 'fast' );
}
if (settings.onNodeClick){
settings.onNodeClick(this);
}
});
});
};
}( jQuery ));
The working url is here
jQuery Treeview demo
References
- March 12, 2018- Added jquery custom treeview plugin (explanation pending)
- December 18, 2016- Added note regarding attribute selector. @symbol is no longer required after jQuery 1.3 version.
- December 16, 2016 - Added the slide show module example
- August 31, 2010 - Create your own Custom filter
- May 22, 2010 - Performance Best Practices.
- December 06, 2009 - Limit text input entry.
- September 15, 2009 - Generate Automatic TOC.
- August 24, 2009 - Added a simple AJAX use case.
- August 23, 2009 - Created the first version of the article.