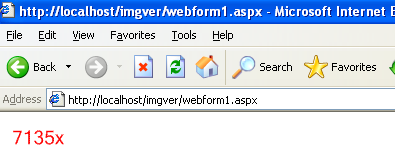
Introduction
This article will help the intermediate ASP.NET developers who may be sometimes in need of implementing a feature like Image Verification when getting inputs from the users. Basically, this is a new technique which will stop the automated programs to process the input forms. But, here I'm not explaining you about the whole technique of Image Verification. This article will explain two things, which we really need :
- How to generate alpha-numeric characters randomly?
- How to make it as an image?
Generating Random Alpha-Numerics
Microsoft .NET framework provides System.Security.Cryptography
namespace which contains RandomNumberGenerator
to produce random numbers.
The class CImgVerify
contains the code to produce the random alphabets/numbers in the function getRandomAlphaNumeric
.
RandomNumberGenerator rm;
rm = RandomNumberGenerator.Create();
The above code will create a RandomNumberGenerator
object.
byte[] data = new byte[3];
rm.GetNonZeroBytes(data);
The byte
type "data
" will hold three elements of array to have the randomly generated numbers by GetNonZeroBytes
. The GetNonZeroBytes
fills an array of bytes with a cryptographically strong random sequence of nonzero values.
The generated random numbers will be stored in a byte
variable as an array. Each and every elements will be an integer variable. So, make a proper alphanumeric string. The following code will use a loop to identify whether the randomly generated number falls into alphabets.
for(int nCnt=0;nCnt<=data.Length-1;nCnt++)
{
int nVal = Convert.ToInt32(data.GetValue(nCnt));
if(nVal > 32 && nVal < 127)
{
sTmp = Convert.ToChar(nVal).ToString();
}
else
{
sTmp = nVal.ToString();
}
sRand += sTmp.ToString();
}
Generating Image in the Sky
So, now we got the random alphanumeric to display as a image. The image can be created by the System.Drawing
namespace. The following is the code which itself self explanatory.
public Bitmap generateImage(string sTextToImg)
{
PixelFormat pxImagePattern = PixelFormat.Format32bppArgb;
Bitmap bmpImage = new Bitmap(1,1,pxImagePattern);
Font fntImageFont = new Font("Trebuchets",14);
Graphics gdImageGrp = Graphics.FromImage(bmpImage);
float iWidth = gdImageGrp.MeasureString(sTextToImg,fntImageFont).Width;
float iHeight = gdImageGrp.MeasureString(sTextToImg,fntImageFont).Height;
bmpImage = new Bitmap((int)iWidth,(int)iHeight,pxImagePattern );
gdImageGrp = Graphics.FromImage(bmpImage);
gdImageGrp.Clear(Color.White);
gdImageGrp.TextRenderingHint = TextRenderingHint.AntiAlias;
gdImageGrp.DrawString(sTextToImg,fntImageFont, new SolidBrush(Color.Red),0,0);
gdImageGrp.Flush();
return bmpImage;
}
If you have further queries/suggestions, post here.
Vadivel Kumar is from India and he works in C#/ASP.NET platforms.