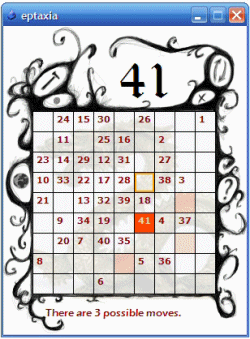
Introduction
When I was a high-school student, one of my friends showed me how to play this game. By the way, I didn't know its real name. And from a message posted to this article, I learned that its real name is "a Knights Tour". I played it all those school years, especially in boring classes. In fact, you can play it simply with paper and pencil.
While I was in the process of learning C#, I came up with the idea of creating a program of this mind game.
First of all, I should admit that I'm only a beginner in game programming in C#. So I wanted to share what I've learned on the way. I tried my best to present a clean code and clean design, and I'm eager for all kinds of feedback to improve it.
The target audience of this article is Beginner or Intermediate level programmers who wish to develop some skills in C# game programming. I do not consider myself as an expert, but a hearty programmer who wants to share what have been learned through the way.
Thanks for your interest.
Background
Eptaxia is a simple game, but winning is hard.
The rules are simple. You start from any cell of the grid, and then you make an "L" shaped move (like the horse movement in chess) from the previous one. And then it goes like this, move after move, until you fill all the cells, or you're stuck. In the figure below, you see the possible moves after the first move.

When I started to play it, it took me weeks of practice to make 49 out of a 7x7 grid. All the grid dimensions are possible to solve. The game starts as a 7x7 grid by default.
For further help, you can refer to the Help file in the package; or simply press the Help button in the game.
Using the code
In this game program, you can develop your abilities such as:
- Form handling, gathering information from another form
- Graphic abilities, drawing lines, rectangles, and images
- Double buffering in graphics
- Custom mouse event handling
- Playing sound
Also, this game program enables a clean class structure for graphical applications. Please note that, the sample code is written in Visual Studio 2005, and the demo is functional with .NET Framework 2.0.
The Class Structure
The game is based on a three-layer architecture: Windows Forms, the EptaxiaGame
class - the main engine, and the classes that serve the EptaxiaGame
class, as seen in the figure below.

EptaxiaGame
is the main engine of the game. An instance of this class (theGame
) is created in frmMain
. Event handling of frmMain is used as player-game interaction. The player uses the button clicks for making moves and to modify settings. These actions are passed to the EptaxiaGame
class.
All the drawing is done by EptaxiaGraphics
class. The graphics controller dc
is passed from the OnPaint
method of frmMain
to the ReDraw
method of EptaxiaGame
, and then to EptaxiaGraphics
.
Also, there's a subclass Grid
. This class is solely responsible for drawing the grid and the cursor. The drawing methods in ExptaxiaGraphics
are all very simple. For example, the code for drawing the Grid is as follows:
public void DrawGrid(Graphics dc)
{
Pen BlackPen = new Pen(Color.Black, lineThickness);
for (int i = 0; i < dimX + 1; i++)
{
dc.DrawLine(BlackPen, posX + gridSpace * i, posY,
posX + gridSpace * i, posY + dimY * gridSpace);
}
for (int j = 0; j < dimY + 1; j++)
{
dc.DrawLine(BlackPen, posX, posY + gridSpace * j,
posX + dimX * gridSpace, posY + gridSpace * j);
}
}
EptaxiaMoves
is the class that handles the moves made in the game. EptaxiaMessages
handles the messages given to the player, and EptaxiaSound
handles playing the sound.
Also, there are two common classes: EptaxiaLocations
provides a vector space model for locations on the grid, while EptaxiaButtons
handles the action buttons of the game (as Settings, New Game, Exit, etc.).
The Algorithm
The rule of the game is simple; you should fill all the cells of the grid. The tricky part is the rules of the "moves". You start from any cell of the grid, and then you make an "L" shaped move (like the horse movement in chess) from the previous one. And then it goes like this, move after move, until you fill all the cells, or you're stuck.
In order to understand the algorithm, you can analyze the AddNewMove
method. It directs you to the appropriate directions. There are methods that help the player, for example, the possible move locations are highlighted, but these are only cosmetics. The algorithm is really simple.
public bool AddNewMove(EptaxiaLocation ptMove)
{
if (CheckInCanvas(ptMove)
&& CheckAvailability(ptMove)
&& CheckValidity(ptMove))
{
locations[count] = ptMove;
count++;
return true;
}
else
{
return false;
}
}
For each move, when the player makes a mouse click, the click locations are converted to a vector state defined in EptaxiaLocations
. This location is checked for three conditions:
CheckInCanvas
; Is it in the grid canvas?
CheckAvailability
; Is it available? If filled before, then it's not available.
CheckValidity
; Is it really an "L" shaped legal move?
If all three conditions are met, the new "move" is added to the locations[]
array. The state of the game is controlled by the Controller
method in the EptaxiaGame
class. You can also check it for further analysis.
Points of Interest
I love programming. And after I've started game programming, I've learned that it's much harder than developing client-server programs or any business applications. This is just a simple game program, but I had hard times, especially implementing the buttons and button clicking engine. It's yet not perfect.
A special note on the screen graphics design: it's a Victorian style book ornament. I've drawn it on my Tablet PC. I know it's not perfect. Especially I'm not happy with the icons.
References
For years, CodeProject has been my reference library for my work. Countless times, I've borrowed sample codes and methods from here. So it's my time to contribute, if appropriate.
For this work also, I've used some of the CodeProject contributions. For example, for playing sounds, I've borrowed the code of MeterMan, from the article: A simple class to allow you to play Wavs in C#.
Also, for double buffering of graphics display, I've used the solution of Gil_Schmidt from his article; Don't Flicker! Double Buffer!.
Thanks for those who love to share and grow.
History & Future Development
For now, the high-score feature is not functional. The next version will cover this issue.
- 08-28-2006:
- Font problem fixed
- Also, some minor fixes on the forms
- 08-27-2006:
Conclusion
Writing the code of Eptaxia was lots of fun for me. And I'll be very glad, if you find it somehow useful for you. My main purpose was to share what I learned on the way.
I'd like to receive feedback on this work. Also, check for code update, from my site, here.
Thanks.
I was born in Kastamonu in 21 March 1971 - a small town in Black Sea region. We lived in Bursa, Zonguldak, Ankara and finally Istanbul (where I lived majority of my life).
I've completed Istanbul Technical University as an Electronics Engineer, but I've never practiced it. Now, I'm running a small software company with my brother. And also I'm continuing my PhD on Pattern Recognition at Bogazici University.
I love programming, gaming, art, painting, languages, world music, cycling.