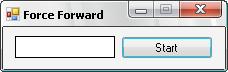
Introduction
After all those times that I have used code examples and code snippets from The Code Project, it is time to contribute something back. This article shows how easy it is to use two pre-programmed properties in the Form
class in your own project. It shows how easily the this.WindowState
and this.TopMost
can be used.
The Code
The force forward function is small and simple, it is called with a bool
value that forces the window to be on top, or resets it back to normal. If the window has been minimized to the taskbar, the window state is changed to normal so it will pop up from the taskbar.
private void ForceForward(bool forceForward)
{
if (forceForward)
{
if (this.WindowState == FormWindowState.Minimized)
this.WindowState = FormWindowState.Normal;
this.TopMost = true;
}
else
this.TopMost = false;
}
The Sequence
This program uses a timer to demonstrate. The delay is set to five seconds as the timer is started. For each time the timer “ticks”, the delay decreases by one. When the delay has reached down to zero (or somehow below), it executes ForceForward(true)
and then ends the sequence.
private void btStart_Click(object sender, EventArgs e)
{
btStart.Enabled = false;
timer.Enabled = true;
timer.Interval = 1000;
delay = 5;
lbCountdown.Text = delay.ToString();
this.Text = delay.ToString();
timer.Start();
}
private void timer_Tick(object sender, EventArgs e)
{
delay--;
lbCountdown.Text = delay.ToString();
this.Text = delay.ToString();
if (delay <= 0)
{
ForceForward(true);
EndSequence();
}
}
private void EndSequence()
{
timer.Stop();
timer.Enabled = false;
lbCountdown.Text = "";
this.Text = "Force Forward";
btStart.Enabled = true;
ForceForward(false);
}
When the end sequence has been executed, the timer is stopped and the program turns back to normal; it does not “top most” windows any more.
It all started with enthusiasm for creating mods for Quake 2 many many years ago, somehow that turned into mediocre tutorials on this website #Winning