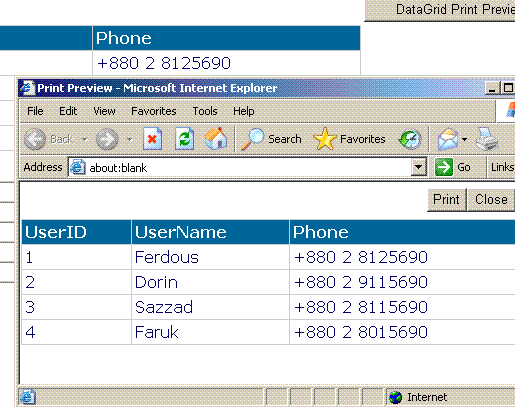

Introduction
If you want to show a print preview page before printing any page, then you have to make a page like the one that currently is showing.
Or, if you want to print a particular section of that page, like only a DataGrid
, HTML table, or any other section, and you also need to preview that in a separate page before printing, you have to create a separate print preview page to show, which is more difficult for you.
I have introduced a technique to avoid this problem. You do not need to create a separate page for print preview. You just use the JavaScript code in Script.js to create a print preview page dynamically. It will take less time to implement and so is faster. Hopefully, it will be helpful for you.
Background
I was developing a report module in my existing project. The report contents are generated dynamically by giving input (like generate by status, date range, etc). And, there is a print button to print. My client wanted to view a print preview page before printing, but we had already completed this module then. It was a really hard situation for my developers to build a print preview page for all the reports. I got this idea during that situation.
Using the code
You will just add the Script.js file in your project. The following code has been written in that file.
Source code
function getPrint(print_area)
{
var pp = window.open();
pp.document.writeln('<HTML><HEAD><title>Print Preview</title>')
pp.document.writeln('<LINK href=Styles.css type="text/css" rel="stylesheet">')
pp.document.writeln('<LINK href=PrintStyle.css ' +
'type="text/css" rel="stylesheet" media="print">')
pp.document.writeln('<base target="_self"></HEAD>')
pp.document.writeln('<body MS_POSITIONING="GridLayout" bottomMargin="0"');
pp.document.writeln(' leftMargin="0" topMargin="0" rightMargin="0">');
pp.document.writeln('<form method="post">');
pp.document.writeln('<TABLE width=100%><TR><TD></TD></TR><TR><TD align=right>');
pp.document.writeln('<INPUT ID="PRINT" type="button" value="Print" ');
pp.document.writeln('onclick="javascript:location.reload(true);window.print();">');
pp.document.writeln('<INPUT ID="CLOSE" type="button" ' +
'value="Close" onclick="window.close();">');
pp.document.writeln('</TD></TR><TR><TD></TD></TR></TABLE>');
pp.document.writeln(document.getElementById(print_area).innerHTML);
pp.document.writeln('</form></body></HTML>');
}
The getPrint(print_area)
function takes the DIV ID of the section you want to print. Then, it creates a new page object and writes the necessary HTML tags, and then adds Print and Close buttons, and finally, it writes the print_area
content and the closing tag.
Call the following from your ASPX page. Here, getPrint('print_area')
has been added for printing the print_area
DIV section. print_area
is the DIV ID of the DataGrid
and the other two will work for others DIVs. Whatever areas you want to print must be defined inside of DIV
tags. Also include the Script.js file in the ASPX page.
btnPrint.Attributes.Add("Onclick", "getPrint('print_area');")
btnTablePP.Attributes.Add("Onclick", "getPrint('Print_Table');")
btnPagepp.Attributes.Add("Onclick", "getPrint('Print_All');")
Download the source code to get the getPrint()
function.
I have used the following code in the demo project to generate a sample DataGrid
:
Private Sub PopulateDataGrid()
Dim dt As New System.Data.DataTable("table1")
dt.Columns.Add("UserID")
dt.Columns.Add("UserName")
dt.Columns.Add("Phone")
Dim dr As Data.DataRow
dr = dt.NewRow
dr("UserID") = "1"
dr("UserName") = "Ferdous"
dr("Phone") = "+880 2 8125690"
dt.Rows.Add(dr)
dr = dt.NewRow
dr("UserID") = "2"
dr("UserName") = "Dorin"
dr("Phone") = "+880 2 9115690"
dt.Rows.Add(dr)
dr = dt.NewRow
dr("UserID") = "3"
dr("UserName") = "Sazzad"
dr("Phone") = "+880 2 8115690"
dt.Rows.Add(dr)
dr = dt.NewRow
dr("UserID") = "4"
dr("UserName") = "Faruk"
dr("Phone") = "+880 2 8015690"
dt.Rows.Add(dr)
DataGrid1.DataSource = dt
DataGrid1.DataBind()
End Sub
Use the following code in a separate style sheet page. See PrintStyle.css if you want to hide the Print and Close buttons during printing.
#PRINT ,#CLOSE
{
visibility:hidden;
}
Points of interest
I was facing a problem during print. Print was not working the first time I clicked it. I solved that problem by reloading by using location.reload(true);
on that page.
Revision history
- 4-19-2006:
- Second revision. Incorporated readers comments: Fleshed out several concepts, modified a few sections (sorry BigJim61, I think I have discussed more in my article now).
- 4-17-2006:
- First revision. Added a new section.
- 4-15-2006:
Ferdous has industry level experience with SharePoint and has done several presentations and workshops on SharePoint. He also worked as SharePoint Consultant (CREDEM Italy, Robi etc) and Trainer (BASIS, JAXARA IT, LEADS Co.). He is currently working as SharePoint Architect at BrainStation-23. He also worked as Technical Project Manager for Congral LLC for managing revolutionizing Patient Centric Healthcare applications at the same company since 2009.
He worked on many international projects during his professional life. The major projects are included below:
Internal Enterprise Portal for Robi, Dhaka
nVision Solution for nSales A/S, Denmark
Shared Care Plan for Congral LLC, USA
Internet Banking Portal for the Bank of CREDEM, Italy
Document Management (DMS) for the Bank of CREDEM, Italy
MES solution for Rockwell Automation, Italy
Tourism for Travel Curve Inc., USA
and so on...
He is the author of several technical articles with over 49 articles published on http://mrbool.com where he is the Technical Author for the site and author of mssharepointtips.com as well. He is also founder of SharePoint Expert group.
Looking for a Offshore Development or partnership.............. in any development in Dot.Net & Sharepoint 2007,2010 Platform.
Search him in google by 'MJ Ferdous' to get all links, articles, profile etc
Contact him: ferdouscs@gmail.com mjferdous@live.com
Blog: http://geekswithblogs.net/ferdous
Specialties
===========
Production troubleshooting, maintainability and scalability
SharePoint 2007/2010
Dot.Net Application
Project Management
Document Management Solution