Introduction
Refactoring is the process of improving your code after it has been written by changing the internal structure of the code without changing the external behavior of the code.
Refactoring can be accessed either by going to the Refactor menu or by right clicking on the text editor. Visual Studio comes with seven refactoring options as seen below.
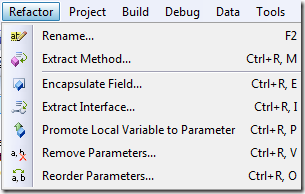
I will now show you how some of these refactorings work. I will work with this simple piece of code to illustrate simple refactorings. I will use another code sample for some refactorings. You will see it when it is required.
static void Main(string[] args)
{
string msg = "Hello";
if (msg != string.Empty)
{
Console.WriteLine(msg);
}
}
Rename:
This is perhaps simplest of all. It renames a member. This refactoring comes in very handy and is most likely one of the most used refactoring. It can rename a member within scope. Here I have few lines of code with a member declared as msg. To rename the member I just need to put my cursor on the member name and click on Refactor –> Rename menu or hit F2.

I can also include comments and/or strings in my refactor operation. Here I will ask msg
to be renamed to message
. Clicking OK on the dialog above opens another dialog where I can preview my changes.

Extract Method
Extract method extracts a method out of one or many statements. I can extract a method for the code within if statement. I am presented with a dialog which asks me the name of my new method. It is also smart enough to figure out that I need a parameter passed into this method.

Resulting code now looks like this:
class Program
{
static void Main(string[] args)
{
string msg = "Hello";
PrintToConsole(msg);
}
private static void PrintToConsole(string msg)
{
if (msg != string.Empty)
{
Console.WriteLine(msg);
}
}
}
Encapsulate Field:
From here on I will use a different code sample. This code sample is a customer object with two variables and a method.
public class Customer
{
private string name;
private string address;
public void PrintCustomerInformation()
{
string result =
string.Format("Name = {0}nAddress = {1}n", Name, Address);
}
}
This refactoring converts a private variable into a public property. By running Encapsulate Field on the private name variable presents this screen.

Once again I get a preview of changes which will be made.

I will also apply Encapsulate Field to address variable. The resulting code is modified to this.
public class Customer
{
private string name;
public string Name
{
get { return name; }
set { name = value; }
}
private string address;
public string Address
{
get { return address; }
set { address = value; }
}
public void PrintCustomerInformation()
{
string result =
string.Format("Name = {0}nAddress = {1}n", name, address);
}
}
Extract Interface:
Extract Interface as the name says will extract an interface based on the members I select. By running Extract Interface I am presented with a dialog where I can select the members I wish to include in my interface definition. For this example I will include all Address, Name, and PrintCustomerInformation members. I will also name my interface ICustomer
.

By applying this refactoring Visual Studio creates another file for ICustomer
interface. The resulting code of both files looks like this.
interface ICustomer
{
string Address { get; set; }
string Name { get; set; }
void PrintCustomerInformation();
}
public class Customer : RefactoringDemo.ICustomer
{
private string name;
public string Name
{
get { return name; }
set { name = value; }
}
private string address;
public string Address
{
get { return address; }
set { address = value; }
}
public void PrintCustomerInformation()
{
string result =
string.Format("Name = {0}nAddress = {1}n", name, address);
}
}
Conclusion
Visual Studio refactoring tools may not be at the level of Resharper or Refactor Pro but they are powerful enough for most refactoring jobs.
For further reference: http://msdn.microsoft.com/en-us/library/719exd8s.aspx
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.