Introduction
In the ASP.NET we need to access the master page controls from content page. It's true master page and content page become a single page once we run it but master page controls are not directly accessibly by content page. There are different approach to access it like using Findcontrol but here we are going to expose the gets and sets public properties of controls.
A sample project is developed in VS 2010. Master page is located under MasterPages folder and Default.aspx page under root directory of project solution. A custom CSS style is created and saved under Style directory. Project solution directory look like as shown in Fig1.
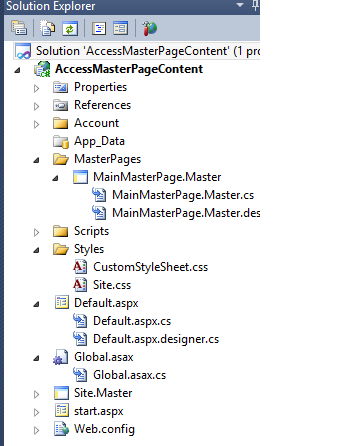
Fig1.
There is one textbox and one button in master page we would like to send data from this text box to content page by clicking the master page button. There is another button which is a content page button we would like to get the master page text box data by clicking this one also. So we can get the master page text box data to content by either click on Master page button or content page button. There is a label called lblDisplay in the content page which is used to display retrieved data.

Fig2.
Final page will look like as shown in Fig2.
Here is the code for master page.
<%@ Master Language="C#" AutoEventWireup="true" CodeBehind="MainMasterPage.master.cs" Inherits="AccessMasterPageContent.MasterPages.MainMasterPage" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<asp:ContentPlaceHolder ID="head" runat="server">
</asp:ContentPlaceHolder>
<link href="../Styles/CustomStyleSheet.css?v=c298c7f8233d" rel="stylesheet" type="text/css" />
</head>
<body>
<form id="form1" runat="server">
<div id="pageWrapper">
<div id="pageHeader">
<h3>Access Master Page Control from Content Page.</h3>
</div>
<div id="mainContent">
<asp:Label ID="lblEnterName" runat="server" Text="Enter Text: "></asp:Label>
<asp:TextBox ID="txtEnterName" runat="server"></asp:TextBox><br /> <br />
<asp:Button ID="btnMasterPageSendData" runat="server" Text=" Mater Page Button - Send Data to Content Page" /> <br />
<asp:ContentPlaceHolder ID="ContentPlaceHolder1" runat="server">
</asp:ContentPlaceHolder>
</div>
</div>
</form>
</body>
</html>
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace AccessMasterPageContent.MasterPages
{
public partial class MainMasterPage : System.Web.UI.MasterPage
{
protected void Page_Load(object sender, EventArgs e)
{
}
public TextBox EnterNameTextbox
{
get { return txtEnterName; }
set { txtEnterName = value; }
}
public Button SendDataToContentPageButton
{
get { return btnMasterPageSendData; }
set { btnMasterPageSendData = value; }
}
}
}
EnterNameTextBox
and SendDataToContentPageButton
are public proerty method of master page txyEnterName Textbox
and btnMasterPageSendData
button.
Lets create a Default.aspx page which has used master page as a template page.
<%@ Page Title="" Language="C#" MasterPageFile="~/MasterPages/MainMasterPage.Master" AutoEventWireup="true"
CodeBehind="Default.aspx.cs" Inherits="AccessMasterPageContent.Default" %>
<%@ MasterType VirtualPath="~/MasterPages/MainMasterPage.Master" %>
<asp:content contentplaceholderid="head" id="Content1" runat="server">
<asp:content contentplaceholderid="ContentPlaceHolder1" id="Content2" runat="server">
<asp:button id="btnGetDatFromMaterPage" onclick="btnGetDatFromMaterPage_Click" runat="server" text="Content Page Button -Get Dat From Mater Page">
<asp:label id="lblDispay" runat="server" text="">
We have to register the master page directive in content page to access master page controls property. So we use this
<%@ MasterType VirtualPath="~/MasterPages/MainMasterPage.Master" %>
Here is code in Default.apsx.cs file.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace AccessMasterPageContent
{
public partial class Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Page_Init(object sender, EventArgs e)
{
Master.SendDataToContentPageButton.Click += new EventHandler(btnMasterPageSendData_Click);
}
protected void btnMasterPageSendData_Click(object sender, EventArgs e)
{
lblDispay.Text = "Text " + Master.EnterNameTextbox.Text.ToString() + " send to content page form Master page.";
Master.EnterNameTextbox.Text = "";
}
protected void btnGetDatFromMaterPage_Click(object sender, EventArgs e)
{
lblDispay.Text = Master.EnterNameTextbox.Text.ToString();
Master.EnterNameTextbox.Text = "";
}
}
}
We have raised the click event of mater page button from content page from Page_Init
method. we create the instance of master page button click event before content page raises its page_load
event.
To access the master page controls we need to use the Master key word then call the Property method of control. As we use Master.EnterNameTextBox()
to access the master page EnterName
textbox and Master.SendDataToContentPageButton()
method is used to acees master page button.
There is another button which is a content page button so we don't need to keep it under page_Init
method.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.