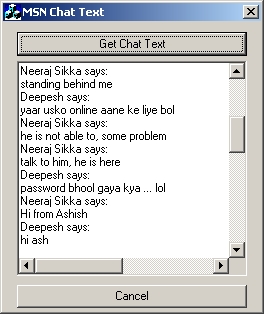
Introduction
In this article I will show you how to retrieve conversation text from MSN Messenger. In another article I have shown how the same can be done with Yahoo Messenger which can be accessed here. So without wasting any time, lets explore MSN messenger.
In MSN Messenger, the window class of the window that contains conversation text is 'RichEdit20W' for Windows NT/2K and 'RichEdit20A' for Windows 95/98. These classes support copying their content to the clipboard by sending the WM_COPY
message. Since the clipboard is available to all the applications in Windows any text copied from another application is available to all the applications in Windows. So to retrieve text from the MSN conversation window, simply copy text from the MSN Conversation window to the clipboard and paste it in your application. Simple isn't it? But sending WM_COPY
message to this window requires an HWND
of the 'RichEdit20W' window class window.
To get the HWND
, first we need to check if there is any MSN conversation windows open, this can be done by using EnumWindows()
API. If its open then get its HWND
and check its child windows to find a window having the window class 'RichEdit20W' or 'RichEdit20A', this can be done using the EnumChildWindows()
API. After getting the HWND
of conversation text window, we need to send WM_COPY
message. But sending WM_COPY
message alone will be of no use if we don't select all the text in this window. I mean simulating 'Ctrl+A'. So, in total we need to send three window messages to the conversation window. First for selecting all the text in the conversation window by sending EM_SETSEL
message with 0 for the WPARAM
and -1 for the LPARAM
; second for copying the selected text in clipboard by sending WM_COPY
message; and third for deselecting the selected text by sending EM_SETSEL
message with -1 for WPARAM
and 0 for LPARAM
value. This will be clear from the code snippet given below:
void CMSNChatTextDlg::OnGetnow()
{
if (! EnumWindows((WNDENUMPROC )EnumWindowsProc,0))
return;
}
BOOL CALLBACK CMSNChatTextDlg::EnumWindowsProc(HWND hwnd,
LPARAM lParam)
{
TCHAR buff[1000];
int buffsize=100;
HWND hMSNWnd;
hMSNWnd=NULL;
::GetWindowText(hwnd,buff,buffsize);
if (strlen(buff)<1)
return TRUE;
string strTemp(buff);
string::size_type pos=0;
pos=strTemp.rfind("- Conversation",strTemp.length());
if (pos!=-1)
EnumChildWindows(hwnd,ChildWndProc,0);
return TRUE;
}
BOOL CALLBACK CMSNChatTextDlg::ChildWndProc(HWND hwnd,
LPARAM lParam)
{
static int i=0;
LPTSTR lptstr;
HGLOBAL hglb;
char wndowclass[CLASS_SIZE];
if (GetClassName(hwnd,wndowclass,CLASS_SIZE)==0)
return TRUE;
string strTemp(wndowclass);
if ((strTemp==string("RichEdit20W")) ||<BR> (strTemp==string("RichEdit20A")))
{
::SendMessage(hwnd,EM_SETSEL,0,-1); ::SendMessage(hwnd,WM_COPY,0,0);
::SendMessage(hwnd,EM_SETSEL,-1,0);
if (!IsClipboardFormatAvailable(CF_TEXT))
return TRUE;
if (! ::OpenClipboard(NULL))
return TRUE;
hglb = GetClipboardData(CF_TEXT);
if (hglb != NULL)
{
lptstr = (LPTSTR)GlobalLock(hglb);
GlobalUnlock(hglb);
EmptyClipboard();
CloseClipboard();
pChatText->SetWindowText(lptstr);
return FALSE;
}
}
return TRUE;
}
This gives us the opportunity to make some fantastic SPYING applications that can email MSN conversations of someone to you or whatever you can think of!
In my next article, we will discuss how the same can be done with Yahoo messenger. Remember, WM_COPY
messages does not work in Yahoo. We need to put some extra efforts to accomplish this.