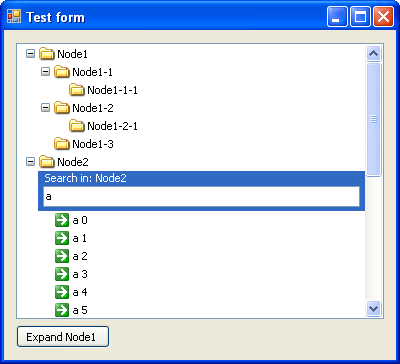
Introduction
This article illustrates a treeview control that implements node search.
Using the control
The library is very similar to the TreeView
library available in Windows Forms. It includes three classes: TreeViewEx
, TreeNodeEx
, and TreeNodeCollectionEx
, and an interface: INodeItemsFilter
. All classes behave almost the same as their Windows Forms counterparts.
Implementing a node with filtering/search
In order to implement a search mechanism for your filter node, you need to define a filter class inherited from INodeItemsFilter
. Take, for example, the following code:
using Asterix.Controls;
...
public class FindMyNodes : INodeItemsFilter {
...
TreeNodeEx[] GetItems(string query) {
ArrayList items = new ArrayList(20);
for (int i = 0; i < 20; i++) {
items.Add(new TreeNodeEx(
string.Format("{0}: {1}", query, i)));
}
return (TreeNodeEx[]) items.ToArray(typeof(TreeNodeEx));
}
}
Pass an instance of FindMyNodes
to the constructor of TreeNodeEx
, and set it as FilterNode
to one of the nodes in your collection. Take, for example, the following code:
treeViewEx1.Nodes[0].FilterNode =
new TreeNodeEx(string.Empty, new FindMyNodes());
Now, the first node in your collection expands to a search form. Type the search query and press Enter. The TreeView
will be filled with the nodes that match your query (in this case, 20 items returned by FindMyNodes
). That's it.
Points of Interest
The control fully implements visual styles with no overhead, except for the .NET framework 2.0. Still, the project could be compiled on Visual Studio .NET 2003 with little change.
The problem with visual styles, that I found pretty tricky, is that ScrollableControl
doesn't implement borders. Without borders implemented by the base class, I couldn't create borders that look like those of a TreeView
. As a workaround, I used the following piece of code:
protected override CreateParams CreateParams {
get {
CreateParams p = base.CreateParams;
p.ClassName = "SysTreeView32";
p.ClassStyle = 8;
p.ExStyle = 512;
p.Style = 1442914336;
return p;
}
}
I copied the exact CreateParams
of the standard TreeView
. This actually creates the window of a TreeView
and implements the functionality of ScrollableControl
. Now, Windows paints the borders exactly like a TreeView
and the contents are custom-drawn.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.