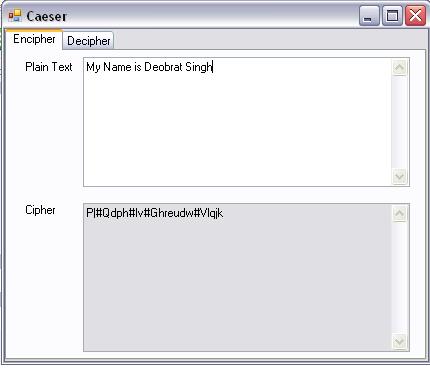
Introduction
This article describes implementing the Caesar Cipher (shift by 3) algorithm in VB.NET 2005. It’s a very simple piece of code (was difficult for me as I belong to the C# family). This was one of my practice applications to get my hands on VB.NET.
Background
Caesar Cipher is a rotation based encryption algorithm that replaces each character in the input string with the third (or nth) next character. For example, “ABC” when encrypted, will become “DEF”. Decryption is similar, simply rotate in the backward direction.
Using the code
The solution has a class CaesarCipher
which you can use in your application for encrypting and decrypting stuff. This solution applies not just to readable English characters but to any Unicode character (making it specific to English would have been a little tricky).
The source code of the CaesarCipher
class is given below:
Friend Class CaeserCipher
Private _shiftCount As Integer
Public Property ShiftCount() As Integer
Get
Return _shiftCount
End Get
Set(ByVal value As Integer)
_shiftCount = value
End Set
End Property
Public Sub New()
Me.New(3)
End Sub
Public Sub New(ByVal shiftCount As Integer)
Me.ShiftCount = shiftCount
End Sub
Public Function Encipher(ByVal plainText As String) As String
Dim cipherText As String = String.Empty
Dim cipherInChars(plainText.Length) As Char
For i As Integer = 0 To plainText.Length - 1
cipherInChars(i) = _
Convert.ToChar((Convert.ToInt32(
Convert.ToChar(plainText(i))) + Me.ShiftCount))
Next
cipherText = New String(cipherInChars)
Return cipherText
End Function
Public Function Decipher(ByVal cipherText As String) As String
Dim plainText As String = String.Empty
Dim cipherInChars(cipherText.Length) As Char
For i As Integer = 0 To cipherText.Length - 1
cipherInChars(i) = _
Convert.ToChar((Convert.ToInt32(
Convert.ToChar(cipherText(i))) - Me.ShiftCount))
Next
plainText = New String(cipherInChars)
Return plainText
End Function
End Class
Enhancements
No exception handling has been done, and the code is not unbreakable. So please modify it if you plan to use it in your application..
I am a developer (okay... that's very obvious!). I started programming at the age of 17 when I entered my engineering graduation back in the year 2001. I started off with C, C++ on UNIX. But soon I realized that if I need to make money out of my skills, I need to add some visual element to my applications (and that I need to switch to MS technologies!). Someone told me about VB. I started learning it but couldn’t get thru (I was religiously in love with curly braces; I never felt VB is a language). Then I began with VC++ 6.0 and I fell in love with it. Since then, I have been working on various MS technologies (C++, C#, VB.NET, SQL Server 2000/2005 etc.) I graduated in 2005 and currently, I am working with Microsoft in Hyderabad, India.
That’s all I can write as of now. If you want to know more, drop me a mail, I will try and respond