Introduction
Different technologies can be used for communication with a client and a server application. We can program our applications by using sockets, or we can use some helper classes from the System.Net
namespace that makes it easier to deal with protocols, IP addresses, and port numbers. .NET applications work within an application domain. An application domain can be seen as a subprocess within a process. Traditionally, processes are used as an isolation boundary. An application running in one process cannot access and destroy memory in another process. Cross-process communication is needed for applications to communicate with each other. With .NET, the application domain is the new safety boundary inside a process, because the MSIL code is type-safe and verifiable.
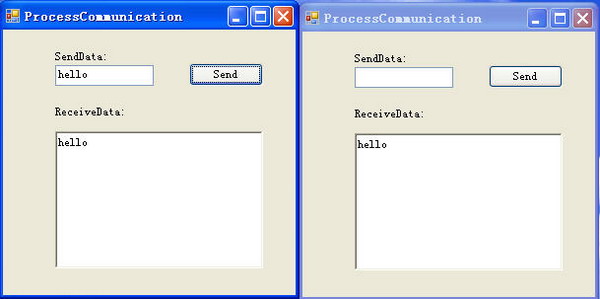
Remoting communication library
public class DataEventRepeator:MarshalByRefObject
{
public event DataReceiveHandler ReceiveData;
public void OnReceiveData(object sender, ReceiveDataEventArgs e)
{
if (this.ReceiveData!=null)
{
ReceiveData(this, e);
}
}
}
public class DataEventRepeators : List<DataEventRepeator>
{ }
DataServer communication library
public class DataServer:MarshalByRefObject ,IDataReceiver
{
public event DataReceiveHandler ReceiveData;
private static DataEventRepeators reapters;
public static DataEventRepeators Reapters
{
get
{
if (reapters==null)
{
reapters = new DataEventRepeators();
}
return DataServer.reapters;
}
}
public void SendData(string rec_data)
{
ReceiveDataEventArgs e = new ReceiveDataEventArgs();
e.Data = rec_data;
try
{
OnReceiveData(e);
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
}
private void OnReceiveData(ReceiveDataEventArgs e)
{
if (ReceiveData!=null)
{
ReceiveData(null, e);
}
}
public void AddEventRepeater(DataEventRepeator repeater)
{
Reapters.Add(repeater);
this.ReceiveData += new DataReceiveHandler(repeater.OnReceiveData);
}
}
Initial communication when first starting application via ProcessComLib.dll
public void InitCommunication()
{
BinaryServerFormatterSinkProvider provider =
new BinaryServerFormatterSinkProvider();
provider.TypeFilterLevel = TypeFilterLevel.Full;
IDictionary props = new Hashtable();
props["port"] = 0;
TcpChannel chn = new TcpChannel(props, null, provider);
ChannelServices.RegisterChannel(chn, false);
repeater.ReceiveData += new DataReceiveHandler(repeater_ReceiveData);
try
{
IDictionary prop = new Hashtable();
prop["port"] = 2007;
TcpChannel chnn = new TcpChannel(prop,null,provider);
ChannelServices.UnregisterChannel(chn);
ChannelServices.RegisterChannel(chnn, false);
RemotingConfiguration.RegisterWellKnownServiceType
(
typeof(DataComServer.DataServer),
"DataServer.rem",
WellKnownObjectMode.Singleton
);
}
catch
{
}
Connection();
}
private void Connection()
{
dataServer = (IDataReceiver)Activator.GetObject(typeof(IDataReceiver),
"tcp://localhost:2007/DataServer.rem");
dataServer.AddEventRepeater(repeater);
}
OK. That is all. More details can be found in the downloadable source code.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.