Introduction
This program will draw the Mandelbrot set in C#. It allows you to zoom in to any measure. It also supports ColorMaps to style the set. Each ColorMap file has 256 lines, one for each RGB value calculated by the Mandel set.
The Calculation
Now, let me make this perfectly clear. This article is not about the actual calculation of the Mandelbrot set. I do not claim to have come up with this code. I just found a C++ version and converted it to C# (not that hard at all). Here is the function that calculates the Mandel set:
private void DrawMandel()
{
Color[] cs = new Color[256];
cs = GetColors(ColMaps[CurColMap]);
Bitmap b = new Bitmap(this.Width,this.Height);
double x, y, x1, y1, xx, xmin, xmax, ymin, ymax = 0.0;
int looper, s, z = 0;
double intigralX, intigralY = 0.0;
xmin = Sx;
ymin = Sy;
xmax = Fx;
ymax = Fy;
intigralX = (xmax - xmin) / this.Width;
intigralY = (ymax - ymin) / this.Height;
x = xmin;
for(s = 1; s < this.Width; s++)
{
y = ymin;
for(z = 1; z < this.Height; z++)
{
x1 = 0;
y1 = 0;
looper = 0;
while(looper < 100 && Math.Sqrt((x1 * x1) + (y1 * y1)) < 2)
{
looper++;
xx = (x1 * x1) - (y1 * y1) + x;
y1 = 2 * x1 * y1 + y;
x1 = xx;
}
double perc = looper / (100.0);
int val = ((int)(perc * 255));
b.SetPixel(s,z,cs[val]);
y += intigralY;
}
x += intigralX;
}
bq = b;
this.BackgroundImage = (Image)bq;
}
I would recommend that you run this method on a different thread so it doesn't tie up your app.
Zooming
The zooming concept was a hard one to get nailed down. I knew what had to be done, but it took me a while to figure out how to do it. What you have to do is change Sx
, Sy
, Fx
, and Fy
. If you change where the method starts and finishes, the function will decrease how much the value changes each time. I did this by getting the percent of where you clicked and multiplying it by the x and y integrals.
One of the only problems with zooming was keeping an aspect ratio. I solved this problem by setting a point where the mouse is first clicked and, on MouseMove and MouseUp, calculating the height of the rectangle using the width of the rectangle. This seems to work OK for me. It is a bit slow when making a large selection, but it works.
Side notes
My mouse has 3 buttons. I used the left one to zoom, the right one to reset back to normal values, and the middle one to change the color maps. The only problem is that there are more than 3 things you can do in this app. For example, you can also save settings and open old settings. Thus, I also had to incorporate keyboard commands.
Yes, I know my code isn't commented very well, but if you do not understand a piece of it, just post the question in the forum below.
One last note, you'll notice that there are no pictures with this article. You want to know why? Because the possibilities are endless with the Mandelbrot set. I couldn't show you just 1 picture and say, "That is the Mandelbrot set." because there is so much more to it. I will try to post some pictures I have gotten from this app somewhere on the web and put the address here.
On second thought, here is one picture of the Mandelbrot. This is with no zoom or anything.
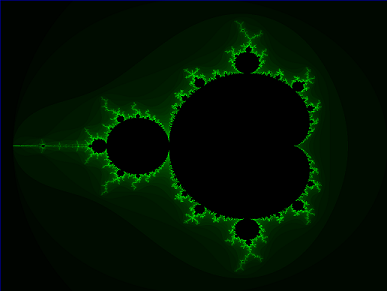
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.