Introduction
Classical Rule&Run software become more and more boring since they make users do the same tasks again and again without any response. Although they are able to, most software do not take care of what you are trying to do, or what you are exhausted to do. Another issue is that, due to users with differing habits and due to developers who believe 'more is better', software are providing more than demanded. As this is the case, for most application software, among the numerous properties and use cases they have, only a few of them are preferred by the user and the others remain unemployed. Therefore, a software forms a narrow but dull usage pattern. As you can easily observe in technology (Application software, TVs, mobile phones, PDAs, kiosks, industrial systems etc.), assisting and self-customizing systems are today's most demanded types while users are beginning to complain about the static systems. As seen in MS Office 2000 intelligent menu or intelligent font selection combobox cases, even a small piece of intelligence makes a lot of people happy.
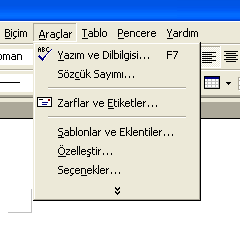
I call the assisting, self-customizing and aware applications by a common name, Intelligent Systems. Apart from artificial intelligence or learning systems, Intelligent Systems (going to be 'IS' throughout the article) has 'initially-set' decisions and variables appropriately chosen for the use case.
In this series of articles, I will present some IS applications that you can easily use in your application to make it smarter. Also, I will try to mention the underlying thoughts and mechanism of IS. For all the work, I have used my favorite pair; C# and Visual Studio .NET 2003.
Background
Although not a prerequisite for using the code, I want to present the idea for IS. The ones who are impatient to use IS in their applications can skip this part while recommended for the 'thinking developers'.
- Humans observe. We have five senses to make observations. They are the five senses which make us aware of things going around.
- Humans remember. The observation can stop and continue at any time and the they can be appended by any means.
- Humans are intelligent. Processing of gathered observations is performed by large number of logical gates fed by present and past observations.
To end up with a result like "it's cold these days", we have to observe the cold weather some adjacent days, remember the previous observations, append them, and end up with a conclusion (think about this!). In the same manner;
- Applications have events. They are the events (poor, they have only one sense) which make them aware of things going around.
- Applications have memories. Present information can be permanent in terms of files, and future information can be appended to it.
- Applications are dummy. But we can educate them! This is the main idea of IS.
Step by Step IS
A - Counting
To make observations on a running application, you have events to use. How many times an event is fired, when is it mostly fired, what are the other conditions when the event is fired, which events are fired sequentially, which events are followed by an error... These questions are numerous. The answers are obtained by counting. You have to develop a counting mechanism which should be easily mounted to the event handlers. If you are going to learn the frequency of clicking a button, you have to set an integer flag, increment it by one also with a time-stamp at each click, and divide the number of clicks by the observation time.
B - Storing
Observations (counts) you have made so far are valuable and must be permanent. (After some time) At each run of your application, user should not start from the beginning but should feel more familiar to the application. So, you have to store the counting data. For storing, you should use a simple, fast-access and standard method. This obviously points at XML.
C - Processing
Now, you have a live counting mechanism and stored information about the use of your application. Processing is the next and most crucial part for the IS applications. You, as a developer, have to determine the UI strategies. What can I do for the most frequent use-case to make it more friendly, or least frequent use-case to remove it from sight? How should I offer the user an alternative way (or a shortcut) if it is not used (which means that the user is not aware of it)? How should I remind the user that he didn't perform a task today that he used to do everyday? After determining the strategies, you should chose the appropriate processing (grading) mechanism. You have to end up with precise meanings for the grading you have: what means 'not used', what means 'frequently used', what means 'he forgot' etc. A distribution of events on time will be helpful to achieve this. The distribution can be a Gaussian (Normal) distribution, Rayleigh distribution etc. (This approach will be exampled in the next part of my article.) Good news for ones who hate mathematics; you can also use predefined grade levels and use if-else statements. Like 'if the button1Click event is fired more than 500 times a day, button1 is frequently-used, assign button1 as accept button' or 'when the student is not selected on the listview for ten days , hide him'...
Using the code
The sample code shows the top-down implementation of an intelligent combobox sorting its items according to their selection hits. Depending on properties of your observation fields, you can build your own class for your information object. I have built a class called GradeInfo
which has the following structure, and remaining of my project is dependent on GradeInfo
class. I could not find a shorter and more suitable name for 'the object whose event frequency is to be counted', and I will call it 'item' throughout the code and article. I hope it won't be confusing.
using System;
namespace Codelaboratory.IntelligentSystems
{
public class GradeInfo
{
private string itemName;
private bool recentlyUsed;
private DateTime lastHitDate;
private int hits;
private int gradePoint;
public GradeInfo()
{
}
.
.
.
}
}
I think everything is obvious in the GradeInfo
class. Most are helpful fields for my processing; nothing is a must, except the itemName
. itemName
is an identifier for what is counted. (For the previous buttonClick
example, itemName
is the button name, "button1").
My items are companies in this case. To start grading of items, you have to supply the ItemGrader
class, the item collection you want to grade; and the identifying member, name of the property of the object to use as itemName
.
private void ISExample_Load(object sender, System.EventArgs e)
{
this.cmbIntelligent.DataSource = this.Companies;
this.cmbIntelligent.DisplayMember = "Name";
ItemGrader itemGrader = new ItemGrader();
itemGrader.Items = this.Companies;
itemGrader.IdentifyingMember = "Name";
itemGrader.InitializeGrading();
}
The ItemGrader
class encapsulates the necessary methods and properties to form GradeInfo
objects and make the counting process ready for storing. Once you make the above initialization, you have to decide where to hit the count! The most important method of ItemGrader
object is the Hit
method. This method is static
and increments the indicated item's hit by one when called.
private void cmbItems_SelectedIndexChanged(object sender, System.EventArgs e)
{
ItemGrader.Hit(((Company) this.cmbItems.SelectedItem)).Name);
.
.
.
}
As the hits for the items are counted and stored in the XML file, your valuable information is maturing. You can already use the information through GradeInfo
object of an item. To achieve this, you have to call the GetGrade(string itemName)
method by passing the itemName
as the input parameter.
GradeInfo gradeInfo = ItemGrader.GetGrade("myCompany.Name");
Or, in order to get the grade point;
int point = ItemGrader.GetGradePoint("myCompany.Name");
GradeComputer
class is reserved for main processing algorithms. For now, class contains direct ordering mechanism which is provided by ReorderCollection (ArrayList items)
method. This method puts the items in the order of frequency of use and returns the resulting collection for use. Like:
private void btnSymbolic_Click(object sender, System.EventArgs e)
{
ArrayList list = GradeComputer.ReorderCollection(this.Companies ,"Name");
this.cmbIntelligent.DataSource = list;
this.cmbIntelligent.DisplayMember = "Name";
}
Conclusion
As you see in the demo project, companies are reordered at runtime in the descending order of selection numbers. This combobox is only a practical example for IS and it will be released as a single user control here at CodeProject soon.
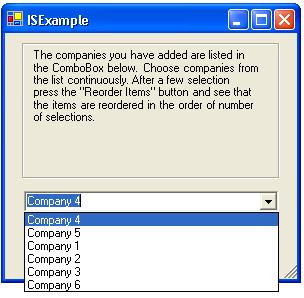
Dear CodeProject website builders; you can use my code for the forum subjects' combobox at the main page (Got programming questions?), since I visit only two or three forums at Code Project but I always go some depth in the combobox to select them. I already have a cookie to log in.
In Part II ...
- New aspects of IS reduced to "I only believe what I see" developers.
- More approximate user analysis and more surprised users.
- Embedded mathematical distributions in a separate assembly, away from developers.
- More handy and more overloaded methods in
GradeComputer
class for processing results.
- A drag and use intelligent control.
Utku has better things than developing software but it can also make a day. He likes C# much.
Utku likes elegance and sincerity.