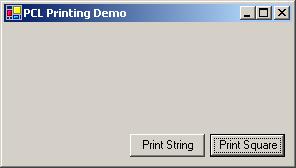
Introduction
With this code, you can print directly to the printer using WIN32 API calls and therefore should enable you to print at maximum speed. Additionally, there is code to show how to send Hewlett Packard PCL5 codes to the printer.
Using the Code
- Add a form to your project.
- Add a button to the form.
- Change the
Name
property of the button to btnString
. - Add another button to the form.
- Change the
Name
property of the button to btnSquare
. - Write the following code for the buttons:
private void btnString_Click(object sender, System.EventArgs e)
{
string st1 = "Printing a string test.";
Printing.DirectPrinter.SendToPrinter ("Printing a string",st1,
"\\\\192.168.1.101\\hpl");
}
private void btnSquare_Click(object sender, System.EventArgs e)
{
string st1="*p900x600Y";
st1+="*c600a6b0P";
st1+="*c6a600b0P";
st1+="*p900x1200Y";
st1+="*c606a6b0P";
st1+="*p1500x600Y";
st1+="*c6a600b0P";
st1+="\f";
Printing.DirectPrinter.SendToPrinter ("Printing a square",st1,
"\\\\192.168.1.101\\hpl");
}
Now:
- Add a new class to the project called DirectPrinter.cs.
using System.Text;
using System.Runtime.InteropServices;
[StructLayout( LayoutKind.Sequential)]
public struct DOCINFO
{
[MarshalAs(UnmanagedType.LPWStr)] public stringpDocName;
[MarshalAs(UnmanagedType.LPWStr)] public stringpOutputFile;
[MarshalAs(UnmanagedType.LPWStr)] public string pDataType;
}
namespace Printing
{
public class DirectPrinter
{
public DirectPrinter()
{
}
[ DllImport( "winspool.drv",CharSet=CharSet.Unicode,ExactSpelling=false,
CallingConvention=CallingConvention.StdCall )]
public static extern long OpenPrinter(string pPrinterName,
ref IntPtr phPrinter, int pDefault);
[ DllImport( "winspool.drv",CharSet=CharSet.Unicode,ExactSpelling=false,
CallingConvention=CallingConvention.StdCall )]
public static extern long StartDocPrinter(IntPtr hPrinter,
int Level, ref DOCINFO pDocInfo);
[ DllImport( "winspool.drv",CharSet=CharSet.Unicode,ExactSpelling=true,
CallingConvention=CallingConvention.StdCall)]
public static extern long StartPagePrinter(IntPtr hPrinter);
[ DllImport( "winspool.drv",CharSet=CharSet.Ansi,ExactSpelling=true,
CallingConvention=CallingConvention.StdCall)]
public static extern long WritePrinter(IntPtr hPrinter,string data,
int buf,ref int pcWritten);
[ DllImport( "winspool.drv" ,CharSet=CharSet.Unicode,ExactSpelling=true,
CallingConvention=CallingConvention.StdCall)]
public static extern long EndPagePrinter(IntPtr hPrinter);
[ DllImport( "winspool.drv" ,CharSet=CharSet.Unicode,ExactSpelling=true,
CallingConvention=CallingConvention.StdCall)]
public static extern long EndDocPrinter(IntPtr hPrinter);
[ DllImport( "winspool.drv",CharSet=CharSet.Unicode,ExactSpelling=true,
CallingConvention=CallingConvention.StdCall )]
public static extern long ClosePrinter(IntPtr hPrinter);
public static void SendToPrinter(string jobName,
string PCL5Commands, string printerName)
{
System.IntPtr lhPrinter=new System.IntPtr();
DOCINFO di = new DOCINFO();
int pcWritten=0;
di.pDocName=jobName;
di.pDataType="RAW";
OpenPrinter(printerName,ref lhPrinter,0);
StartDocPrinter(lhPrinter,1,ref di);
StartPagePrinter(lhPrinter);
WritePrinter(lhPrinter,PCL5Commands,PCL5Commands.Length,ref pcWritten);
EndPagePrinter(lhPrinter);
EndDocPrinter(lhPrinter);
ClosePrinter(lhPrinter);
}
}
}
If you have any questions, please leave a comment below.
History
- Version 1.0 - Initial release
License
This article has no explicit license attached to it, but may contain usage terms in the article text or the download files themselves. If in doubt, please contact the author via the discussion board below. A list of licenses authors might use can be found here.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.