Introduction
This tool was created to search Active Directory for user's information. But it is not complete yet. It will be very useful to get any comments on this theme.
Here is the screenshot and part of the code (see file ActiveDirectorySearcher.cs in the source code) with all the application functionality.
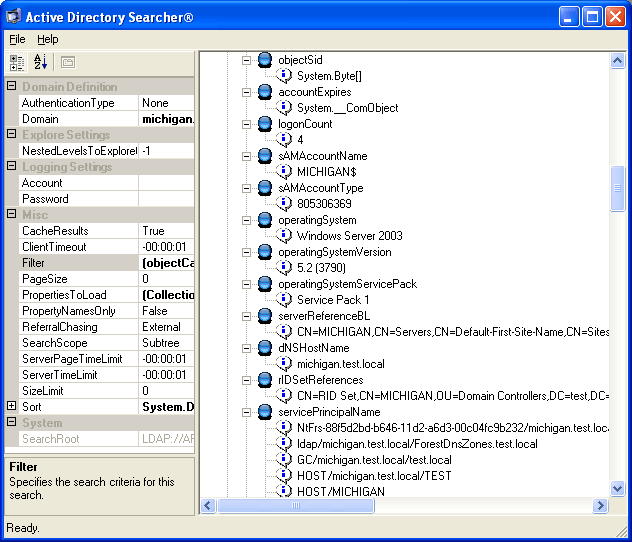
using System;
using System.DirectoryServices;
using System.Windows.Forms;
using System.ComponentModel;
using System.Collections;
namespace Ads
{
[DefaultProperty("Domain")]
public class ActiveDirectorySearcher : DirectorySearcher
{
private string strDomain;
private string strAccount;
private string strPassword;
private AuthenticationTypes atAuthType;
private int intNestedLevelCount;
public ActiveDirectorySearcher()
{
base.Filter = "(objectCategory=computer)";
intNestedLevelCount = -1;
}
[Description("Path to search")]
[Category("System")]
[ReadOnly(true)]
public new DirectoryEntry SearchRoot
{
get { return new DirectoryEntry(String.Format("LDAP://{0}",
strDomain), String.Format("{0}/{1}", strDomain, strAccount),
strPassword, atAuthType); }
}
private void SetSearchRoot()
{
if((strAccount != null)&&(strAccount.Trim() != String.Empty))
base.SearchRoot = new DirectoryEntry(String.Format("LDAP://{0}",
strDomain), String.Format("{0}/{1}",
strDomain, strAccount), strPassword, atAuthType);
else
base.SearchRoot = new DirectoryEntry(String.Format("LDAP://{0}",
strDomain));
}
[Description("Domain to search")]
[Category("Domain Definition")]
public string Domain
{
get { return strDomain; }
set
{
strDomain = value;
SetSearchRoot();
}
}
[DefaultValue(AuthenticationTypes.None)]
[Description("Authentication type")]
[Category("Domain Definition")]
public AuthenticationTypes AuthenticationType
{
get { return atAuthType; }
set
{
atAuthType = value;
SetSearchRoot();
}
}
[Description("Account in domain to login to")]
[Category("Logging Settings")]
public string Account
{
get { return strAccount; }
set
{
strAccount = value;
SetSearchRoot();
}
}
[DefaultValue("")]
[Description("Password to login to account in domain")]
[Category("Logging Settings")]
public string Password
{
get { return strPassword; }
set
{
strPassword = value;
SetSearchRoot();
}
}
[DefaultValue(-1)]
[Description("Nested levels to explore quantity. " +
"Set it to -1 to explore all available.")]
[Category("Explore Settings")]
public int NestedLevelsToExploreQuantity
{
get { return intNestedLevelCount; }
set { intNestedLevelCount = value; }
}
private TreeNode GetChildrenOfDirectoryEntry(DirectoryEntry cde,
int currentLevel)
{
try
{
if((intNestedLevelCount > 0)&&(currentLevel >= intNestedLevelCount))
return new TreeNode("Children: max. nested level reached", 7, 8);
TreeNode tnChilds = new TreeNode("Children", 6, 8);
foreach(DirectoryEntry de in cde.Children)
{
tnChilds.Nodes.Add(GetNodeFromDirectoryEntry(de, currentLevel+1));
}
return tnChilds;
}
catch(Exception x)
{
return new TreeNode("<ERROR> "+x.Message, 7, 7);
}
}
private TreeNode GetDescriptionOfDirectoryEntry(DirectoryEntry de)
{
try
{
TreeNode tnDescription = new TreeNode("Description", 9, 11);
if(de.NativeGuid != null)
tnDescription.Nodes.Add(new TreeNode("Native GUID: "+
de.NativeGuid, 12, 14));
else
tnDescription.Nodes.Add(new
TreeNode("Native GUID: <NULL>", 13, 14));
tnDescription.Nodes.Add(new TreeNode("GUID: "+
de.Guid.ToString(), 12, 14));
tnDescription.Nodes.Add(new TreeNode("Authentication Type: "
+de.AuthenticationType.ToString(), 12, 14));
if(de.Password != null) tnDescription.Nodes.Add(new
TreeNode("Password: "+de.Password, 12, 14));
else
tnDescription.Nodes.Add(new
TreeNode("Password: <UNAVAILABLE>", 13, 14));
if(de.Path != null)
tnDescription.Nodes.Add(new TreeNode("Path: "
+de.Path, 12, 14));
else
tnDescription.Nodes.Add(new
TreeNode("Path: <NULL>", 13, 14));
if(de.SchemaClassName != null)
tnDescription.Nodes.Add(new TreeNode("Schema Class Name: "
+de.SchemaClassName, 12, 14));
else
tnDescription.Nodes.Add(new
TreeNode("Schema Class Name: <NULL>", 13, 14));
if(de.Username != null)
tnDescription.Nodes.Add(new TreeNode("User Name: "+
de.Username, 12, 14));
else
tnDescription.Nodes.Add(new
TreeNode("User Name: <NULL>", 13, 14));
if(de.Site != null)
tnDescription.Nodes.Add(new TreeNode("Site: " +
de.Site.Name, 12, 14));
else
tnDescription.Nodes.Add(new
TreeNode("Site: <NULL>", 13, 14));
return tnDescription;
}
catch(Exception x)
{
return new TreeNode("<ERROR> "+x.Message, 13, 13);
}
}
private TreeNode GetPropertiesFromDirectoryEntry(DirectoryEntry de)
{
try
{
TreeNode tnProperties = new TreeNode("Properties", 3, 5);
foreach(string strPropertyName in de.Properties.PropertyNames)
{
TreeNode tnCurrentProperty;
try
{
tnCurrentProperty = new TreeNode(strPropertyName, 15, 17);
foreach(object objValue in de.Properties[strPropertyName])
tnCurrentProperty.Nodes.Add(new
TreeNode(objValue.ToString(), 12, 14));
}
catch(Exception x)
{
tnCurrentProperty = new TreeNode(strPropertyName+
": <ERROR>: "+x.Message, 16, 16);
}
tnProperties.Nodes.Add(tnCurrentProperty);
}
return tnProperties;
}
catch(Exception x)
{
return new TreeNode("<ERROR> "+x.Message, 4, 4);
}
}
private TreeNode GetNodeFromDirectoryEntry(DirectoryEntry de, int currentLevel)
{
try
{
string strName = (de.Name == null) ? "<UNNAMED>" : de.Name;
RaizeSearchInfoEvent("Creating "+strName+" entry...");
TreeNode tnResult = new TreeNode(strName, 0, 2);
tnResult.Nodes.Add(GetDescriptionOfDirectoryEntry(de));
tnResult.Nodes.Add(GetPropertiesFromDirectoryEntry(de));
tnResult.Nodes.Add(GetChildrenOfDirectoryEntry(de, currentLevel));
RaizeSearchInfoEvent(strName+" added.");
return tnResult;
}
catch(Exception x)
{
return new TreeNode("<ERROR> "+x.Message, 1, 1);
}
}
public TreeNode[] Search()
{
RaizeSearchInfoEvent("Initializing...");
ArrayList alResults = new ArrayList();
SetSearchRoot();
RaizeSearchInfoEvent("Starting search...");
SearchResultCollection src = FindAll();
RaizeSearchInfoEvent("Creating search results...");
foreach(SearchResult sr in src)
alResults.Add(GetNodeFromDirectoryEntry(
sr.GetDirectoryEntry(), 0));
RaizeSearchInfoEvent("Search complete.");
return (TreeNode[])(alResults.ToArray(typeof(TreeNode)));
}
public delegate void SearchInfoEventHandler(string info);
public event SearchInfoEventHandler SearchInfo;
private void RaizeSearchInfoEvent(string info)
{
if(SearchInfo != null) SearchInfo(info);
}
}
}
History
- 17th June, 2005: Initial post
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.