Introduction
This article depicts how to refresh a portion of a parent ASP.NET page while closing a child/pop-up window. This won't affect any sessions, cookies, and other public/static variables in the parent page.
Using the code
This sample uses two ASPX pages: Parent.aspx and popup.aspx. Place whatever code you want in the Parent.aspx page with one DataGrid
bound with a DataTable
. Pass a DataTable
as a Session variable to the popup window. Do something in the popup window, e.g., add or remove some data from the DataTable
.
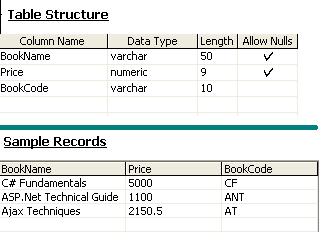
Parent.aspx
Place two similar DataGrid
s in this page, bind same data with both the grids, and place a hidden button (the Click
event of this button is called while closing the popup window). Add a Show button to show the popup window.
<%@ Page Language="C#" AutoEventWireup="true"
CodeFile="Default.aspx.cs" Inherits="Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>Parent-Child</title>
</head>
<body>
<form id="frmParent" runat="server">
<table id="tableGrid2" cellSpacing="0" cellPadding="0"
width="100%" align="center" border="0">
<tr>
<td>
<asp:DataGrid id="GridOne" runat="server"
Font-Names="Tahoma" Font-Size="8pt"
HorizontalAlign="Center" Width="100%"
GridLines="Both" AllowSorting="True"
AutoGenerateColumns="False" AllowPaging="True"
CellPadding="1" BackColor="Beige">
<HeaderStyle Font-Size="8pt" Font-Names="Tahoma"
Font-Bold="True" Height="20px"
ForeColor="White" BackColor="#669999"
HorizontalAlign="Left"></HeaderStyle>
<Columns>
<asp:BoundColumn DataField="MagazineName"
HeaderText="Magazine Name"/>
<asp:BoundColumn DataField="Price" HeaderText="Price"/>
</Columns>
<PagerStyle HorizontalAlign="Right" BackColor="#669999"
Mode="NumericPages" ForeColor="White" />
</asp:DataGrid>
</td>
</tr>
</table>
<table id="tableGrid2" cellSpacing="0" cellPadding="0"
width="100%" align="center" border="0">
<tr>
<td>
<asp:DataGrid id="GridTwo" runat="server"
Font-Names="Tahoma" Font-Size="8pt"
HorizontalAlign="Center" Width="100%"
GridLines="Both" AllowSorting="True"
AutoGenerateColumns="False"
AllowPaging="True" CellPadding="1" BackColor="Beige">
<HeaderStyle Font-Size="8pt" Font-Names="Tahoma"
Font-Bold="True" Height="20px" ForeColor="White"
BackColor="#669999" HorizontalAlign="Left"></HeaderStyle>
<Columns>
<asp:BoundColumn DataField="MagazineName" HeaderText="Magazine Name"/>
<asp:BoundColumn DataField="Price" HeaderText="Price"/>
</Columns>
<PagerStyle HorizontalAlign="Right" BackColor="#669999"
Mode="NumericPages" ForeColor="White" />
</asp:DataGrid>
</td>
</tr>
</table>
<asp:Button ID="btnHidden" runat="Server"
style="display:none" OnClick="btnHidden_Click" />
<asp:Button ID="btnShowPopup" runat="Server"
Text="Open Window" OnClick="btnShowPopup_Click" />
</form>
</body>
</html>
Parent.aspx.cs
Create a DataTable
(here, I query a SQL table Books having columns BookName
, Price
, and BookCode
. Create functions to bind the grids. Call the function BindGridTwo()
to bind GridTwo
on the hidden button's Click
event (the event will be fired from the pop-up window's JavaScript).
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
public partial class Parent : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridOne();
BindGridTwo();
}
}
private DataTable CreateDataTable()
{
DataSet bookSet = new DataSet();
string Stmt = "Select BookName,Price,BookCode from Books";
SqlConnection Conn = new SqlConnection(
ConfigurationManager.ConnectionStrings["TmpConString"].ConnectionString);
Conn.Open();
SqlDataAdapter adapter = new SqlDataAdapter(Stmt, Conn);
adapter.Fill(bookSet);
Conn.Close();
return bookSet.Tables[0];
}
private void BindGridOne()
{
DataTable bookTable_one = CreateDataTable();
GridOne.DataSource = bookTable_one;
GridOne.DataBind();
}
private void BindGridTwo()
{
DataTable bookTable_one = CreateDataTable();
GridTwo.DataSource = bookTable_one;
GridTwo.DataBind();
}
protected void btnHidden_Click(object sender, EventArgs e)
{
BindGridTwo();
}
protected void btnShowPopup_Click(object sender, EventArgs e)
{
string popupScript = "<script language="'javascript'">" +
"window.open('popup.aspx', 'ThisPopUp', " +
"'left = 300, top=150, width=400, height=300, " +
"menubar=no, scrollbars=no, resizable=no')" +
"</script>";
Page.ClientScript.RegisterStartupScript(GetType(), "PopupScript", popupScript);
}
}
popup.aspx
Just add two buttons in this page; first to update the table, and second to close the window as well as calls the parent page's btnHidden_Click
event (this is defined in the client-side script RefreshParent
).
<%@ Page Language="C#" AutoEventWireup="true"
CodeFile="popup.aspx.cs" Inherits="popup" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Popup window</title>
<script language="Javascript">
function RefreshParent()
{
window.opener.document.getElementById('btnHidden').click();
window.close();
}
</script>
</head>
<body>
<form id="frmpopup" runat="server">
<asp:Button ID="btnUpdate" runat="Server"
Text="Update Record" OnClick="btnUpdate_Click" />
<asp:Button ID="btnClose" runat="Server"
Text="Close Window" OnClientClick="RefreshParent();" />
<asp:Label ID="lblMsg" runat="Server" />
</form>
</body>
</html>
popup.aspx.cs
Do something with the Books table. Here, I'm updating a record.
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
public partial class popup : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnUpdate_Click(object sender, EventArgs e)
{
string Stmt = "Update Books Set Price = 1500 Where BookCode = 'CF'";
SqlConnection Conn = new SqlConnection(
ConfigurationManager.ConnectionStrings["TmpConString"].ConnectionString);
SqlCommand cmd = new SqlCommand(Stmt, Conn);
Conn.Open();
cmd.ExecuteNonQuery();
Conn.Close();
lblMsg.Text = "Record updated";
}
}
web.config
You have to define the connection string in the web.config file as shown below:
<connectionStrings>
<add name="TmpConString"
connectionString="Data Source=localhost;
Initial Catalog=Shopping;User ID=xxxxx;Password=yyyyy"
providerName="System.Data.SqlClient"/>
</connectionStrings>
Conclusion
You can notice that the second grid's record against your update query will be updated.
Solution Architect | Technical Lead | Team Lead | Expertise in Microsoft Technologies |