Introduction
IKVM.NET is an open source implementation or JVM for Microsoft .NET Framework and Mono. Suppose you have developed some library in java and you want to use in your .NET Projects, then IKVM.NET comes in role and helps to use java libraries in .NET.
Now a days Java and .NET are two major technologies for software development and java is older technology and there were already lots of work has done. So when it comes to reusability then it is very common practice to use library created in java, require in .NET.
Details
IKVM.NET includes following three main components:
1. A Java Virtual Machine implemented in .NET :
It has a JVM which is developed using C#.NET, which provides facilities like byte-code translation and verification, class loading, etc.
2. A .NET implementation of the Java class libraries :
It basically uses OpenJDK project for implementations of the JDK libraries.
3. Tools that enable Java and .NET interoperability :
IKVM.NET includes the following tools:
a) ikvm - Java Virtual Machine
b) ikvmc - Compiles Java Bytecode to CIL
c) ikvmstub - Generates Java stub classes from .NET assemblies
a) IKVM.NET Virtual Machine (ikvm.exe):
This is Starter executable. We can compare it with java.exe ("dynamic mode") .It loads class file and execute its main method, if we pass class file name as parameter and it will execute java code in executable jar file ,if we pass jar file as parameter while execute it.
Syntax for use:
ikvm [ options ] classname [ args1,args2…..argsN]
ikvm [ options ] -jar jarfile [args1,args2…..argsN]
Example :
ikvm -jar /usr/share/myprog.jar
Executes Java code in the executable jar file /usr/share/myprog.jar.
b) IKVM.NET ByteCode Compiler (ikvmc.exe) :
This is a Static compiler, Used to compile Java classes and jars into a .NET assembly ("static mode").This tool converts Java bytecode to .NET dll's and exe's. It converts the Java bytecodes in the input files to .NET DLL. So when we pass multiple jar files and class file it will combine and generate single .exe or dll file. exe or dll depends whether the class file and jar file that we have passed, having Main method or nor. If they have main method then it will generates exe otherwise DLL.
Syntax for use:
ikvmc [ options ] classOrJarfile [ classOrJarfile ... ]
Example :
ikvmc myProg.jar
Scans myprog.jar for a main method. If found, an .exe is produced; otherwise, a .dll is generated.
c) IKVM.NET Stub Generator (ikvmstub.exe) :
A tool that generates stub class files from a .NET assembly, so that Java code can be compiled against .NET code. The ikvmstub tool generates Java stubs from .NET assemblies. ikvmstub reads the specified assembly and generates a Java jar file containing Java interfaces and stub classes. For more information about the generated stubs. The tool uses the following algorithm to locate the assembly:
First it attempts to load the assembly from the default load context of ikvmstub.exe
. For practical purposes, this usually means it searches the Global Assembly Cache.
If not found in the default load context, ikvmstub looks for the assembly at the indicated path (or the current directory, if no path is supplied).
Syntax for use:
ikvmstub assemblyNameOrPath
Example :
ikvmstub c:\lib\mylib.dll
Generates mylib.jar
, containing stubs for classes, interfaces, etc., defined in c:\lib\mylib.dll.
We can use IKVM.NET by two ways:
1) Dynamically:
In this mode, Java classes and jars are used directly to execute Java applications on the .NET runtime. The full Java class loader model is supported in this mode.
2) Statically:
In order to allow Java code to be used by .NET applications, it must be compiled down to a DLL and used directly. The assemblies can be referenced directly by the .NET applications and the "Java" objects can be used as if they were .NET objects. While the static mode does not support the full Java class loader mechanism, it is possible for statically-compiled code to create a class loader and load classes dynamically.
Uses for IKVM.NET
IKVM.NET is useful for several different software development scenarios. Here is a sampling of some of the possibilities.
1) Drop in JVM :
The IKVM application included with the distribution is a .NET implementation of a Java Virtual Machine. In many cases, you can use it as a drop-in replacement for java. For example, instead of typing
java -jar myapp.jar
to run an application, you can type
ikvm -jar myapp.jar
2) Use Java libraries in your .NET application
IKVM.NET includes ikvmc, a Java bytecode
to .NET IL translator. If you have a Java library that you would like to use in a .NET application, run ikvmc -target:library mylib.jar
to create mylib.dll.
For example, the Apache FOP project is an open source XSL-FO processor written in Java that is widely used to generate PDF documents from XML source. With IKVM.NET technology, Apache FOP can be used by any .NET application.
3) Develop .NET applications in java
IKVM provides a way for you to develop .NET applications in Java. Although IKVM.NET does not include a Java compiler for .NET, you can use any Java compiler to compile Java source code to JVM bytecode, then use ikvmc -target:exe myapp.jar to produce a .NET executable. You can even use .NET API's in your Java code using the included ikvmstub application.
Example :
Now lets see how we can use class file or Library created in java in .NET. Here I am creating a class file and library in java for displaying welcome and goodbye message.We have following code in java file:
package Msg;
public class Message {
public static void WelcomeMsg(String msg) {
System.out.println("Welcome"+msg);
}
public static void GoodByeMsg(String msg) {
System.out.println("Good Bye"+msg);
}
}
Now compile this Java file into class file, so we have Message.class file. I have also created Message.jar file because i am going to show how to use class file or jar file to generate DLL file using IKVM.NET. By following way we can generate jar file from class file:
jar cvf Message.jar Message.class
Now we need to generate Message DLL from class file or jar file. so we need to use IKVMC for this. you can download IKVM.NET from here.
After downloading this zip file ,when you unzip it, you can see bin folder. Inside bin folder you can find all necessary files. Now copy your class file or jar (Message.class
or Message.jar
file) inside bin folder. Open your command prompt and go to this bin folder by cd command.
Type following command for generating DLL file from class file or jar file.
ikvmc -target:library Message.jar
ikvmc -target:library Message.class
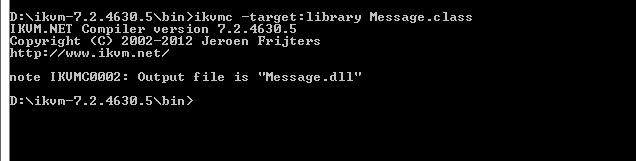

You can use either one of command to generate dll file.Now you can found Message.dll
file inside bin folder.
Create a console Project in c#.net.Add reference of Message.dll
and IKVM.OpenJDK.Core.dll
.Write following code in your project to access methods of this dll.
using Msg;
namespace MessageDemo
{
class Program
{
static void Main(string[] args)
{
Message.WelcomeMsg(" Abhishek");
Console.WriteLine("Now you can start your work............");
Message.GoodByeMsg(" Abhishek");
}
}
}
Now you can run your project. you will get following output :

Points of Interest
IKVM.NET is very interesting library for developers who knows both C# and Java language ,they can understand how much useful it is. If they have worked on one language and created some important library and after this they want to use in another language then they can use it very easily using IKVM.NET.
References
http://www.ikvm.net/
I like to code and I really enjoy to share my knowledge with all, Its my passion.
http://abhishekgoswami.com/