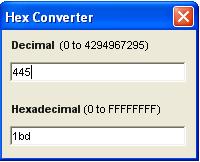
Introduction
This article is about how you would go about converting numeric values to hexadecimal and vice versa. The main reason why I did this program is to help me to cheat when playing computer games, mainly role-playing ones cos I normally need to find the correct hex data in those save game file and furthermore I do not have a good hex editor to help me do those conversion. :)
Using the code
The main code used for the conversion are from the System.Convert
class. Below is the code that is used to convert a string to unsigned integer while checking for overflow, and then convert it to hexadecimal format before showing it as a string in a text box.
uint uiDecimal = 0;
try
{
uiDecimal = checked((uint)System.Convert.ToUInt32(tbDecimal.Text));
}
catch (System.OverflowException exception)
{
tbHex.Text = "Overflow";
return;
}
tbHex.Text = String.Format("{0:x2}", uiDecimal);
and vice versa
uint uiHex = 0;
try
{
uiHex = System.Convert.ToUInt32(tbHex.Text, 16);
}
catch (System.OverflowException exception)
{
tbDecimal.Text = "Overflow";
return;
}
tbDecimal.Text = uiHex.ToString();
Conclusion
This is my first attempt at C# and WinForms so I believe by starting with such simple and small programs, it will eventually build up my skills and knowledge to attempt bigger ones. :)
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.