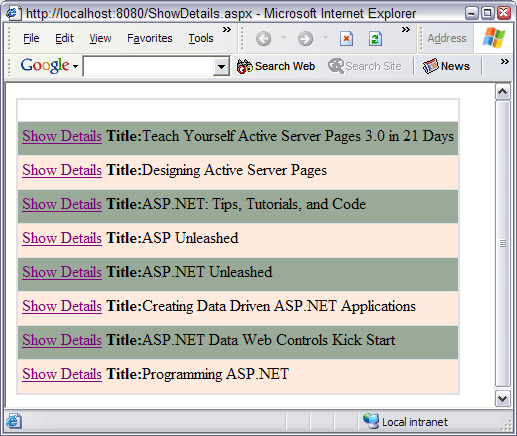

Introduction
I wanted to find a way to show details of a datagrid
row in the same row as opposed to binding a new query to a separate datagrid
. To do this, I had to bind my query results to a datagrid
, hide the results that I did not want displayed behind a style
tag, and then toggle the style
as needed to show or hide the results.
Overview
Using a simple Page_Load
event to bind XML data to a datagrid
and display the results.
public void Page_Load(Object sender, EventArgs e)
{
string books = Server.MapPath("aspnetbooks.xml");
DataSet dataSet = new DataSet();
dataSet.ReadXml(books);
dgBooks.DataSource = dataSet;
dgBooks.DataBind();
}
Once the data is displayed, use the JavaScript below in combination with the datagrid
to manipulate the unique Id
s and show or hide the details.
Using the Code
JavaScript Code
This script first determines the browser version being used in order to then ascertain which method it should use to manipulate the elements in the HTML. Next, it loops through the arguments and sets up an element
object to change the visibility to hidden
or visible
. The following code illustration shows the DataGrid
itself and then explains the details behind calling the showDetails
function.
<script type="text/javascript">
var action;
IE4 = (document.all && !document.getElementById) ? true : false;
NS4 = (document.layers) ? true : false;
IE5 = (document.all && document.getElementById) ? true : false;
NS6 = (!document.all && document.getElementById) ? true : false;
function showDetails(action)
{
x = 0;
changeText(arguments[x]);
if (IE4) { prefix = document.all(arguments[x]).style; }
if (NS6 || IE5) { prefix = document.getElementById(arguments[x]).style }
if (NS4){ prefix = document.layers(arguments[x]); }
if (prefix.visibility == "visible")
{
prefix.visibility = "hidden";
prefix.fontSize = "0";
}
else
{
prefix.visibility = "visible";
prefix.fontSize = "16";
}
}
function changeText(link)
{
link += "link";
linkText = document.getElementById(link);
linkText.firstChild.nodeValue;
if (linkText.firstChild.nodeValue == "Show Details")
{
linkText.firstChild.nodeValue = "Hide Details";
}
else
{
linkText.firstChild.nodeValue = "Show Details";
}
}
</script>
DataGrid Code
This datagrid
is pretty simple. autoGenerateColumns
is set to False
in order to manipulate the columns manually. First, notice line #9. This is where the showDetails
function is called. Using the DataBinder.Eva
l to bind a unique database record ID to each function; that is the same record ID used on line #20-#22 to set the id of a cell in the table and the same unique ID assigned to the "a href
" tag except it has "link
" appended to the ID to keep it unique.
Notice that Row #14 has a style that sets the font-size to "0
" and the visibility to hidden. This is what the JavaScript above will toggle. The URL is set to call "showDetails('UniqueID of the row')
". Once the function has performed browser detection, a call is made to another function: changeText('Unique ID of the row')
. This function appends the "link
" to the ID and changes the text to either "Show Details" or "Hide Details" by checking the innerText
of the href
tag. The showDetails
function then resumes by changing the visibility and font-size
style according to its current state.
1. <asp:DataGrid id="dgBooks" runat="server" Height="35px"
autoGenerateColumns="False" BorderWidth="2px">
2. <AlternatingItemStyle forecolor="#99CC99"
backcolor= "#FFEADE"></AlternatingItemStyle>
3. <ItemStyle forecolor="#FFCC99" backcolor= "#99AA99"></ItemStyle>
4. <Columns>
5. <asp:TemplateColumn>
6. <ItemTemplate>
7. <table border="0" id="Table1">
8. <tr>
9. <td>
10. <a id='<%# DataBinder.Eval(
Container.DataItem, "bookID") %>link'
href="javascript:showDetails('<%# DataBinder.Eval(
Container.DataItem, "bookID") %>')">
History
- 8th July, 2003: Initial version
License
This article has no explicit license attached to it, but may contain usage terms in the article text or the download files themselves. If in doubt, please contact the author via the discussion board below. A list of licenses authors might use can be found here.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.