Introduction
When it comes to access Microsoft's Active Directory using C#, a lot of people get confused how to get started. This article attempts to show you how to communicate with active directory using C# in a simple way. I make a simple web application interact with active directory using ASP.NET MVC .This application performs only three operations on active directory:
- get all users
- get all groups
- reset password for users
Background
You should have some basic knowledge with ASP.NET MVC.
Active Directory
- Install Windows Server 2012 R2.
- Install Active Directory Domain Service.
- Create new domain in a new forest. I named it “
MBS.Com
”. - Add organizational unit and named it “
DevOU
. - Add some users and groups in OU.
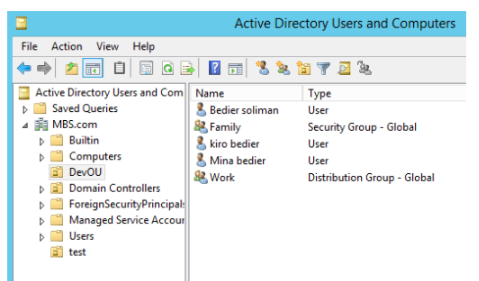
Using the Code
There are two namespaces to communicate Active Directory with C#:
System.DirectoryServices.ActiveDirectory
System.DirectoryServices.AccountManagement
(this is what I used)
Note
In my case, IIS server and directory domain controller reside on the same machine to run task successfully.
- Add Action called
HomePage
in your Controller containing two buttons, one for Users and other for Groups.
public ActionResult HomePage()
{
return View();
}
- Add View for this Action:
@{
ViewBag.Title = "HomePage";
}
@*<h2>HomePage</h2>*@
<br />
<br />
<div class="row text-center">
<div class="col lg-6">
@Html.ActionLink
("Users", "GetAllUsers", "Home", null, new { @class = "btn btn-primary" })
@Html.ActionLink
("Groups", "GetAllGroups", "Home", null, new { @class = "btn btn-primary" })
</div>
</div>

- Add two Classes for User and Group in Models Folder:
public class User
{
public int Id { get; set; }
[Display(Name = "Display Name")]
public string DisplayName { get; set; }
public string Samaccountname { get; set; }
}
public class Group
{
public int Id { get; set; }
[Display(Name = "Group Name")]
public string GroupName { get; set; }
}
- Then we will implement three methods which will be performed on Active Directory: Get All Users, Get All Groups, Set Password:
public ActionResult GetAllUsers()
{
List<User> ADUsers = GetallAdUsers();
return View(ADUsers);
}
public ActionResult GetAllGroups()
{
List<Group> ADGroups = GetallGroups();
return View(ADGroups);
}
public static List<User> GetallAdUsers()
{
List<User> AdUsers = new List<User>();
var ctx = new PrincipalContext(ContextType.Domain, "MBS","OU=DevOU,DC=MBS,DC=com");
UserPrincipal userPrin = new UserPrincipal(ctx);
userPrin.Name = "*";
var searcher = new System.DirectoryServices.AccountManagement.PrincipalSearcher();
searcher.QueryFilter = userPrin;
var results = searcher.FindAll();
foreach (Principal p in results)
{
AdUsers.Add(new User { DisplayName = p.DisplayName,
Samaccountname = p.SamAccountName });
}
return AdUsers;
}
public ActionResult ResetPassword(string Samaccountname)
{
PrincipalContext context = new PrincipalContext
(ContextType.Domain, "MBS", "OU=DevOU,DC=MBS,DC=com");
UserPrincipal user = UserPrincipal.FindByIdentity
(context, IdentityType.SamAccountName, Samaccountname);
user.Enabled = true;
string newPassword = "P@ssw0rd";
user.SetPassword(newPassword);
user.ExpirePasswordNow();
user.Save();
TempData["msg"] = "<script>alert('Password Changed Successfully');</script>";
return RedirectToAction("GetAllUsers");
}
public static List<Group> GetallGroups()
{
List<Group> AdGroups = new List<Group>();
var ctx = new PrincipalContext(ContextType.Domain, "MBS", "OU=DevOU,DC=MBS,DC=com");
GroupPrincipal _groupPrincipal = new GroupPrincipal(ctx);
PrincipalSearcher srch = new PrincipalSearcher(_groupPrincipal);
foreach (var found in srch.FindAll())
{
AdGroups.Add(new Group { GroupName = found.ToString() });
}
return AdGroups;
}
- Then Add view for
GetAllUsers
action.
@model IEnumerable<ActiveDirectory.Models.User>
@{
ViewBag.Title = "GetAllUsers";
}
<br />
<form>
<div class="form-group">
<label for="SearchInput" class="col-sm-2 col-form-label">Search for User</label>
<div class="col-md-10">
<input type="text" class="form-control" id="SearchInput"
onkeyup="myFunction()" placeholder="Enter User">
</div>
</div>
</form>
<br />
<br />
@Html.Raw(TempData["msg"])
<table class="table table-bordered table-striped" id="tblUsers">
<tr>
<th>
@Html.DisplayNameFor(model => model.DisplayName)
</th>
<th>
@Html.DisplayNameFor(model => model.Samaccountname)
</th>
<th></th>
</tr>
@foreach (var item in Model)
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.DisplayName)
</td>
<td>
@Html.DisplayFor(modelItem => item.Samaccountname)
</td>
<td>
@Html.ActionLink("Reset Password", "ResetPassword",
new { Samaccountname = item.Samaccountname })
</td>
</tr>
}
</table>
@section scripts
{
<script>
function myFunction() {
var input, filter, table, tr, td, i;
input = document.getElementById("SearchInput");
filter = input.value.toUpperCase();
table = document.getElementById("tblUsers");
tr = table.getElementsByTagName("tr");
for (i = 0; i < tr.length; i++) {
td = tr[i].getElementsByTagName("td")[0];
if (td) {
if (td.innerHTML.toUpperCase().indexOf(filter) > -1) {
tr[i].style.display = "";
} else {
tr[i].style.display = "none";
}
}
}
}
</script>
}


- Add another view for
GetAllGroups
Action.
@model IEnumerable<ActiveDirectory.Models.Group>
@{
ViewBag.Title = "GetAllGroups";
}
<br />
<table class="table table-striped table-bordered">
<tr>
<th>
@Html.DisplayNameFor(model => model.GroupName)
</th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.GroupName)
</td>
</tr>
}
</table>

Notes
All these functionalities work on specific organizational unit I have created “DevOU
”.
To get All Users and groups of Active Directory, just remove “OU
” from path in the context.

History
- 7th March, 2019: Initial version
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.