Introduction
Hello friends, in this article we are going to learn two things related to images and file upload control. This article is for complete beginners who are
working with File Upload control and images and do not know much about the below mentioned points. I have divided this article into
three sections:
- Finding the height and width of an image.
- Appending a time stamp with the image name while saving it.
- Adding a watermark on an image while uploading it.
First, before looking at the code, this is how my sample page looks like...
Image of my sample application
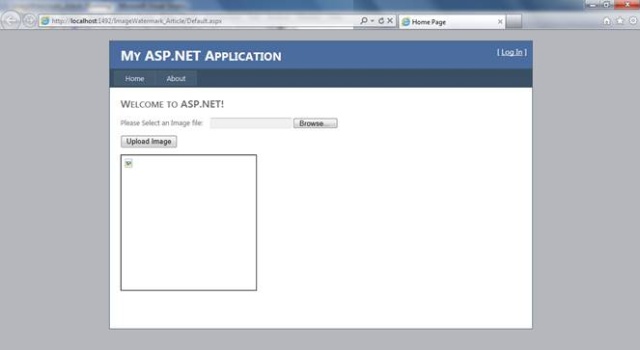
Explanation
- Finding the height and width of an image – Sometimes in our applications we allow our users to upload their images.
And in some cases we want them to upload an image of a specific dimension. For example – 450x900.
Below is the code for finding the height and width.
Code to check the height and width of an image – Default.aspx page code
<%@ Page Title="Home Page" Language="C#" MasterPageFile="~/Site.master"
AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<asp:Content ID="HeaderContent" runat="server" ContentPlaceHolderID="HeadContent">
</asp:Content>
<asp:Content ID="BodyContent" runat="server" ContentPlaceHolderID="MainContent">
<h2>
Welcome to ASP.NET!
</h2>
<p>
Please Select an Image file:
<asp:FileUpload ID="FUP_WatermarkImage" runat="server" />
</p>
<p>
<asp:Button ID="btnUpload" runat="server" Text="Upload Image" onclick="btnUpload_Click" />
</p>
<p>
<asp:Image ID="imgUploadedImage" runat="server" Width="250"
Height="250" BorderColor="Black" BorderStyle="Solid" BorderWidth="1" />
</p>
</asp:Content>
Note – Please note that in the ASP.NET Image
control I have kept the width and height as 250 for both. So in my code I am going to check the images
whether its height and width exceeds 250*250 dimension. If it exceeds, then I’ll show an error message to the
user and won’t allow him to upload the image.
protected void btnUpload_Click(object sender, EventArgs e)
{
if (Fupload_WatermarkImage.HasFile)
{
string FileType = Path.GetExtension(Fupload_WatermarkImage.PostedFile.FileName).ToLower().Trim();
if (FileType != ".jpg" && FileType != ".png" && FileType != ".gif" && FileType != ".bmp")
{
string alert = "alert('File Format Not Supported. Only .jpg, .png, .bmp and .gif file formats are allowed.');";
ScriptManager.RegisterStartupScript(this, GetType(), "JScript", alert, true);
}
else
{
System.Drawing.Image UploadedImage = System.Drawing.Image.FromStream(Fupload_WatermarkImage.PostedFile.InputStream);
if (UploadedImage.PhysicalDimension.Width > 250 || UploadedImage.PhysicalDimension.Height > 250)
{
string alert = "alert('Image size should be exactly 250*250 dimension. Your Current Image width is " +
UploadedImage.PhysicalDimension.Width + " and height is " +
UploadedImage.PhysicalDimension.Height + ".');";
ScriptManager.RegisterStartupScript(this, GetType(), "JScript", alert, true);
}
else
{
}
}
}
}
User tries to upload image with a dimension of more than 250 pixels
Image indicating the width of the uploaded image–

Image indicating the height of the uploaded image–

Error message shown to the user

- Appending a time stamp with the image name while saving it – Sometimes a
user might add an image with the same name twice. In that case if
you want to differentiate between the images, then you can attach the current timestamp with the image name. Below is the code for doing the same.
string FileName = Path.GetFileNameWithoutExtension(Fupload_WatermarkImage.PostedFile.FileName);
string FileExtension = Path.GetExtension(Fupload_WatermarkImage.PostedFile.FileName);
string path = "Watermarked Images/" + FileName +
DateTime.Now.ToString("yyyy-MM-dd HHmmtt") + FileExtension;
Fupload_WatermarkImage.SaveAs(Server.MapPath(path));
imgUploadedImage.ImageUrl = path;
if (!String.IsNullOrEmpty(imgUploadedImage.ImageUrl))
{
string alert = "alert('Image uploaded successfully');";
ScriptManager.RegisterStartupScript(this, GetType(), "JScript", alert, true);
}
Note – You can easily understand the code since I have added the appropriate comments with each line of my code. The line of code marked in
yellow is where I am attaching my current time stamp with the image name. Below is the image which shows how exactly the image is saved.
Selected an image with height and width less than 250 pixels

Code debugged

The current time is attached with the image name. So the next time even if I upload an image with the same name, I can still upload it since the timestamp differentiates it.
Final output – Image being displayed in the Image control

Image uploaded in the folder–

- Watermarking an image – Sometimes when we add images from our application, we do not
want other users to
copy it or use it in their applications. Watermarking is the option which helps protect our images from getting copied or used by other users. Below is
the code written to add watermark on an image before saving it in a folder.
Code for watermarking – Content marked in yellow actually writes / watermarks the image.
System.Drawing.Image image =
System.Drawing.Image.FromStream(Fupload_WatermarkImage.PostedFile.InputStream);
int width = image.Width;
int height = image.Height;
System.Drawing.Bitmap bmp = new System.Drawing.Bitmap(width, height);
System.Drawing.Graphics graphics1 = System.Drawing.Graphics.FromImage((System.Drawing.Image)bmp);
graphics1.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode.High;
graphics1.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.HighQuality;
graphics1.Clear(System.Drawing.Color.Transparent);
graphics1.DrawImage(image, 0, 0, width, height);
System.Drawing.Font font = new System.Drawing.Font("Arial", 20);
System.Drawing.SolidBrush brush = new System.Drawing.SolidBrush(System.Drawing.Color.Aqua);
graphics1.DrawString("C Sharp Corner", font, brush, 25F, 115F);
System.Drawing.Image newImage = (System.Drawing.Image)bmp;
string FileName = Path.GetFileNameWithoutExtension(Fupload_WatermarkImage.PostedFile.FileName);
string FileExtension = Path.GetExtension(Fupload_WatermarkImage.PostedFile.FileName);
string path = "Watermarked Images/" + FileName + DateTime.Now.ToString("yyyy-MM-dd HHmmtt") + FileExtension;
newImage.Save(Server.MapPath(path));
imgUploadedImage.ImageUrl = path;
graphics1.Dispose();
if (!String.IsNullOrEmpty(imgUploadedImage.ImageUrl))
{
string alert = "alert('Image uploaded successfully');";
ScriptManager.RegisterStartupScript(this, GetType(), "JScript", alert, true);
}
Note – For understanding the code, I have added the appropriate comments with the code.
Final output of the above code – The watermark appears on the image in Cyan (Blue) color. The text is “C# Corner”.

Image saved in the folder with watermark–


So this was a simple application where we played with images while uploading it to
a folder. Hope you liked it and that this article helped you... Don’t forget to
leave your comments. It always encourages me to move ahead... :-)
If you want to check the entire code, please find the attached code file with this article.