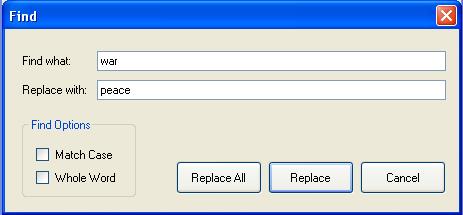
Introduction
This article describes how to create a find and replace method for the RichTextBox control. It is easy to understand. If you develop a word processing application this method will be a grate gadget for you. You can create any method for any control like this.
How To Create It
1. First, create a new project and name it "FRRichTextBox"
2. Then we need to create a class RichTextBoxFR.vb. to create it, click on Add New Item button on the Standard Toolbar.
3. Select Class from the template and name it RichTextBoxFR.vb and Click on Add button.
4. We use inherits statement and derive our class from RichTextBox Class
<FONT color=#0000ff size=2>Public Class</FONT><FONT size=2> RichTextBoxFR</FONT>
<FONT color=#0000ff> Inherits </FONT>RichTextBox
<FONT color=#0000ff>End Class</FONT>
5. We create a FindAndReplace method with two arguments - FindText and ReplaceText.
<FONT color=#0000ff size=2>Public Class</FONT><FONT size=2> RichTextBoxFR</FONT>
<FONT color=#0000ff>Inherits</FONT> RichTextBox
<FONT color=#0000ff>Public Sub</FONT> FindAndReplace(<FONT color=#0000ff>ByVal</FONT> FindText <FONT color=#0000ff>As</FONT> <FONT color=#0000ff>String</FONT>, <FONT color=#0000ff>ByVal</FONT> ReplaceText <FONT color=#0000ff>As String</FONT>)
<FONT color=#0000ff>Me</FONT>.Find(FindText)
<FONT color=#0000ff>If Not Me</FONT>.SelectionLength = 0 <FONT color=#0000ff>Then</FONT>
<FONT color=#0000ff>Me</FONT>.SelectedText = ReplaceText
<FONT color=#0000ff>Else</FONT>
MsgBox("The following specified text was not found: " & FindText)
<FONT color=#0000ff>End If</FONT>
<FONT color=#0000ff>End Sub</FONT>
<FONT color=#0000ff>End Class</FONT>
Me here is RichTextBox. We use Me.Find(FindText) to search the text of the RichTextBox control and select the text. If the search text is found, RichTextBox select it and SelectionLength of RichTextBox will be > 0. Then we use if else statement to replace that text as above.
6. Now we create another FindAndReplace method by overloading our previous FindAndReplace method. You can see that method has five arguments - FindText, ReplaceText, ReplaceAll, MatchCase and WholeWord
<FONT color=#0000ff size=2>Public Class</FONT><FONT size=2> RichTextBoxFR</FONT>
<FONT color=#0000ff>Inherits</FONT> RichTextBox
<FONT color=#0000ff>Public Sub</FONT> FindAndReplace(<FONT color=#0000ff>ByVal</FONT> FindText <FONT color=#0000ff>As</FONT> <FONT color=#0000ff>String</FONT>, <FONT color=#0000ff>ByVal</FONT> ReplaceText <FONT color=#0000ff>As String</FONT>)
<FONT color=#0000ff>Me</FONT>.Find(FindText)
<FONT color=#0000ff>If Not Me</FONT>.SelectionLength = 0 <FONT color=#0000ff>Then</FONT>
<FONT color=#0000ff>Me</FONT>.SelectedText = ReplaceText
<FONT color=#0000ff>Else</FONT>
MsgBox("The following specified text was not found: " & FindText)
<FONT color=#0000ff>End If</FONT>
<FONT color=#0000ff>End Sub</FONT>
<FONT color=#0000ff>Public Sub</FONT> FindAndReplace(<FONT color=#0000ff>ByVal</FONT> FindText <FONT color=#0000ff>As String</FONT>, <FONT color=#0000ff>ByVal</FONT> ReplaceText <FONT color=#0000ff>As String</FONT>, <FONT color=#0000ff>ByVal</FONT> ReplaceAll <FONT color=#0000ff>As Boolean</FONT>, _
<FONT color=#0000ff>ByVal</FONT> MatchCase <FONT color=#0000ff>As Boolean</FONT>, <FONT color=#0000ff>ByVal</FONT> WholeWord <FONT color=#0000ff>As Boolean</FONT>)
<FONT color=#0000ff> Select Case</FONT> ReplaceAll
<FONT color=#0000ff>Case False</FONT>
<FONT color=#0000ff>If</FONT> MatchCase = <FONT color=#0000ff>True</FONT> <FONT color=#0000ff>Then</FONT>
<FONT color=#0000ff>If</FONT> WholeWord = <FONT color=#0000ff>True</FONT> <FONT color=#0000ff>Then</FONT>
<FONT color=#0000ff>Me</FONT>.Find(FindText, RichTextBoxFinds.MatchCase <FONT color=#0000ff>Or</FONT> RichTextBoxFinds.WholeWord)
<FONT color=#0000ff>Else</FONT>
<FONT color=#0000ff>Me</FONT>.Find(FindText, RichTextBoxFinds.MatchCase)
<FONT color=#0000ff>End If</FONT>
<FONT color=#0000ff>Else</FONT>
<FONT color=#0000ff>If</FONT> WholeWord = <FONT color=#0000ff>True</FONT> <FONT color=#0000ff>Then</FONT>
<FONT color=#0000ff>Me</FONT>.Find(FindText, RichTextBoxFinds.WholeWord)
<FONT color=#0000ff>Else</FONT>
<FONT color=#0000ff>Me</FONT>.Find(FindText)
<FONT color=#0000ff>End If</FONT>
<FONT color=#0000ff>End If</FONT>
<FONT color=#0000ff>If Not</FONT> <FONT color=#0000ff>Me</FONT>.SelectionLength = 0 <FONT color=#0000ff>Then</FONT>
<FONT color=#0000ff>Me</FONT>.SelectedText = ReplaceText
<FONT color=#0000ff>Else</FONT>
MsgBox("The following specified text was not found: " & FindText)
<FONT color=#0000ff>End If
</FONT>
<FONT color=#0000ff>Case True</FONT>
<FONT color=#0000ff>Dim</FONT> i <FONT color=#0000ff>As Integer</FONT>
<FONT color=#0000ff>For</FONT> i = 0 <FONT color=#0000ff>To Me</FONT>.TextLength <FONT color=#008000>'We know that strings we have to replace < TextLength of RichTextBox</FONT>
<FONT color=#0000ff>If</FONT> MatchCase = <FONT color=#0000ff>True Then</FONT>
<FONT color=#0000ff>If</FONT> WholeWord = <FONT color=#0000ff>True Then</FONT>
<FONT color=#0000ff>Me</FONT>.Find(FindText, RichTextBoxFinds.MatchCase <FONT color=#0000ff>Or</FONT> RichTextBoxFinds.WholeWord)
<FONT color=#0000ff>Else</FONT>
<FONT color=#0000ff>Me</FONT>.Find(FindText, RichTextBoxFinds.MatchCase)
<FONT color=#0000ff>End If</FONT>
<FONT color=#0000ff>Else</FONT>
<FONT color=#0000ff>If</FONT> WholeWord = <FONT color=#0000ff>True</FONT> <FONT color=#0000ff>Then</FONT>
<FONT color=#0000ff>Me</FONT>.Find(FindText, RichTextBoxFinds.WholeWord)
<FONT color=#0000ff>Else</FONT>
<FONT color=#0000ff>Me</FONT>.Find(FindText)
<FONT color=#0000ff>End If</FONT>
<FONT color=#0000ff>End If</FONT>
<FONT color=#0000ff>If</FONT> <FONT color=#0000ff>Not</FONT> Me.SelectionLength = 0 <FONT color=#0000ff>Then</FONT>
<FONT color=#0000ff>Me</FONT>.SelectedText = ReplaceText
<FONT color=#0000ff>Else</FONT>
MsgBox(i & " occurrence(s) replaced")
<FONT color=#0000ff>Exit For</FONT>
<FONT color=#0000ff>End If</FONT>
<FONT color=#0000ff>Next</FONT> i
<FONT color=#0000ff>End Select
End Sub</FONT>
<FONT color=#0000ff>End Class</FONT>
7. We can use RichTextBoxFinds enumerations (which enables us to specify how the search is performed when the Find method is called)such as MatchCase, WholeWord, for advance search by thinking logically.
8. When we use ReplaceAll = True, we do not know how many strings we have to replace, but we know that Strings we have to replace < textlength of the richtextbox. We use For Loop statement to implement it.
How To Use It
Now we have created our RichTextBoxFR control.
9. Select Build Menu --> Build Solution
10. Select RichTextBoxFR from the ToolBox and draw it on the form.
11. Create a new form as above and name it frmFind. This form has
i. Two TextBoxes :- FindTextBox and ReplaceTextBox
ii. Three Buttons :- btnReplaceAll, btnReplace and btnCancel
iii. Two CheckBoxes :- MatchCase, WholeWord
12. Here are the codes for the form
<FONT color=#0000ff>Public Class</FONT> frmFind
<FONT color=#0000ff>Private Sub</FONT> btnReplaceAll_Click(<FONT color=#0000ff>ByVal</FONT> sender <FONT color=#0000ff>As System.Object</FONT>, <FONT color=#0000ff>ByVal</FONT> e <FONT color=#0000ff>As System.EventArgs</FONT>) <FONT color=#0000ff>Handles</FONT> btnReplaceAll.Click
<FONT color=#0000ff>If</FONT> MatchCase.Checked <FONT color=#0000ff>Then</FONT>
<FONT color=#0000ff>If</FONT> WholeWord.Checked <FONT color=#0000ff>Then</FONT>
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, <FONT color=#0000ff>True</FONT>, <FONT color=#0000ff>True</FONT>, <FONT color=#0000ff>True</FONT>)
<FONT color=#0000ff>Else</FONT>
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, <FONT color=#0000ff>True</FONT>, <FONT color=#0000ff>True</FONT>, <FONT color=#0000ff>False</FONT>)
<FONT color=#0000ff>End If</FONT>
<FONT color=#0000ff>Else</FONT> <FONT color=#008000>' If FindMe.MatchCase.Checked = False</FONT>
<FONT color=#0000ff>If</FONT> WholeWord.Checked <FONT color=#0000ff>Then</FONT>
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, <FONT color=#0000ff>True</FONT>, <FONT color=#0000ff>False</FONT>, <FONT color=#0000ff>True</FONT>)
<FONT color=#0000ff>Else</FONT>
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, <FONT color=#0000ff>True</FONT>, <FONT color=#0000ff>False</FONT>, <FONT color=#0000ff>False</FONT>)
<FONT color=#0000ff>End If</FONT>
<FONT color=#0000ff>End If</FONT> <FONT color=#008000>'End FindMe.MatchCase.Checked </FONT>
<FONT color=#0000ff>End Sub</FONT>
<FONT color=#0000ff>Private Sub</FONT> btnReplace_Click(<FONT color=#0000ff>ByVal</FONT> sender <FONT color=#0000ff>As System.Object</FONT>, <FONT color=#0000ff>ByVal</FONT> e <FONT color=#0000ff>As</FONT> <FONT color=#0000ff>System.EventArgs</FONT>) <FONT color=#0000ff>Handles</FONT> btnReplace.Click
<FONT color=#0000ff>If</FONT> MatchCase.Checked <FONT color=#0000ff>Then</FONT>
<FONT color=#0000ff>If</FONT> WholeWord.Checked <FONT color=#0000ff>Then</FONT>
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, <FONT color=#0000ff>False</FONT>, <FONT color=#0000ff>True</FONT>, <FONT color=#0000ff>True</FONT>)
<FONT color=#0000ff>Else</FONT>
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, <FONT color=#0000ff>False</FONT>, <FONT color=#0000ff>True</FONT>, <FONT color=#0000ff>False</FONT>)
<FONT color=#0000ff> End If</FONT>
<FONT color=#0000ff>Else</FONT> <FONT color=#008000>'If FindMe.MatchCase.Checked = False</FONT>
<FONT color=#0000ff>If</FONT> WholeWord.Checked <FONT color=#0000ff>Then</FONT>
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, <FONT color=#0000ff>False</FONT>, <FONT color=#0000ff>False</FONT>, <FONT color=#0000ff>True</FONT>)
<FONT color=#0000ff>Else</FONT>
frmMain.RichTextBoxFR1.FindAndReplace(FindTextBox.Text, ReplaceTextBox.Text, <FONT color=#0000ff>False</FONT>, <FONT color=#0000ff>False</FONT>, <FONT color=#0000ff>False</FONT>)
<FONT color=#0000ff>End If</FONT>
<FONT color=#0000ff>End If</FONT> <FONT color=#008000>'End FindMe.MatchCase.Checked </FONT>
<FONT color=#0000ff>End Sub</FONT>
<FONT color=#0000ff>End Class</FONT>
History
created - 17 March 2006