Introduction
in that Article i will manage XML Schema File by selecting all the schema file elements and
display them in DropDownList , Adding Elements to root element and select elemet to add an
attributes to the selected element
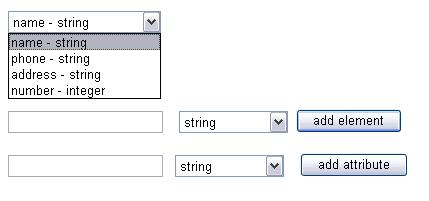
Background
First you must know some information abut xml and xmls schema .
Using the code
public void load_element()that funcation load the element and display them in
DropDownList
public void load_element()
{
DropDownList_elements.Items.Clear();
XmlTextReader reader = new XmlTextReader(Server.MapPath("person.xsd"));
XmlSchema my_xmlschema = new XmlSchema();
my_xmlschema = XmlSchema.Read(reader, null);
reader.Close();
XmlSchemaElement my_xmlschemaelement = new XmlSchemaElement();
int counter = my_xmlschema.Items.Count;
for (int i = 0; i < counter; i++)
{
XmlSchemaElement current_element = (XmlSchemaElement)my_xmlschema.Items[i];
if (current_element.Name.ToString() == "person")
{
XmlSchemaComplexType current_element_complexType = (XmlSchemaComplexType)current_element.SchemaType;
XmlSchemaSequence current_elemen_sequence = (XmlSchemaSequence)current_element_complexType.Particle;
foreach ( XmlSchemaObject my_XmlSchemaObject in current_elemen_sequence.Items)
{
XmlSchemaElement my_XmlElement = (XmlSchemaElement)my_XmlSchemaObject;
string[] TypeName = my_XmlElement.SchemaTypeName.ToString().Split(':');
DropDownList_elements.Items.Add(my_XmlElement.Name.ToString() + " " + "-" + " " + TypeName[2]);
}
}
}
DropDownList_elements.DataBind();
}
Button_add_element : that button get the element name from TextBox and type from
drodownlist and add it in root element then call the void load_element() funcation to load the
element aftar adding the new element .
protected void Button_add_element_Click(object sender, EventArgs e)
{
XmlTextReader reader = new XmlTextReader(Server.MapPath("person.xsd"));
XmlSchema my_xmlschema = new XmlSchema();
my_xmlschema = XmlSchema.Read(reader, null);
reader.Close();
XmlSchemaElement my_xmlschemaelement = new XmlSchemaElement();
int counter = my_xmlschema.Items.Count;
for (int i = 0; i < counter; i++)
{
XmlSchemaElement current_element = (XmlSchemaElement)my_xmlschema.Items[i];
if (current_element.Name.ToString() == "person")
{
XmlSchemaComplexType current_element_complexType = (XmlSchemaComplexType)current_element.SchemaType;
XmlSchemaSequence current_elemen_sequence = (XmlSchemaSequence)current_element_complexType.Particle;
XmlSchemaElement new_element = new XmlSchemaElement();
new_element.Name = TextBox_element_name.Text;
new_element.SchemaTypeName = new XmlQualifiedName(DropDownList_type.SelectedValue.ToString(), <a href="http://www.w3.org/2001/XMLSchema);">http:
XmlSchemaObject new_element_object = (XmlSchemaObject)new_element;
current_elemen_sequence.Items.Add(new_element_object);
}
}
FileStream writer = new FileStream(Server.MapPath("person.xsd"), System.IO.FileMode.Create);
my_xmlschema.Write(writer);
writer.Close();
load_element();
}
Button_add_attribute : that button get element name from textbox and type from
drodownlist and add the attribute to the selected element and cheak if the selected element dont have attribute creat new complex type and add the attribute in and if the element have
an attribut add the element in the current complex type.
protected void Button_add_attribute_Click(object sender, EventArgs e)
{
XmlTextReader reader = new XmlTextReader(Server.MapPath("person.xsd"));
XmlSchema my_xmlschema = new XmlSchema();
my_xmlschema = XmlSchema.Read(reader, null);
reader.Close();
XmlSchemaElement my_xmlschemaelement = new XmlSchemaElement();
int counter = my_xmlschema.Items.Count;
for (int i = 0; i < counter; i++)
{
XmlSchemaElement current_element = (XmlSchemaElement)my_xmlschema.Items[i];
if (current_element.Name.ToString() == "person")
{
XmlSchemaComplexType current_element_complexType = (XmlSchemaComplexType)current_element.SchemaType;
XmlSchemaSequence current_elemen_sequence = (XmlSchemaSequence)current_element_complexType.Particle;
foreach (XmlSchemaObject my_XmlSchemaObject in current_elemen_sequence.Items)
{
XmlSchemaElement my_XmlElement = (XmlSchemaElement)my_XmlSchemaObject;
string[] ElementName = DropDownList_elements.SelectedValue.ToString().Split(' ');
if (my_XmlElement.Name.ToString() == ElementName[0])
{
if (my_XmlElement.SchemaType == null )
{
XmlSchemaComplexType new_XmlSchemaComplexType = new XmlSchemaComplexType();
XmlSchemaAttribute new_XmlSchemaAttribute = new XmlSchemaAttribute();
new_XmlSchemaAttribute.Name = TextBox_Attribute.Text;
new_XmlSchemaAttribute.SchemaTypeName = new XmlQualifiedName(DropDownList_Attribute.SelectedValue.ToString(), <a href="http://www.w3.org/2001/XMLSchema);">http:
XmlSchemaObject new_XmlSchemaAttribute_object = (XmlSchemaObject)new_XmlSchemaAttribute;
new_XmlSchemaComplexType.Attributes.Add(new_XmlSchemaAttribute_object);
my_XmlElement.SchemaType = new_XmlSchemaComplexType;
}
else
{
XmlSchemaComplexType select_elemet_XmlSchemaComplexType = (XmlSchemaComplexType)my_XmlElement.SchemaType;
XmlSchemaAttribute new_XmlSchemaAttribute = new XmlSchemaAttribute();
new_XmlSchemaAttribute.Name = TextBox_Attribute.Text;
new_XmlSchemaAttribute.SchemaTypeName = new XmlQualifiedName(DropDownList_Attribute.SelectedValue.ToString(), <a href="http://www.w3.org/2001/XMLSchema);">http:
XmlSchemaObject new_XmlSchemaAttribute_object = (XmlSchemaObject)new_XmlSchemaAttribute;
select_elemet_XmlSchemaComplexType.Attributes.Add(new_XmlSchemaAttribute_object);
}
}
}
}
}
FileStream writer = new FileStream(Server.MapPath("person.xsd"), System.IO.FileMode.Create);
my_xmlschema.Write(writer);
writer.Close();
}
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
load_element();
}
}
Points of Interest
main idea of controling and manageing XML Schema Elements.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.