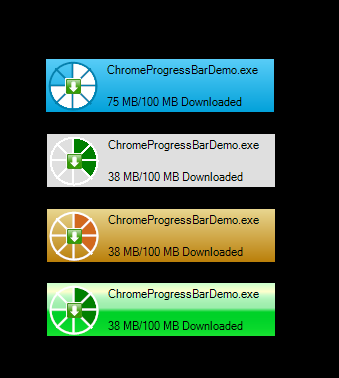
Introduction
This article demonstrates creating a Google Chrome themed ProgressBar control from scratch.
Background
Any ProgressBar control relies on a value and its graphical representation. Google Chrome downloader has a circular representation of its progress.
Using the Code
ChromeProgressBar
is designed the same way the Chrome ProgressBar works. This converts the progress values into a circular graphical representation.
private void PaintProgress(PaintEventArgs e)
{
using( SolidBrush progressBrush = new SolidBrush(this.ProgressColor))
{
Rectangle rect = LayoutInternal.ProgressRectangle;
rect.Inflate(-2, -2);
rect.Height -= 2; rect.Width -= 2;
float startAngle = -90;
float sweepAngle = Progress / 100 * 360;
e.Graphics.FillPie(progressBrush, rect, startAngle, sweepAngle);
}
}
Here is how the circle and the segments are drawn, with the graphics path and four lines. The clipping region of the Graphics
object is adjusted to clip the lines that run beyond the circle.
private void PaintBorder(PaintEventArgs e)
{
GraphicsPath borderPath = new GraphicsPath();
Rectangle progressRect = LayoutInternal.ProgressRectangle;
borderPath.AddArc(progressRect, 0, 360);
....
....
....
using (Pen borderPen = new Pen(this.BorderColor, 2))
{
e.Graphics.DrawPath(borderPen, borderPath);
e.Graphics.DrawLine(borderPen,
new Point(progressRect.Left + progressRect.Width / 2, progressRect.Top),
new Point(progressRect.Left + progressRect.Width / 2, progressRect.Bottom));
e.Graphics.DrawLine(borderPen,
new Point(progressRect.Left, progressRect.Top + progressRect.Height / 2),
new Point(progressRect.Right, progressRect.Top + progressRect.Height / 2));
e.Graphics.DrawLine(borderPen,
new Point(progressRect.Left ,progressRect.Top),
new Point(progressRect.Right,progressRect.Bottom));
e.Graphics.DrawLine(borderPen,
new Point(progressRect.Left, progressRect.Bottom),
new Point(progressRect.Right, progressRect.Top ));
}
e.Graphics.Clip = clip;
}
Points of Interest
Take a look at the InternalLayout
class to see how the control layouts individual items on the control. The nested Internal Layout class takes ChromeProgressBar
as an argument and provides all the necessary layout information required for the control leaving the painting process alone to the ChromeProgressBar
.

Hope this will you with a good start in creating controls. Please do leave your valuable comments and suggestions.