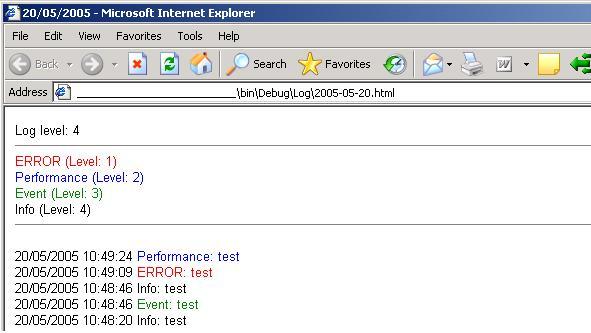
Introduction
In order to track the execution (events) of an application, log files may be useful. Usually they are text files that record the events by adding lines at the end of the file. The CHTMLLogFile
class presented in this article implements an HTML format for the log file with some (useful) features:
- The newest event is recorded first. (Using IE and F5 will always show the latest event at the top of the list without the need to scroll down.)
- Loglevel control. (User defined level for each type of entry. Only events with the right detail level will be recorded.)
- Different colors for different events/log entries.
- Log file size control. (When reaching the size limit, the file is renamed with a suffix and a new one is created with the same name so there is no need to close/open IE when file changes. Just F5 is enough.)
Background
The structure of the log file is presented below:

When adding new events to the log file, the header is assumed to be of fixed size since it is read and skipped.
The execution sequence is:
- Read existing file.
- Truncate existing file.
- Write header
- Write new event
- Parse the read file by skipping the header and add the old events to the file.
Using the code
There are two classes: LogItem
and CHTMLLogFileClass
which are part of the CHTMLLogNamespace
namespace. (Add the CHTMLLogfile.cs file to your project.)
The LogItem
class listed below implements some sort of structure with details about a specific event on the application's execution. For instance, if we want to log an error we will create a LogItem
with the following values:
Name="ERROR", Color="red", FontSize=2, Level= 1
(1 is the highest severity. The higher the level the lower the severity.)
public class LogItem : IComparable
{
public string m_strName;
public string m_strColor;
public int m_nFontSize;
public int m_nLevel;
public LogItem(string strName,string strColor, int nFontSize,int nLevel)
{
m_strName =strName;
m_strColor = strColor;
m_nFontSize = nFontSize;
m_nLevel=nLevel;
}
public Int32 CompareTo(Object obj)
{
LogItem tmpObj = (LogItem) obj;
return (this.m_strName.ToLower().CompareTo(tmpObj.m_strName.ToLower()));
}
}
Note: The CompareTo
method is not used at this point. It can be used to sort an array of LogItem
elements if needed.
Follow the guidelines below to enable and use the class in your application:
using CHTMLLogNamespace;
public class MainForm : System.Windows.Forms.Form
{
private System.ComponentModel.Container components = null;
private CHTMLLogFileClass m_logfileMain;
public MainForm()
{
InitializeComponent();
InitializeApplication();
}
private bool InitializeApplication()
{
string strPath = AppDomain.CurrentDomain.BaseDirectory+"Log\\";
string strName = System.DateTime.Now.ToString("yyyy-MM-dd")+ ".html";
m_logfileMain = new CHTMLLogFileClass(strPath+strName);
m_logfileMain.LOGLEVEL = 4;
m_logfileMain.MAXSIZE = 5;
m_logfileMain.AddHeaderItem("ERROR","red",2,1);
m_logfileMain.AddHeaderItem("Performance","blue",2,2);
m_logfileMain.AddHeaderItem("Event","green",2,3);
m_logfileMain.AddHeaderItem("Info","black",2,4);
return true;
}
}
m_logfileMain.LOGLEVEL = 4;
The log level 4 will record only events created with the 4th parameter in m_logfileMain.AddHeaderItem("Info","black",2,4);
with a value of 4 or below. A log entry created with a level of 5 will not be recorded when the LogMessage
method is invoked in the application.
Whenever there is a need to log an event, the LogMessage
method should be invoked. The 1st parameter is the name of the entry created in the code snippet above. The comparison is case insensitive so it is not important if you call the method below with "Error" or "error" for an entry type added like this:
m_logfileMain.AddHeaderItem("ERROR","red",2,1);
m_logfileMain.LogMessage("Performance","test");
m_logfileMain.LogMessage("ERROR","test");
m_logfileMain.LogMessage("Event","test");
m_logfileMain.LogMessage("Info","test");
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.