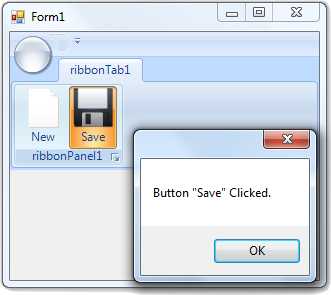
Content
Part 1: Background
The ribbon that is going to be used in this article is an open source project created by Jose Menendez Poo and SpiderMaster ( http://ribbon.codeplex.com/ ).
Main development platform: .NET Framework 2.0.
Might not compatible with .NET Framework 3.5 and 4.0.
The author has posted an article explaining what is this ribbon all about at here: A Professional Ribbon You Will Use (Now with orb!)
However, in that article doesn't describe about how to use it in your project. Therefore, this article will show how to use it.
I have posted another article explaining how to use this ribbon with MDI Client Enabled WinForm at here: Using Ribbon with MDI in WinForm Application (Update: This guide is reposted in this article as Part 4)
Part 2: How to Use This Ribbon Control
1. Download the Ribbon Source Code and obtain System.Windows.Forms.Ribbon.dll.
2. Create a blank WinForm Project.

3. Add a Reference of System.Windows.Forms.Ribbon.dll to your project.
4. Right click on Reference at the Solution Explorer, choose Add.

5. Locate the System.Windows.Forms.Ribbon.dll.

6. Open the designer of the Main Form. In this example, Form1.Designer.cs.

7. This is the initial code for Form1.Designer.cs:
namespace WindowsFormsApplication1
{
partial class Form1
{
private System.ComponentModel.IContainer components = null;
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.Text = "Form1";
}
#endregion
}
}
8. Add three lines of code into
Form1.Designer.cs.
private System.Windows.Forms.Ribbon ribbon1;
this.ribbon1 = new System.Windows.Forms.Ribbon();
this.Controls.Add(this.ribbon1);

9. Save and close
Form1.Designer.cs.
10. Double click and open
Form1.cs, and now the Ribbon control is added into the main form.


11. Click on the Ribbon and Click
Add Tab.

12. Click on the newly added
RibbonTab, then click
Add Panel.

13. Click on the newly added
RibbonPanel, go to
Properties. A set of available controls that can be added to the
RibbonPanel.

14. Try add some buttons into the
RibbonPanel.
15. Click on the
RibbonButtons, go to
Properties. Lets try to change the image and the label text of the button.

16. This is how your ribbon looks like now.
17. Now, create the click event for the buttons. Click on the
RibbonButton, go to
Properties, modify the
Name of the button.

18. Open the code of
Form1.cs.
19. This is what we have initially:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
}
}
20. Add the
Button Clicked event for the
RibbonButton.
public Form1()
{
InitializeComponent();
cmdNew.Click += new EventHandler(cmdNew_Click);
cmdSave.Click += new EventHandler(cmdSave_Click);
}
void cmdNew_Click(object sender, EventArgs e)
{
MessageBox.Show("Button \"New\" Clicked.");
}
void cmdSave_Click(object sender, EventArgs e)
{
MessageBox.Show("Button \"Save\" Clicked.");
}
21. Press
F5 to run the application. Done.

22. You might want to inherit your
Main Form into a
RibbonForm to have extra feature. Such as:
Note: Inherit the
Main Form to
RibbonForm will have some compatible problems with some of
System.Windows.Forms controls.

23. At the code of
Form1.cs, change this line:
public partial class Form1 : Form
to this line:
public partial class Form1 : RibbonForm
A sample project (RibbonSample.zip) that used to explained in this article is downloadable at top.
Part 3: Cautious While Using With Visual Studio 2010
ALWAYS SAVE AND CLOSE straight away after you have finished designing the GUI editing of
Main Form (The form that contains the ribbon control).
Don't Run (Press F5) The Application while the
Main Form is open in
Visual Studio 2010.
Or else, you might experience that the ribbon control has disappeared. You will end up redesigning/redraw the ribbon and reconnect all the events that associated with the ribbon.
Part 4: Using This Ribbon with MDI Enabled WinForm

The following guide will show how to apply this ribbon with MDI (Multi Document Interface) enabled WinForm.
Simple Walkthrough
I'll briefly explain what are we going to do here.
The original System.Windows.Forms.Ribbon.dll obtained from the source code is not fully compatible with System.Windows.Forms's MDI Client. You'll experience a delay while closing forms within MDI Client area. Therefore, we have to make some modification on the DLL so that is will enable us to use the Ribbon with MDI fluently. A sample project which describe in this article can be downloaded at top. You may download it, view the code to have a better understanding on the work flow of implementing MDI with this ribbon control.
This guide will also show you how eliminate the control box (highlighted in the below picture) when a MDI child form is Maximize within the MDI Client control of the main form. This will help to make the appearance of the application will look less weird.

Start
1. Start off by modifying the original System.Windows.Forms.Ribbon.dll.
2. Download the source code of the ribbon. Open GlobalHook.cs.

3. Find private void InstallHook(). Add a return statement at the beggining of the method so nothing is done.

4. Build the solution and obtain the newly edited
System.Windows.Forms.Ribbon.dll. We'll use this to build our next MDI WinForm. Or, you can simply download it (which I've modified) at the top of this page.
Add this edited
System.Windows.Forms.Ribbon.dll as reference and start building the application.
5. Lets first create a Ribbon application with the edited
System.Windows.Forms.Ribbon.dll like this. Don't inherit the
MainForm (The form that contain ribbon control) with
RibbonForm. Inheritance of
RibbonForm is not compatible with
MDI Client Control.

Create the
Click Event for the Ribbon Buttons.
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
cmdCloseForm.Click += new EventHandler(cmdCloseForm_Click);
cmdForm1.Click += new EventHandler(cmdForm1_Click);
cmdForm2.Click += new EventHandler(cmdForm2_Click);
cmdWelcome.Click += new EventHandler(cmdWelcome_Click);
}
void cmdWelcome_Click(object sender, EventArgs e)
{
}
void cmdForm2_Click(object sender, EventArgs e)
{
}
void cmdForm1_Click(object sender, EventArgs e)
{
}
void cmdCloseForm_Click(object sender, EventArgs e)
{
}
}
6. Next, set the
MainForm's properties of
IsMdiContainer to
True.

7. Create a few Forms that to be opened at
MainForm's MDI. You can name it anything, of course, but we take these as example:
- Form1.cs
- Form2.cs
- WelcomeForm.cs
and the codes we use to open the forms in MDI might look like this:
void cmdForm1_Click(object sender, EventArgs e)
{
Form1 f1 = new Form1();
f1.MdiParent = this;
f1.ControlBox = false;
f1.MaximizeBox = false;
f1.MinimizeBox = false;
f1.WindowState = FormWindowState.Maximized;
f1.Show();
}
8. This forms run normally, but you will notice there is a annoying
Control Box appear at the top of Ribbon Bar control.

9. To get rid of the
Control Box, we need to rearrange these codes in the correct sequence.
f1.ControlBox = false;
f1.FormBorderStyle = System.Windows.Forms.FormBorderStyle.None
f1.MaximizeBox = false;
f1.MinimizeBox = false;
f1.WindowState = FormWindowState.Maximized;
10. First, we create another form named
MdiChildForm.cs. Open the designer of
MdiChildForm.

11. Add the below code to
MdiChildForm.Designer.cs at the right sequence:
this.WindowState = System.Windows.Forms.FormWindowState.Normal;
this.ControlBox = false;
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.None;
this.MaximizeBox = false;
this.MinimizeBox = false;

at the
Load event of
MdiChildForm, add this:
public partial class MdiChildForm : Form
{
public MdiChildForm()
{
InitializeComponent();
this.Load += new System.EventHandler(this.MdiChildForm_Load);
}
private void MdiChildForm_Load(object sender, EventArgs e)
{
this.ControlBox = false;
this.WindowState = FormWindowState.Maximized;
this.BringToFront();
}
}
12. Save and Close
MdiChildForm.cs and
MdiChildForm.Designer.cs.
13. Modify all forms (forms that will be load at
MainForm.cs's MDI) to inherit
MdiChildForm.
Form1.csChange this:
public partial class Form1 : Form
to this:
public partial class Form1 : MdiChildForm
Form2.csChange this:
public partial class Form2: Form
to this:
public partial class Form2: MdiChildForm
WelcomForm.csChange this:
public partial class WelcomForm: Form
to this:
public partial class WelcomForm: MdiChildForm
14. Open forms and load it into the MDI Client of
MainForm.
void cmdForm1_Click(object sender, EventArgs e)
{
Form1 f1 = new Form1();
f1.MdiParent = this;
f1.Show();
}
15.
Done. A sample project
(RibbonMdiSample.zip) that used in this article can be downloaded at top.
