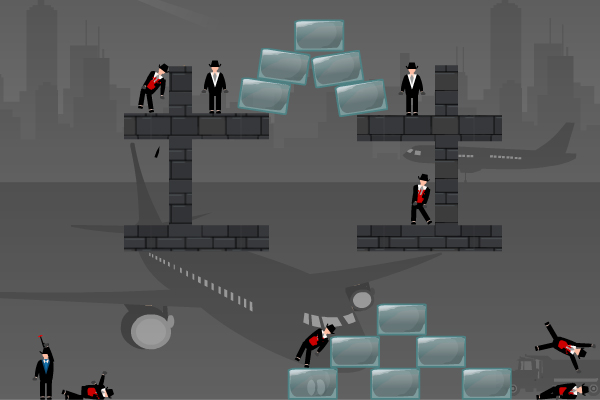
Introduction
Killer Instinct is a physics based shooting game. The objective is to perfectly shoot the enemies. The game consists of a character holding a gun and the enemies standing at their positions. The obstacles can stop the hero's bullets or fall over the hero. This game is written in AS3 using physics game engine called Box2D.
- Fully playable with mouse, and touch.
- Bullets are projected from the gun with certain speed and it repels when it hits to any physics bodies or walls.
- Hero and enemy characters behaves as rag-dolls.
Background
Interest towards shooting games made me think of this. We planned to add physics to make the game interesting.
Using the Code
The back end of the game is built in AS3, making use of physics based engine Box2D. The main aspect of the game is to move the bullet.
public function BulletActor(parent,xValue,yValue,rotation,vx,vy)
{
skin = new Arrow();
skin.x = xValue;
skin.y = yValue;
skin.rotation = rotation*180/Math.PI;
parent.addChild(skin);
prevX = skin.x;
prevY = skin.y;
var shape:b2PolygonShape = new b2PolygonShape();
shape.SetAsBox(6 / Phyvalues.RATIO, 1.4 / Phyvalues.RATIO);
var fixtureDef:b2FixtureDef= new b2FixtureDef();
fixtureDef.density = 20;
fixtureDef.restitution = 1;
fixtureDef.friction = 0;
fixtureDef.userData = skin;
fixtureDef.userData.remove = remove;
fixtureDef.userData.prevX = prevX;
fixtureDef.userData.prevY = prevY;
fixtureDef.userData.count = count;
fixtureDef.shape = shape;
fixtureDef.filter.groupIndex = -1;
var bodyDef:b2BodyDef = new b2BodyDef();
bodyDef.bullet = true;
bodyDef.angle = rotation;
bodyDef.type = b2Body.b2_dynamicBody;
bodyDef.position.Set(xValue / Phyvalues.RATIO, yValue / Phyvalues.RATIO);
bodyDef.fixedRotation = true;
body = Phyvalues._world.CreateBody(bodyDef);
body.CreateFixture(fixtureDef);
body.SetLinearVelocity(new b2Vec2(vx, vy));
body.SetBullet(true);
body.applyGravity = false;
super(body, skin);
}
Bullet Movement:
private function loop(e:Event):void
{
if (_costume is Bullet) {
var dx = _costume.x - _costume.prevX;
var dy = _costume.y - _costume.prevY;
var ang = Math.atan2(dy, dx);
_body.SetAngle(ang);
_costume.prevX = _costume.x;
_costume.prevY = _costume.y;
_costume.remove++;
var force:b2Vec2 = _body.GetLinearVelocity();
_body.ApplyForce(force, _body.GetWorldCenter());
}
_costume.x = _body.GetPosition().x * Phyvalues.RATIO;
_costume.y = _body.GetPosition().y * Phyvalues.RATIO;
_costume.rotation = _body.GetAngle() * 180 / Math.PI;
}
var xpt = Phyvalues._allActors[i].xv;
var ypt = Phyvalues._allActors[i].yv;
var vx = Phyvalues._allActors[i].velocity.x;
var vy = Phyvalues._allActors[i].velocity.y;
ragDolls = new RagDolls1(xpt, ypt);
ragDolls.body.ApplyImpulse(new b2Vec2(vx, vy), ragDolls.body.GetWorldCenter());
...
Points of Interest
Creation of ragdolls and their movement.
History
- 22nd October, 2012: Initial version
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.