Introduction
This article shows how to create a shortcut file (.lnk) to your application in the Windows Startup folder (or any other folder). This is useful if you want your application to be started when a user logs in to Windows. The sample code also shows how to read the target file of a shortcut in order to determine if it points to your application. This is useful when you want to delete existing shortcuts to your application.
Using the code in this article, you will be able to add a checkbox with the label 'Start this application when Windows is started' to your application.
Starting your application on login by means of a Startup folder shortcut is more user friendly than starting the application as a service. It makes it visible to the user that the application is started on login, as opposed to the many update managers (from Adobe, Google, Apple) that are started in the background and can only be disabled by special tools like msconfig.
Background
There is no direct API in the .NET Framework for creating and editing shortcut files in Windows. You will have to use COM APIs exposed by the Windows Shell and Windows Script Host. Also, the shortcut file format is not documented by Microsoft.
The article shows how to create and read shortcut files from a C# application using COM interop.
Using the code
The sample code is a simple Windows Forms application with two buttons: Create Shortcut and Delete Shortcuts that allows you to test the functionality. The sample application is a Visual Studio 2010 solution.
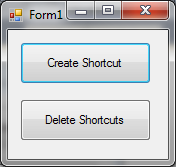
The first thing you need to do is to add two references to your project. Select Add Reference, select the COM tab, and select these two components:
- Microsoft Shell Controls and Automation
- Windows Script Host Object Model
Click OK, and you will have two new references highlighted below:
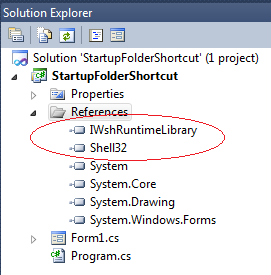
For each of these new references, open their Properties window in Visual Studio and change the property Embed Interop Types from True to False.
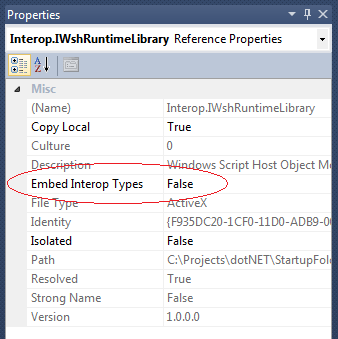
If you don't do this, you will get the compiler error: Interop type 'IWshRuntimeLibrary.WshShellClass' cannot be embedded. Use the applicable interface instead., when using the .NET 4.0 framework.
In the C# source code, we add these two using
s:
using IWshRuntimeLibrary;
using Shell32;
The code that creates a shortcut file in the Startup Folder is shown below:
public void CreateStartupFolderShortcut()
{
WshShellClass wshShell = new WshShellClass();
IWshRuntimeLibrary.IWshShortcut shortcut;
string startUpFolderPath =
Environment.GetFolderPath(Environment.SpecialFolder.Startup);
shortcut =
(IWshRuntimeLibrary.IWshShortcut)wshShell.CreateShortcut(
startUpFolderPath + "\\" +
Application.ProductName + ".lnk");
shortcut.TargetPath = Application.ExecutablePath;
shortcut.WorkingDirectory = Application.StartupPath;
shortcut.Description = "Launch My Application";
shortcut.Save();
}
We are using the WshShellClass
class to create a shortcut. The shortcut TargetPath
is taken from the global Application
object.
In order to determine if an existing shortcut file is pointing to our application, we need to be able to read the TargetPath
from a shortcut file. This is done using the code below:
public string GetShortcutTargetFile(string shortcutFilename)
{
string pathOnly = Path.GetDirectoryName(shortcutFilename);
string filenameOnly = Path.GetFileName(shortcutFilename);
Shell32.Shell shell = new Shell32.ShellClass();
Shell32.Folder folder = shell.NameSpace(pathOnly);
Shell32.FolderItem folderItem = folder.ParseName(filenameOnly);
if (folderItem != null)
{
Shell32.ShellLinkObject link =
(Shell32.ShellLinkObject)folderItem.GetLink;
return link.Path;
}
return String.Empty;
}
The code that searches for, and deletes, existing shortcuts to our application is shown below:
public void DeleteStartupFolderShortcuts(string targetExeName)
{
string startUpFolderPath =
Environment.GetFolderPath(Environment.SpecialFolder.Startup);
DirectoryInfo di = new DirectoryInfo(startUpFolderPath);
FileInfo[] files = di.GetFiles("*.lnk");
foreach (FileInfo fi in files)
{
string shortcutTargetFile = GetShortcutTargetFile(fi.FullName);
if (shortcutTargetFile.EndsWith(targetExeName,
StringComparison.InvariantCultureIgnoreCase))
{
System.IO.File.Delete(fi.FullName);
}
}
}
Using these methods in your application, you can now implement the code behind a checkbox with the label: Start application when Windows is started.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.