Overview
ASP.NET provides the MVC framework as an alternate to the ASP.NET Web Forms pattern for developing web applications. MVC (Model-View-Controller) is a lightweight, highly testable presentation framework that is integrated with the existing ASP.NET features such as Master Pages and Membership-based authentication.
Introduction
The System.Web.Mvc
namespace is for ASP.NET MVC. MVC is a standard Design Pattern. MVC has the following components:
- Controller
Classes that handle incoming requests to the application, retrieve model data, and then specify view templates that return a response to the client.
- Model
Classes that represent the data of the application and that use validation logic to enforce business rules for that data.
- View
Template files that your application uses to dynamically generate HTML responses.
Background
In this demonstration, I will design a database using SQL Server Management Studio 2005. I will develop an ASP.NET MVC application and will describe all of its parts.
Let’s Get Started
Open SQL Management Studio 2005. Crate a database and name it Inventory. Design a database table like below:
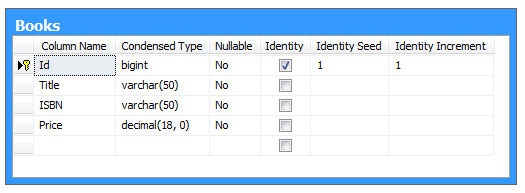
Figure 1
- Open Microsoft Visual Studio 2010
- Create a new project (File> New> Project> Visual C#): ASP.NET MVC 3 Web Application
- Named it ASPNETMVCApplication
- Click on the OK button

Figure 2
- Select a template for Internet Application
- View engine to Razor
- Create a unit test project to uncheck
- Click on the OK button

Figure 3
The default project will be created. Now Add Library Package Reference. We will use a .NET Framework data-access technology known as the Entity Framework. The Entity Framework (often referred to as “EF”) supports a development paradigm called code-first. Code-first allows you to create model objects by writing simple classes. You can then have the database created on the fly from your classes, which enables a very clean and rapid development workflow.
We'll start by using the NuGet package manager (automatically installed by ASP.NET MVC 3) to add the EFCodeFirst library to the ASPNETMVCApplication project. This library lets us use the code-first approach. To add Entity Framework reference:
From the Tools menu, select Library Package Manager and then Add Library Package Reference.

Figure 4
In the Add Library Package Reference window, click online.

Figure 5
Click the NuGet Official package source.

Figure 6
Find EFCodeFirst, click on install.

Figure 7
Click on I Accept. Entity Framework will install and will add a reference to the project.

Figure 8
Click on the Close button.

Figure 9
Adding a Model Class
- Right click on Models from Solution Explorer
- Select Add
- Select Class
- Name it Book.cs

Figure 10

Figure 11
Add the following properties in the Book.cs file:
namespace ASPNETMVCApplication.Models
{
public class Book
{
public Int64 Id { get; set; }
public String Title { get; set; }
public String ISBN { get; set; }
public Decimal Price { get; set; }
}
}
Add another class the way you did for Book.cs and name it BookDBContext.cs. Add a System.Data.Entity
namespace reference in the BookDBContext.cs file.
using System.Data.Entity;
Add the following code in the BookDBContext
class. And derive it from the DbContext
class so that all data operations can be handled by the Books
property.
Note: The property name Books
must be the same as the database table name Books.
Here is the complete code:
using System;
using System.Web;
using System.Data.Entity;
namespace ASPNETMVCApplication.Models
{
public class BookDBContext:DbContext
{
public DbSet<Book> Books { get; set; }
}
}
Add a connection string in the web.config file. Remember, the connection string name must be the same as BookDBContext
.
<connectionStrings>
<add name="BookDBContext"
connectionString="data source=Home-PC;
uid=sa;pwd=1234;Initial Catalog=Inventory"
providerName="System.Data.SqlClient" />
</connectionStrings>
Adding the Controller
- Right click on Controller from Solution Explorer
- Select Add
- Select Controller
- Name it BookController
- Add action methods for Create, Update, Delete, and Details scenarios to Checked

Figure 12

Figure 13
It will add the following code:
namespace ASPNETMVCApplication.Controllers
{
public class BookController : Controller
{
public ActionResult Index()
{
return View();
}
public ActionResult Details(int id)
{
return View();
}
public ActionResult Create()
{
return View();
}
[HttpPost]
public ActionResult Create(FormCollection collection)
{
try
{
return RedirectToAction("Index");
}
catch
{
return View();
}
}
public ActionResult Edit(int id)
{
return View();
}
[HttpPost]
public ActionResult Edit(int id, FormCollection collection)
{
try
{
return RedirectToAction("Index");
}
catch
{
return View();
}
}
public ActionResult Delete(int id)
{
return View();
}
[HttpPost]
public ActionResult Delete(int id, FormCollection collection)
{
try
{
return RedirectToAction("Index");
}
catch
{
return View();
}
}
}
}
Now add the following namespaces in the BookController.cs file:
using System.Linq;
using ASPNETMVCApplication.Models;
Declare a global instance variable of the BookDBContext
class in the BookController
class.
BookDBContext _db = new BookDBContext();
Add the following code in the Index()
method:
public ActionResult Index()
{
var books = from book in _db.Books
select book;
return View(books.ToList());
}
This is the LINQ code for getting books and books.ToList()
to display the book list in the View.
Now right click on Solution and select Build to build the solution.
Adding the View
- Right click in the Index method
- Select View
- Create a strongly-typed view to Checked
- Select Model class to Book (ASPNETMVCApplication.Models)
- Scaffold template to List
- Click on the OK button

Figure 14
It will add the Index.cshtml file.
@model IEnumerable<ASPNETMVCApplication.Models.Book>
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
<p>
@Html.ActionLink("Create New", "Create")
</p>
<table>
<tr>
<th></th>
<th>
Title
</th>
<th>
ISBN
</th>
<th>
Price
</th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.ActionLink("Edit", "Edit", new { id=item.Id }) |
@Html.ActionLink("Details", "Details", new { id=item.Id }) |
@Html.ActionLink("Delete", "Delete", new { id=item.Id })
</td>
<td>
@item.Title
</td>
<td>
@item.ISBN
</td>
<td>
@String.Format("{0:F}", item.Price)
</td>
</tr>
}
</table>
Now, add the Create View.
Adding the Create View
- Right click on the Create method
- Select View
- Create a strongly-typed view to Checked
- Select Model class to Book (ASPNETMVCApplication.Models)
- Scaffold template to Create
- Click on the OK button
It will add the Create.cshtml file.
@model ASPNETMVCApplication.Models.Book
@{
ViewBag.Title = "Create";
}
<h2>Create</h2><script src="@Url.Content("~/Scripts/jquery.validate.min.js")"
type="text/javascript"></script><script
src="@Url.Content("~/Scripts/jquery.validate.unobtrusive.min.js")"
type="text/javascript"></script>@using (Html.BeginForm()) {
@Html.ValidationSummary(true)
<fieldset>
<legend>Book</legend><div class="editor-label">
@Html.LabelFor(model => model.Title)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.Title)
@Html.ValidationMessageFor(model => model.Title)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.ISBN)
</div>
<div class="editor-field">
@Html.EditorFor(model =>
Now add the following code in the Create
method in the BookController
class.
[HttpPost]
public ActionResult Create(FormCollection collection)
{
try
{
Book book = new Book();
if (ModelState.IsValid)
{
book.Title = collection["Title"].ToString();
book.ISBN = collection["ISBN"].ToString();
book.Price = Convert.ToDecimal(collection["Price"]);
_db.Books.Add(book);
_db.SaveChanges();
return RedirectToAction("Index");
}
else
{
return View(book);
}
}
catch
{
return View();
}
}
Now we will add the Edit View.
Adding the Edit View
- Right click on the Edit method
- Select View
- Create a strongly-typed view to Checked
- Select Model class to Book (ASPNETMVCApplication.Models)
- Scaffold template to Edit
- Click on the OK button

Figure 15
It will add the Edit.cshtml file:
@model ASPNETMVCApplication.Models.Book
@{
ViewBag.Title = "Edit";
}
<h2>Edit</h2><script src="@Url.Content("~/Scripts/jquery.validate.min.js")"
type="text/javascript"></script><script
src="@Url.Content("~/Scripts/jquery.validate.unobtrusive.min.js")"
type="text/javascript"></script>@using (Html.BeginForm()) {
@Html.ValidationSummary(true)
<fieldset>
<legend>Book</legend>@Html.HiddenFor(model => model.Id)
<div class="editor-label">
@Html.LabelFor(model => model.Title)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.Title)
@Html.ValidationMessageFor(model => model.Title)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.ISBN)
</div>
<div class="editor-field">
@Html.EditorFor(model =>
Now add the following code in the Edit
method in the BookController
class.
public ActionResult Edit(int id)
{
Book book = _db.Books.Find(id);
if (book == null)
return RedirectToAction("Index");
return View(book);
}
[HttpPost]
public ActionResult Edit(int id, FormCollection collection)
{
try
{
var book = _db.Books.Find(id);
UpdateModel(book);
_db.SaveChanges();
return RedirectToAction("Index");
}
catch
{
ModelState.AddModelError("", "Edit Failure, see inner exception");
return View();
}
}
Now we will add the Details View.
Adding the Details View
- Right click on the Edit method
- Select View
- Create a strongly-typed view to Checked
- Select Model class to Book (ASPNETMVCApplication.Models)
- Scaffold template to Details
- Click on the OK button

Figure 16
It will add the Details.cshtml file:
@model ASPNETMVCApplication.Models.Book
@{
ViewBag.Title = "Details";
}
<h2>Details</h2><fieldset>
<legend>Book</legend><div class="display-label">Title</div>
<div class="display-field">@Model.Title</div>
<div class="display-label">ISBN</div>
<div class="display-field">@Model.ISBN</div>
<div class="display-label">Price</div>
<div class="display-field">@String.Format("{0:F}", Model.Price)</div>
</fieldset>
<p>
@Html.ActionLink("Edit", "Edit", new { id=Model.Id }) |
@Html.ActionLink("Back to List", "Index")
</p>
Add the following code to the Details
method of the BookController
class.
public ActionResult Details(int id)
{
Book book = _db.Books.Find(id);
if (book == null)
return RedirectToAction("Index");
return View("Details", book);
}
Now we will add the Delete View.
Adding the Delete View
- Right click on the Edit method
- Select View
- Create a strongly-typed view to Checked
- Select Model class to Book (ASPNETMVCApplication.Models)
- Scaffold template to Delete
- Click on the OK button

Figure 17
It will add the Delete.cshtml file.
@model ASPNETMVCApplication.Models.Book
@{
ViewBag.Title = "Delete";
}
<h2>Delete</h2><h3>Are you sure you want to delete this?</h3><fieldset>
<legend>Book</legend><div class="display-label">Title</div>
<div class="display-field">@Model.Title</div>
<div class="display-label">ISBN</div>
<div class="display-field">@Model.ISBN</div>
<div class="display-label">Price</div>
<div class="display-field">@String.Format("{0:F}", Model.Price)</div>
</fieldset>
@using (Html.BeginForm()) {
<p>
<input type="submit" value="Delete" /> |
@Html.ActionLink("Back to List", "Index")
</p>
}
Add the following code to the Delete
method in the BookController
class.
public ActionResult Delete(int id)
{
Book book = _db.Books.Find(id);
if (book == null)
return RedirectToAction("Index");
return View(book);
}
[HttpPost]
public ActionResult Delete(int id, FormCollection collection)
{
Book book = _db.Books.Find(id);
_db.Books.Remove(book);
_db.SaveChanges();
return RedirectToAction("Index");
}
Now run the application. Type /Book in the URL and remember that the port number may be different in your case.

Figure 17
Click Create New Link to add a new book.

Figure 18

Figure 19
This way you can edit, delete, and view details of the books.
Conclusion
This article will help you create your own robust ASP.NET MVC application.
Thank you!
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.