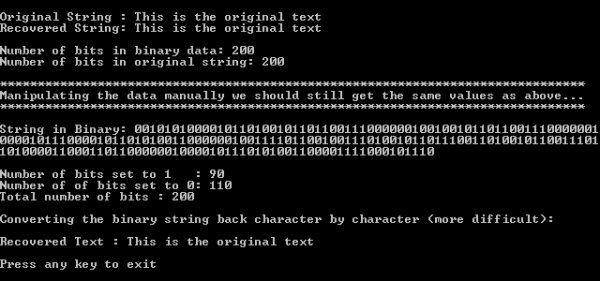
Introduction
This article looks at the conversion of a string to a binary stream and the conversion of a BitArray
of binary data to an ASCII string. The .NET Framework does not provide a method for constructing a byte of data by explicitly specifying each bit. This makes it difficult to convert a string containing binary data back into ASCII encoded data, which is a useful exercise in some debugging scenarios.
Using the code
Let us first look at converting a string into binary. We do this using the MemoryStream
and BinaryWriter
objects. The BinaryWriter
object allows us to write a stream of binary data for a given string. As we are not sending the binary data anywhere (in this example), we will use a MemoryStream
object to provide us with a stream to use.
MemoryStream ms_memStream = new MemoryStream();
BinaryWriter br_binaryWriter = new BinaryWriter(ms_memStream);
try
{
br_binaryWriter.Write(ba_originalString);
}
catch (Exception e)
{
System.Console.WriteLine("Exception writing binary" +
" data to memory stream: " +
e.Message);
Environment.Exit(1);
}
The BinaryWriter.Write(String str)
method converts the string parameter into a stream of binary data that is stored in the associated stream object, in this case our MemoryStream
object. It is important to note that because the data has been serialized, the bytes of data within the binary stream no longer have the most significant bit on the left, so the letter "T" would be 00101010 (ASCII 84) instead of 01010100. It is very important to bear this in mind when reading the data later, as, if we do not account for this, we will not read the value correctly. In this case, we would interpret the data as ASCII value 42, which is not the letter "T".
Converting a binary stream back into ASCII can be done in several ways depending upon what form we have the binary data in. The simplest method is where we have the binary data as a stream, as shown above. This method is useful if the binary data is being read from a file. To do this, we simply use the following code:
String str_recoveredString =
Encoding.ASCII.GetString(ms_memStream.ToArray());
If we want to look at the bits in the stream one by one, we must convert the binary data into a BitArray
object, as shown here:
BitArray ba_bitArray = new BitArray(ms_memStream.ToArray());
N.B. The need for this was encountered by the author by using a stream of binary data for encryption purposes. This required looking at individual bits in a key string to generate a unique salt from it.
Once a binary stream is encapsulated by a BitArray
object, it becomes slightly more difficult to manipulate. None of the traditional conversion/parsing methods work, as a BitArray
object only returns values as Boolean
s. Furthermore, the .NET Framework does not provide a method for creating a byte
object by explicitly setting the values of each bit within it. Thus, to convert binary data within a BitArray
object back into ASCII, we must calculate the decimal values from the binary data, as shown here:
private static string recoverText(BitArray ba_bitArray)
{
string str_finalString = "";
int int_binaryValue;
BitArray ba_tempBitArray;
while (ba_bitArray.Length > 0)
{
ba_tempBitArray = new BitArray(ba_bitArray.Length - 8);
int_binaryValue = 0;
if (ba_bitArray[0])int_binaryValue += 1;
if (ba_bitArray[1]) int_binaryValue += 2;
if (ba_bitArray[2]) int_binaryValue += 4;
if (ba_bitArray[3]) int_binaryValue += 8;
if (ba_bitArray[4]) int_binaryValue += 16;
if (ba_bitArray[5]) int_binaryValue += 32;
if (ba_bitArray[6]) int_binaryValue += 64;
if (ba_bitArray[7]) int_binaryValue += 128;
str_finalString += Char.ConvertFromUtf32(int_binaryValue);
int int_counter = 0;
for (int i = 8; i < ba_bitArray.Length; i++)
{
ba_tempBitArray[int_counter++] = ba_bitArray[i];
}
ba_bitArray = ba_tempBitArray;
}
return str_finalString;
}
Here we are simply converting 8 bits of binary to decimal and using the Char.ConvertFromUtf32(int i)
method to return a String
object of the appropriate ASCII value. We do this for each block of eight bits in the BitArray
and then return the string.
History
- Initially written: 10th October 2006.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.