ASP.NET User Controls - Notify One Control Of Changes In Other Control






4.39/5 (20 votes)
Mar 13, 2002
6 min read

197866

2615
A tutorial on how to use delegate model to notify one ASP.NET user control of changes in the other user control.
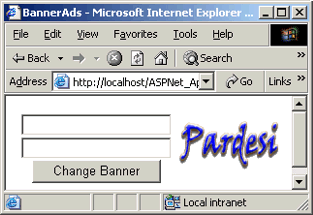
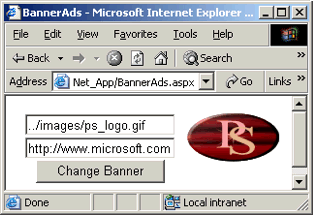
In our previous two articles, User Controls - I and User Controls - II we discussed some basic concepts involved in writing some simple ASP.Net UserControls
. In continuing further, we have tried to take a step further and extend the previously discussed concepts to write a pair of controls that cmunicate with each other using delegate
mechanism.
What Does The Sample Do
To demostrate the concept of two communicating controls, we wrote two simple controls. One control, SiteBanner
, displays an advertisement banner and the other control, SiteBannerManager
, changes the banner's image URL and link URL. When you click on button in SiteBannerManager
control, it raises an event and SiteBanner
control responds to it and changes the URLs.
How Do We Do It
As we said, one control raises the event and the other responds to it. How does this communication take place? In the f.NET Framework, handling and raising of events takes place through delegate
mechanism. We will not be discussing this mechanisn in detail. There is a good explanation of the delegate
mechanism along with a nice and explnatory sample.
The two controls involved in this mechanism plays the following roles.
SiteBannerManager
- Event RaiserSiteBanner
- Event Consumer
The following is sequence of events take plave for this communication to take place.
- The event source,
SiteBannerManager
, responds toClick
event of a button on the control. - In the event handler of this button contol a
BannerChangeEventArgs
object is constructed that contains the new image and link URL information. SiteBannerManager
control then raisesBannerChange
event.- The event consumer,
SiteBanner
, control registers itself withSiteBannerManager
control so that it gets informed when changes take place. - When
BannerChange
event is raised bySiteBannerManager
control, the consumer control recieves the notification through the delegate that it registered with the event raiser control. - In response to event notification,
SiteBanner
control extracts the new link information fromBannerChangeEventArgs
object and assigns the new values to itsasp:HyperLink
control. - And the refeshed page shows the new banner with new navigation link.
Implementation Details
So far so good. Lets discuss how the implementation of this whole event flow mechanism has been accomplished. For event/delegate
mechanism is very well described in .NET Framework documentation. The trick is how to extend it for your requirements. We will try to explain in the same sequence as is there in the documentation so that when you read the documentation it makes sense.
SiteBannerManager
- Event Raiser
First we will write the control that will raise the event communicating that image and navigation URLs have changed.
The geenral definition of an event handler is as follows.
public void MyEventHandler(object sender, MyEventArgs args);
The second argument of the event handler encapsulates the data specific to the event that is being raised. So the first step is to decide what information needs to be passed with the event. In our case, we want to notify all the client of new image and navigation URL. We create BannerChangeEventArgs
class, derived from System.EventArgs
class. The class has two properties storing the values of two URL strings.
public class BannerChangeEventArgs : EventArgs
Then provide the definition of event handler.
public delegate void BannerChangeEventHandler(object sender,
BannerChangeEventArgs e);
Here is the important part. Add an instance of this event handler in SiteBannerManager
class. This instance will provide the connection point for the consumers where they will register their event handlers. And also provide the function that will raise the event.
public abstract class SiteBannerManager : System.Web.UI.UserControl
{
.
.
public event BannerChangeEventHandler BannerChange;
protected void OnChangeBannerImg(object sender, System.EventArgs e)
{
BannerChangeEventArgs args = new BannerChangeEventArgs();
args.ImageURL = wndImgURLTxt.Text;
args.LinkURL = wndLinkURLTxt.Text;
OnBannerChange(args);
}
protected virtual void OnBannerChange(BannerChangeEventArgs args)
{
if (BannerChange != null)
{
BannerChange(this, args);
}
}
}
The above code provides the overview of code that needs to be provided for raising the event. OnChangeBannerImg
is the event handler for Click
event of the button on the control. When user clicks on the button, this event is handled. We extract the values of new URL string s from two asp:Textbox
controls and stuff them into BannerChangeEventArgs
object. Then the control raises ther event for all the delegates that are registered for handling the event.
SiteBanner
- Event Consumer
Event raiser alone cannot accomplish anything unless there is a consumer that will act upon the event raised by it. If there is no event handler registered with SiteBannerManager
object's event handler, the changes to the URLs amde in that control will not make any effect on the page.
So we need to write a consumer that will handle BannerChange
events. This is done in SiteBanner
class. Following steps are followed to implement event handling.
The foremost responsibility of the event consumer is to provide the event handling function. This function has to have the same signatures as the event handler defined by the event raiser. Here is the implementation of the event handler in SiteBanner
class.
public void ChangeBannerImage(object sender, BannerChangeEventArgs args)
{
if (args.ImageURL.Length != 0 &&
args.LinkURL.Length != 0)
{
BannerHyperLink.ImageUrl = args.ImageURL;
BannerHyperLink.NavigateUrl = args.LinkURL;
}
}
The arguments of this event handler function are the same as delegate defined in event raiser class SiteBannerManager
.
Putting It All Together
Now we have a class that raises the event and a class that will handle the raised events. Lets see how the whole thing gets wired up for real action.
We have put together a very simple web form, BannerAds.aspx
. It does nothing fancy. It provides a place holder for adding the two controls that we have created. As discussed in previous articles, first we add the reference to two UserControls
at the top of the page.
<%@ Page language="c#" Codebehind="BannerAds.aspx.cs"
AutoEventWireup="false" Inherits="ASPNet_App.BannerAds" %>
<%@ Reference Control="./Controls/SiteBanner.ascx"%>
<%@ Reference Control="./Controls/SiteBannerControl.ascx"%>
Then we provide a place holder for adding the controls.
<form id="BannerAds" method="post" runat="server">
<asp:Panel Runat="server" ID="BannerDiv"></asp:Panel>
</form>
Notice that we have put the asp:Panel
placeholder control inside form
tag. It is essential to do so because we have asp:Textbox
and asp:Button
controls in the SiteBannerManager
control. To handle the events raised by these controls it is necessary that they are defined inside form
tag. If you forget to do so, don't worry. You will get a run time exception, reminding you to do so.
Now comes the real wiring up of all the pieces. First we load two controls using LoadControl
method in Page_Load
method of BannerAds
class. And then we register ChangeBannerImage
method as the event handler with BannerChange
event of SiteBannerManager
class. Following is the implementation of Page_Load
in BannerAds
class, showing how this wiring up of both controls take place.
Control bannerControl;
Control banner;
try
{
bannerControl = LoadControl("Controls/SiteBannerControl.ascx");
banner = LoadControl("Controls/SiteBanner.ascx");
if (bannerControl != null)
{
((SiteBannerControl)bannerControl).BannerChange +=
new BannerChangeEventHandler(((SiteBanner)banner).ChangeBannerImage);
}
CreateBannerControlTable(bannerControl, banner);
}
And now we are ready to see this whole thing in action. Bring up the BannerAds.aspx page. It will show you two text boxes and a button on left side and a banner on right side. Type in new URL for new image and navigation and click on "Change Banner" button. You will see that banner image changes on right side, provided the URL points to valid image file.
We haven't added validators to the text boxes making sure that strings are not empty. We will update the code as we along with this series on UserControl
topic. But we are sure that it should give you a good idea on how you can make user controls respond to events raised by the other control.
In the coming days we will be posting more articles demonstrating some more aspects of UserControl
development. So If you have any suggestions or would like to some feature to be demonstrated, please feel free to write us at softomatix@pardesiservices.com or contact us at Softomatix.