Wizard Control Installation
First of all you must install Utility Library. Utility Library contains many
controls one from them is a Wizard Control. So let’s step by step go throw the
process of controls installation. First of all you must compile Utility Library
in Debug or Release mode (if you want to well understand how controls works then
build library in Debug mode, then you can set break points in it and view class
members values and etc.). Second Step is to add Utility Library controls to IDE
toolbox. So open any form in design mode. Open Toolbox. And call context menu of
toolbox (right mouse button can help :). You must saw something like on picture
below.
NOTE:
After first iteration of wizard implementation many things changed and now
wizard designers used by IDE are in stand alone DLL file:
UtilityLibrary.Designers.dll
. Also Windows API exported functions are also in
stand alone DLL: UtilityLibrary.Win32.WindowsAPI.dll
. To install designers of
Wizard you must do such steps:
- Compile all library DLLs
- Register in GAC UtilityLibrary.Designers.dll
- Close IDE and start it again
- After that wizard control must work fine.
- If you include library into own project then uncheck Designer library
after first build to make say IDE to skip sub-project build. Use Configuration
manger for such purposes.
Known problems:
- IDE forgot how to show Wizards sometimes. They show wizard form, but
buttons Next and Previous does not work. Restart of IDE can help. In such
error mode Pages of Wizard can be switch by wizard control Property:
PageIndex.
Select in context menu Add Tab item and press it. Type name Utility
Library (you can type everything what you want, but better to keep real name of
library).
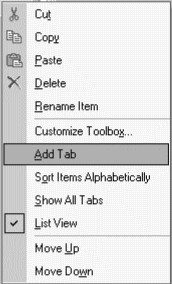
Then click by mouse on newly created tab and again call context menu. Now in
it select Customize Toolbox item.

In the opened dialog select tab .Net Framework Components. On it you
must saw list of installed and known to IDE components. But IDE know about our
newly compiled library nothing. That is why press Browse button and in Open
Dialog select compiled version of Utility Library DLL (for better view apply
sorting on Namespace column).

From that moment the IDE knows about Utility Library and on Toolbox tab you
must saw a list of newly added controls.
How to use Wizard control
Wizard control is based on System.Windows.Forms.UserControl class that
is why IDE can show it more then on one toolbox tab (Don’t worry about that). So
let’s start use of control. First create empty Window Form. Such form will be a
base for our control (parent). Then Open toolbox window and on Utility Library
tab select Wizard Form control. Drag and drop Wizard Form control on our
parent (empty window form).
Window form support all types of Docking, but by default is set none.
So click on control and in properties list in subsection Layout set
property Dock to Fill value.

After that form must look like a picture upper. This is a default view of
wizards. Now we add wizard base, but our wizard still have no any own page.
First of all we must add Wizard Welcome page. To do this simply call context
menu of control or select control and open properties window.


As you saw in context menu we have four wizard actions:
- Add Welcome Page
- Add Page (simple wizard page)
- Add Final Page
- Remove Page
So select Add Welcome Page menu item, and hurrah! Now our wizard has a
Welcome page. Second Action will be to fill well Page properties. Wizard give
you only base and you must by own hands customize it. This is a base:

And this is a customized version of it:

Welcome Page has special properties:
- Description
- Description2
- DontShow
- HeaderImage
- ImageIndex/ImageList
- Title
After customizing first page open context menu and press Add Page item. After
that in Wizard we must have two pages: first is a welcome page and second is a
Standard wizard page (to switch between wizard pages in control use <Back and
Next> buttons. They work in design mode too).

On standard wizard page we have special lines (rectangles in center of page);
they will help us in placing controls according to Wizard 97 standard. By first
line (from left to right) we will place labels. On second Line we will place
controls… and etc. (for more details look into Wizard 97 standard).

Last step of our work will be Final Page creation. By Wizard 97 standard on
final page must be list of done by wizard tasks and status of wizard. To Add
final page select Wizard Form control and from context menu select Add Final
Page item. After page adds our wizard will have three pages: first – welcome
page, second – work page and third – final page.

And after page customizing it will be like:

Code part of wizards
All wizards which based on Wizard Form control have events by which developer
can control wizard work. As you see on picture below all wizard events are
grouped by Wizard Category. Wizard has seven base events. First five events:
Back, Cancel, Finish, Help and Next are events which thrown by wizard form
control on buttons press. Here in more details should be discussed only Next and
Finish events. Any page in wizard has property Finish Page. This is a
Boolean indicator of which button must be shown on bottom of wizard: Next>
or Finish. Also this property control which event will be thrown by
wizard control.

In most cases user must attach delegate-methods only to three events:
Finish, Wizard Closed and Cancel. If algorithm of
wizard not a linear one and has many ways of execution, then also must be used
Next and Back events to control switch between wizard pages.

In sequence diagram you can see process of user interaction with wizard GUI.
As you see Wizard control attach itself to parent form Closing event and
when user press close button dialog act like on Cancel button press.
In more details must be described Finish and Wizard Close
events. Wizard control designed to work well as only one control on form and as
a part of more advanced GUI. That is why process of wizard closing must be
always written by hands. “Wizard Closed” event thrown by control only in
case when “WizardForm” control property “Show Cancel Confirm” is
set to TRUE value. That is why we must make two event handlers: Cancel and “WizardClosed”.
Example of code
private void wizard_Finish(object sender,
UtilityLibrary.Wizards.WizardForm.EventNextArgs e)
{
ITask m_task = null;
if( m_assignedProject == null )
{
m_task = m_application.Tasks.Create( txtShortName.Text, null );
}
else
{
if( lstAssigned.Items.Count == 0 )
{
m_task = m_assignedProject.UnassignedTasks.Create(
txtShortName.Text, m_assignedProject );
}
else
{
m_task = m_assignedProject.Tasks.Create(
txtShortName.Text, m_assignedProject );
foreach( ListViewItem item in lstAssigned.Items )
{
ISecurityUser user =
(ISecurityUser)m_assignedProject.Team.SecurityUsers[ item.Tag ];
m_task.AddAssignment( user );
}
}
}
if( m_task != null )
{
if( comboPriority.SelectedItem != null )
{
m_task.Priority = (int)((ComboItem)comboPriority.SelectedItem).Tag;
}
if( m_request != null )
{
m_task.AssignToRequest( m_request );
}
m_task.Categories.Clear();
foreach( TreeNode catNode in comboCategory.CheckedItems )
{
m_task.Categories.Add( (int)catNode.Tag, catNode.Text );
}
m_task.Deadline = comboDeadline.Value;
m_task.EstimatedTime = int.Parse( txtEstimate.Text );
if( ((RichTextBox)rchComment).Text != "" )
{
IComment comm = m_task.Comments.Create( "Task Comment" );
comm.Text = ((RichTextBox)rchComment).Rtf;
m_task.AddComment( comm );
}
((ITasksCollection)m_task.Parent).UpdateDatabase();
}
Close();
}
private void wizard_Next(object sender,
UtilityLibrary.Wizards.WizardForm.EventNextArgs e)
{
UtilityLibrary.Wizards.WizardPageBase page =
( UtilityLibrary.Wizards.WizardPageBase )sender;
if( page.Equals( wizPageAssign ) && m_assignedProject == null )
{
e.Step = 2;
}
}
private void wizard_Back(object sender,
UtilityLibrary.Wizards.WizardForm.EventNextArgs e)
{
UtilityLibrary.Wizards.WizardPageBase page =
( UtilityLibrary.Wizards.WizardPageBase )sender;
if( page.Equals( wizFinalPage ) == true && m_assignedProject == null )
{
e.Step = 2;
}
}
private void wizard_WizardClosed(object sender, System.EventArgs e)
{
Close();
}
How to create own Wizard Pages
Very often wizards in application have same pages. You can make copy/paste,
but this not a good one solution. That is why creation of custom wizard pages is
a common way to enhance wizard functionality.

First of all let’s look into “WizardPageBase” class inheritors. As you
see Welcome and Final pages are example of how to create custom wizard pages.
Only advantage of this pages that they have IDE integration, which yours page
can not have without control designer code modifications. Process of creation is
simple one. First of all we must create our Page class inherited from
WizardPageBase
class. To do this you can use IDE functionality. Open Solution
Explorer. In it select your project and call context menu. In context menu
select Add ->Add Inherited Control.


In appeared dialog type name of new file, name of file will be used by IDE as
a class name.

Then select class which you want to inherit (IDE will show classes only if
they exist in solution, so if you use Utility Library as stand alone assembly,
then you must create file by hands).
First of add custom page as a form class member:
private CustomWizrdPage wizPageCustom;
Second add new page into designer method:
private void InitializeComponent()
{
...
this.wizPageCustom = new CustomWizrdPage();
...
this.wizard.Pages.Add(this.wizPageCustom);
...
}
and press Shift+F7 to view form in designer. After that you
can do page customization in Visual Studio IDE.
Design Tips and Tricks
To make your wizards up to date and make them look and fill good do such easy
steps:
- Wizard control size must be in 4:3 proportions (width:
height).
- All images and controls must be in one style
- Do not try to place many controls on one page, better
redesign pages and make them in two or three simple steps.
- Add many Hints and help messages to user (in messages
say why and for what you needed user data)
- In application all wizards must in one size
(width/height)
- All text messages keep in resources; this can help in
localization of application.
- Give user freedom in customization (wizard also can have
some settings :)
History
- First revision, 25th April, 2003.