Introduction
As with Neural Networks Fuzzy Logic is something of a black art in that there are few books and articles about it and most of them tend to be extremely specialized. It is a concept that despite it's inherent simplicity is often described using complex mathematical arguments that simply make no sense to the interested observer without a degree in maths. This gives the whole area a degree of mystery that separates it from being a useful tool and strands it in the realm of academics/professionals who seem either unwilling or unable to explain the concepts of Fuzzy Logic in a manner that would be useful to people who want to see what it has to offer without spending years studying. Those that do make the effort seem to think that because everyone else is protecting their knowledge then they should also and cover any information they do give out with restrictive licensing agreements or just basically selling any libraries they may have written.
The Fuzzy Dot Net series of articles intends to break this restrictive chain by publishing a Fuzzy Logic Library that is free to use with no restrictions in whatever context that anyone who comes across the code wishes to use it. The aim of these articles is to not only provide a library but to describe Fuzzy Logic in such a way that as long as the person reading it knows how to program in C# they should be able to follow the articles and be in a position to use the library to write their own Fuzzy Logic enabled code.
Most, if not all programmers have heard the term Fuzzy Logic but few could actually describe what it means and until I read Bart Kosko's "Fuzzy Thinking" book I can't say that I was any more enlightened than any one else in this respect. So I'm going to start with an explanation I gave recently to a friend who has no idea about programming computers at all because it quite simply isn't his field and he doesn't want to know but what the hell if he can rabbit on to me about sport I can try an make him understand the basic concepts behind Fuzzy Logic.
What I told him is that basically Fuzzy Logic is about degrees of correctness, that it is trying to move away from the concept of absolute right or wrong black or white. One of the examples given in Bart Kosko's book is if you have a pile of sand and remove sand a single grain at a time at what point does the pile of sand cease to become a pile and become a lump or a bucket or any term you like that would describe some sand as something smaller than a pile. The simple answer to this is of course when we say so. When the pile of sand reaches a certain weight or only has so many million grains it is no longer a pile. It is whatever we determine it will be, so in code terms we would have something like
while( Sand.IsPile() == true )
{
Sand.RemoveGrain();
}
There is no real specific point at which a pile of sand ceases to be a pile of sand it is down to experience and in truth is totally arbitrary. In the example above the pile of sand ceases to be a pile of sand when the IsPile
function returns false
. From the code above we have no way of knowing when this will be.
To put it another way in this country ( England ) it is accepted that at the age of sixty five you are old enough to retire. Once you retire you automatically become an Old Age Pensioner but if you don't retire you don't become an Old Age Pensioner you are in a sort of middle ground between being Old and not being Old even though at the same time you may well be older than other people who are considered to be old. So the following code is rubbish
if( Person.Age == 65 )
{
Person.Status = Old;
}
You could substitute "Old" for "Retired" but it still would not make the above statement true. There is a reason for the saying that there is always an exception to the rule and that reason is that the rule is usually not flexible enough to be a rule and should instead be considered a general guideline that is mostly true but on occasion completely false. A more realistic interpretation of the above code could be,
if( Person.Age == 65 )
{
Person.PossibleStatus == Old;
Person.Retired = false;
}
The change from an absolute "Status" to a "PossibleStatus" means that in a sense we are right no matter what. If the person is aged 65 then there is a good chance that they are retired and therefore considered old and if the person is still working then although they could possibly be retired in this persons case they have not. It becomes obvious at this point that for a full understanding of the situation we are in need of more information. In the face of the fact that we aren't going to get any more information what we can say about this person that is aged 65 is that there is a good chance that are considered to be old. To give it a figure say that there is a 90% chance that a person aged 65 will be considered old but because in this case out definition of old doesn't depend entirely on age but also on whether or not the person is retired.
Now the next exception to the rule is that most people when they retire stop working and just do whatever it is that retired people do these days, you know the sort of stuff play bowls, moan at kids for being young and stupid enough to still be doing the things they've forgotten they did at that age. Some of course don't retire at all but continue to work in some sort of capacity but for the sake of keeping things simple we'll pretend that we don't know that and then we can have code that goes something like,
if( Person.Old > 90 )
{
if( Person.Retired == true )
{
Person.Status = Old;
}
else
Person.PossibleStatus = Old;
}
This is where we get back to my friend and I having our conversation in the pub and I'm telling him that the main idea is about degrees of correctness, that the whole idea of things being black or white, true or false is an over simplification that we don't live in a world that works according to those rules past a certain point. They may be good generalizations but the point is that from a programming perspective if you are programming with a technique that is only good for generalizations then you are going to reach a point in your coding where you are having difficulty being specific enough to be accurate and at this point I don't mean specific in a true or false way I mean specific in a if a person is sixty five and still working then there is a good chance that they are not retired but they may officially be retired according to one company and collecting their pension and not retired according to another company sort of way.
This could lead one to the conclusion that there is something fundamentally wrong with the way that we are programming and that the very methods that we are using ensure that we will never be able to write truly intelligent programs because they are based on an absolute value system that is too rigid to allow for the complicated definitions that we find in real life. I choose not to go that far but think of Fuzzy Logic as another tool in the armory for effective programming.
First of all as an introduction to the realms of Fuzzy Logic we are going to take a look at the two main classes in the library provided.
The Fuzzy Numbers Class
The Fuzzy Number class is the primary class that implements what I called degrees of correctness above. This is done by the use of three values that are represented within each Fuzzy Number class object. These values are a different way of representing a number and it is important that a clear understanding this concept is gained early on as everything that follows is pretty much built on this stuff.
The concept is this. You start with a number that at any given point you don't actually need to know what that number is, in fact the actual value of the number is not the most important thing about it. The number has two other references that are part of the class these are the maximum value for the number and the minimum value for the number. The idea is that the number itself is somewhere between the maximum and minimum values for the number. There is then a membership indicator that tells you exactly where in between the minimum and the maximum value that the number is. This is done by using a value between 0 and 2, with 1 being the absolute centre. If the value of the number is outside the range of the minimum and the maximum values then the membership value of the number is equal to zero. For the sake of simplicity the Fuzzy Numbers class has a function that calculates the membership value, this is a simplification and there are other ways of implementing it, some ideas can be found in the books in the references section.
The implementation given within the Fuzzy Numbers class is that the value 0 is given if the number is below the minimum or above the maximum values. If the number is above the minimum value and below the centre value then the membership value will be between 0 and 1, in increments rising until it reaches 1. If the value is above the central value and below the maximum then the membership value will be incremented between 1 and 2 changing to 0 as soon as it exceeds the maximum value.
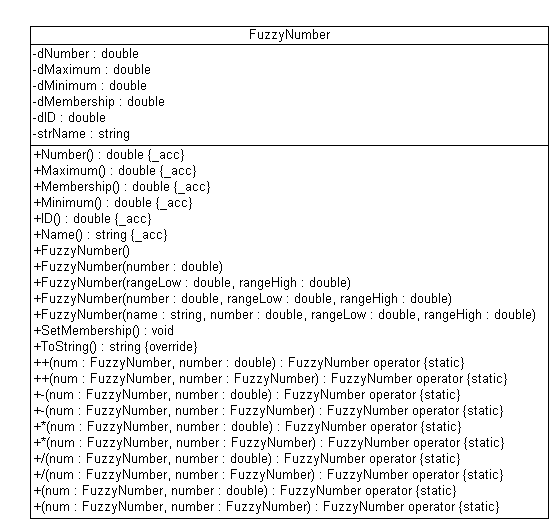
Testing the Fuzzy Numbers Class
The Fuzzy Number class is tested by clicking on the Test Fuzzy Numbers button on the Fuzzy Dot Net Application. This will run through a series of basic tests for the Fuzzy Number class, these tests are fairly basic C# stuff using the constructors and operator overloading, so I wont go through all the details here, but will leave it up to the reader to study the code and the output if they need to. If you do experience any problems understanding what is happening I refer you to the books on C# given in the references section.
The Fuzzy Set Class
The Fuzzy Set class serves as the base class for all fuzzy sets and as such is an abstract class that cannot be instantiated in it's own right. It does however, contain the basic functionality essential to manipulating a range of Fuzzy Numbers. This is done through the use of an array list which stores the Fuzzy numbers and the member functions of the Fuzzy Set class that performs operations on the array. The array list method was chosen in order that there was no limit set by the code on the number of Fuzzy Numbers that could be entered into a Fuzzy Set.
The principal idea behind the Fuzzy Set is that it holds a range of Fuzzy Numbers that can be used for any purpose the implementer of the set requires for example you could use the set to measure different degrees of cold e.g. you would start with a Fuzzy Number that had a minimum value of -10 and a maximum value of +10 which for the sake of argument we will call freezing. This number when it was in perfect balance with between it's minimum and maximum which in this case would be zero and in this case it would just be freezing. One point to note though for a number like this is that as the number approaches it's minimum value the actual temperature that the number represents will grow to be more in keeping with it's name where as the membership value for the number would be dropping towards zero at this point. So it might be conceptually easier for an example like this to rewrite the membership value so that it say climbed the cooler it got and approached zero the warmer it got.
Also as the temperature rose towards its maximum value it would be less of a case of freezing and more of a case of "a bit nippy". Which come to think of it is beginning to make the range of the numbers a bit awkward and should probably be split into two separate Fuzzy Numbers one covering the range between -10 and 0 and another covering the range between 0 and +10 which could be called "below freezing" and "a bit nippy" respectively. Whatever ranges you choose to implement is up to you but some just plain work better than others. There is also the fact that the numbers that are contained within the Fuzzy Set have in no way to be mathematical things like temperatures and such things. You can just as easily have a range of numbers that represent how fat your current boss is, or even if he has gone way beyond being simply fat these days and is heading for truly globular.

The Fuzzy Number Set class derives from the Fuzzy Set class and is used for the implementation of the demonstration code. At present the class just serves to implement the Fuzzy Set class with possible enhancements at a later date.
The Fuzzy Set Parameters class is designed to be a convenient way to change the parameters of the sets when calling one of the set comparison functions. These functions are the Union, Intersection and the ExclusiveOR functions that come in two flavours each. The first is the standard version of the functions and the second is the version of the functions that take a Fuzzy Set Parameters object that will allow the variables within the set to be altered and refined to exclude or include different items within the sets. It should be noted that if you create a Fuzzy Set Parameter object and do not change any of the variables then the results of the function will be the same as if the standard function were used that does not take a Fuzzy Set Parameter.
Testing the Fuzzy Number Set Class
The Fuzzy Number Set class is tested by clicking on the Fuzzy Number Set Test button. This will run through a series of basic tests for the Fuzzy Number Set class, these tests are fairly basic C# and hopefully the output is self explanatory, so I wont go through all the details here, but will leave it up to the reader to study the code and the output if they need to. If you do experience any problems understanding what is happening I refer you to the books on C# given in the references section.
Finally
This brings to an end the introduction to Fuzzy Logic, the sample code for this article is a simple test harness for the classes and is not meant to be anything else. In the second part of this series we will look at a sample program that produces a simplified model of a heating system and uses the ideas an classes given in this article.
History
- 01 August 2003 :- Initial release.
- 08 August 2003 :- added note and link to next article
Note
The last article in the series contains the latest code for the library. No attempt at backward compatibility will be attempted and I will change the library as I see fit.
Link To Next Article
References
- Tom Archer ( 2001 ) Inside C#, Microsoft Press
- Jeffery Richter ( 2002 ) Applied Microsoft .NET Framework Programming, Microsoft Press
- Charles Peltzold ( 2002 ) Programming Microsoft Windows With C#, Microsoft Press
- Robinson et al ( 2001 ) Professional C#, Wrox
- Bart Kosko ( 1994 ) Fuzzy Thinking, Flamingo
- Buckley & Eslami ( 2002 ) An Introduction To Fuzzy Logic And Fuzzy Sets, Physica-Verlag
- Earl Cox ( 1999 ) The Fuzzy Systems Handbook, AP Professional
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.