Introduction
There are many articles and examples about limiting the number of characters allowed in a textbox, that can be found on the Web. But how is it possible to count and - if needed - to limit the number of lines in a textbox? Here is a solution using client-side JavaScript to limit the content of a TextArea
to a certain number of characters as well as to a certain number of lines (not necessarily equal to the number of visible rows of the TextArea
).
Textarea limits
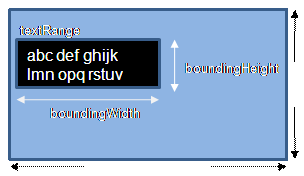
We can define the visible width and height of a TextArea
either using the cols
and rows
attributes or the style properties: width
and height
, but we cannot set a limit to the number of characters or the number of lines for the text entered into the TextArea
by using HTML or CSS. Fortunately we can create a textRange
object for the content of the TextArea
. This JavaScript object has a read-only property boundingHeight
, from which we can retrieve the height of the textRange
as a pixel value. So we only have to divide the boundingHeight
of the textRange
by the lineHeight
(as pixel value) of the TextArea
to get the number of lines used in the TextArea
.
Example

Definition of the TextArea
<textarea name="myText" id="myText" cols="44" rows="4" wrap="virtual"
style="font-family:arial; font-size:14px; line-height:17px; height:77px; overflow:auto;"
onKeyUp="checkLimits(myForm.myText,myForm.myChars,myForm.myLines);"
onPaste="checkLimits(myForm.myText,myForm.myChars,myForm.myLines);"
onCut="checkLimits(myForm.myText,myForm.myChars,myForm.myLines);"
onBlur="checkLimits(myForm.myText,myForm.myChars,myForm.myLines);">
Text in the limited
textarea may never exceed the defined maximum number of rows and/or characters.
</textarea>
Attributes
We assign a name
and an id
to our TextArea
and we define its visible size (rows
and cols
). It is recommended to set the wrap
property to "virtual" or "physical" or "hard", but it should not be set to "off".
Style properties
font-family
: we may use a proportional font (e.g., Arial) or a monospace font (e.g., Courier). In the first case, we will rather reach the character limit; in the second case, the line limit will mostly be reached first.font-size
: We need to know the font-size to choose a proper line-height and to compute the height of the TextArea
, if we wish to show all the allowed lines without a scrollbar (overflow:auto
).line-height
: We have to set the line-height in the TextArea
to a pixel value corresponding with the given font-size.height
: If we want to show all the rows of the TextArea
without a scrollbar, we have to set the height to at least (rows*line-height)+(line-height/2)
. But, we are free to omit this property or to choose any other suitable height for the visible part of our textbox.
Event handlers
onKeyUp
is the main event to call the script checkLimits
to check the limits of the TextArea
when text is entered or deleted using the keyboard.onPaste
and onCut
call the script checkLimits
when text is pasted into or deleted from our TextArea
.onBlur
does a last check when our TextArea
loses focus.
You are free to use other event handlers, e.g., onKeyPress
, etc., depending on the needs of your application. The TextArea
may be empty or contain some default text, as is shown in the example.
The example page contains four input fields to show the actual and the maximum numbers of characters and lines in the TextArea
.
<input name="myChars" id="myChars" type="text"
style="text-align:center; border-width:0px;" value="0"
size="4" maxlength="4" readonly="readonly">
<input name="maxChars" id="maxChars" type="text"
style="text-align:center; border-width:0px;" value="0"
size="4" maxlength="4" readonly="readonly">
<input name="myLines" id="myLines" type="text"
style="text-align:center; border-width:0px;" value="0"
size="4" maxlength="3" readonly="readonly">
<input name="maxLines" id="maxLines" type="text"
style="text-align:center; border-width:0px;" value="0"
size="4" maxlength="3" readonly="readonly">
The code
<script type="text/javascript">
<!--
The function getLines
is called by the function checkLimits
to compute the number of lines used in the TextArea
. The variable lineHeight
is set to the numeric value of the style property line-height
of our TextArea
. The variable tr
represents the text in our TextArea
and holds its dimensions as bounding properties. We divide the property boundingHeight
by lineHeight
to get the number of lines our text occupies.
function getLines(txtArea){
var lineHeight = parseInt(txtArea.style.lineHeight.replace(/px/i,''));
var tr = txtArea.createTextRange();
return Math.ceil(tr.boundingHeight/lineHeight);
}
The main function checkLimits
is called with three parameters by the events: body.onload
, textarea.onKeyUp
, textarea.onCut
, textarea.onPaste
, and textarea.onBlur
:
myForm.myText = name of the textarea
myForm.myChars = name of the field where we display the actual number of characters
myForm.myLines = name of the field where we display the actual number of lines
function checkLimits(txtArea,countChars,countLines){
In the example, the values for maxLines
and maxChars
are derived from the visible size of the TextArea
, but we could choose any other suitable value. We set the counter countChars
to the length of the txtArea.value
and the counter countLines
to the value returned by the function getLines(txtArea)
, and display them on the page in the fields myForm.myChars
and myForm.myLines
, and we also display the limits for lines and characters on our page.
var maxLines = txtArea.rows;
var maxChars = txtArea.rows * txtArea.cols;
countChars.value = txtArea.value.length;
countLines.value = getLines(txtArea);
document.myForm.maxLines.value = maxLines;
document.myForm.maxChars.value = maxChars;
First, we check if the maxChars
or the maxLines
limit was reached and the user entered a line break or new line. In this case, we have to shorten the content of the TextArea
until none of the limits is exceeded and interrupt further input by an alert.
if((txtArea.value.length >= maxChars || getLines(txtArea) >= maxLines)
&& (window.event.keyCode == 10 || window.event.keyCode == 13))
{
while(getLines(txtArea) > maxLines)
txtArea.value = txtArea.value.substr(0,txtArea.value.length-2);
while(txtArea.value.length > maxChars)
txtArea.value = txtArea.value.substr(0,txtArea.value.length-2);
alert("chars and / or lines limit reached");
}
If more characters are entered than maxChars
allows, the length of the TextArea
is automatically reduced until its length is equal to TextArea
and an alert is issued.
else if(txtArea.value.length > maxChars )
{
while(txtArea.value.length > maxChars)
{
txtArea.value = txtArea.value.substr(0,txtArea.value.length-1);
}
alert("chars limit reached");
}
Also, if the text exceeds the lines limit, it is automatically reduced until the number of lines is equal to maxLines
and an alert is issued. There is one thing to mention. The limit is checked after the input has been processed. Therefore, in this moment, a vertical scrollbar is displayed by the browser, and the text in the last line is reduced by some more characters. To avoid this loss of input, we should set the height of the TextArea
to at least ((rows + 1) * lineHeight)
.
else if (f (countLines.value > maxLines)
{
while(countLines.value > maxLines)
{
txtArea.value = txtArea.value.substr(0,txtArea.value.length-1);
}
alert("lines limit reached");
}
Finally, the counters are updated.
countChars.value = txtArea.value.length;
countLines.value = getLines(txtArea);
}
</script>
Conclusion and shortcomings
The script was tested only with Internet Explorer V7.0. In some cases, it would be sufficient to limit the lines of the TextArea
. E.g., we only need to count the characters if the text has to fit into a database field. Further on, we could make use of the property boundingWidth
to limit the width of the TextArea
without automatic line breaks by the browser.
If you like this article, vote for it!
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.