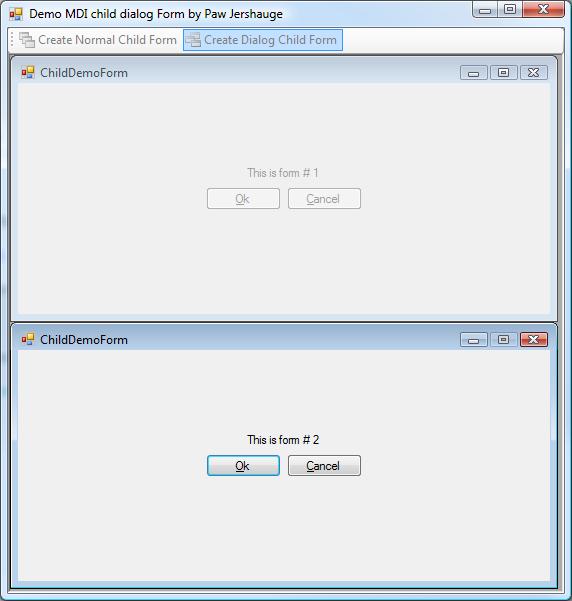
Introduction
Ever had the need to block all other forms and controls when a specific form is shown as a dialog? This is a very simple workaround, for an MDI modal form.
Background
I was designing an MDI rich application, where I needed a dialog form showing only in the parent form, and blocking the rest of the application, until the user selected something on the dialog form.
Using the code
The code is very, very simple; just inherit the MDIParent
form on your MDI parent form instead of the normal form inheritance.
public partial class Your_MDI_Parent_Form : MdiParent
To show a form as a dialog and block other controls, call the ShowChildDialog
method on the parent form, like this:
ChildForm frm = new ChildForm();
ShowChildDialog(frm, ChildForm_DialogReturned);
And, to receive the DialogResult
from the child form, use the following event receiver:
private void ChildForm_DialogReturned(object sender, DialogResultArgs e)
{
MessageBox.Show("ChildForm returned: " + e.Result.ToString());
((Form)sender).Dispose();
}
Explanation of the code
The ShowChildDialog method
public void ShowChildDialog(Form frm,
EventHandler<DialogResultArgs> DialogReturnedValue)
{
frm.MdiParent = this;
frm.MaximizeBox = false;
frm.FormClosed += new FormClosedEventHandler(frm_FormClosed);
callingsender = frm;
DialogReturning += DialogReturnedValue;
DisableControls();
frm.Show();
}
- Set the MDI parent so the form is shown within the parent.
- Remove the Maximize button on the child form.
- Add an event receiver for the
Closed
event on the child form; this will be used to return the dialog result from the child dialog form. callingsender
is for internal use, so the code knows which form it is dealing with.- Add an event receiver for the returned dialog result.
- Calling the
DisableControls
method will disable all other controls and forms in the MdiParent
. - Last, show the form. This will be the only one enabled.
The DisableControls method
private void DisableControls()
{
for (int i = 0; i < this.Controls.Count; i++)
{
if (this.Controls[i].GetType() != typeof(MdiClient))
this.Controls[i].Enabled = false;
}
foreach (Form frm in MdiChildren)
frm.Enabled = false;
if(callingsender != null)
callingsender.Enabled = true;
}
- Loop through all the controls of the MDI parent and disable them if it not an MDI child control.
- Loop through all the child forms and disable them.
- If
callingsender
is not null
, then enable it again.
The ForceReleaseOfControls method
public void ForceReleaseOfControls()
{
for (int i = 0; i < this.Controls.Count; i++)
this.Controls[i].Enabled = true;
foreach (Form frm in MdiChildren)
frm.Enabled = true;
}
- Loop through all the controls and enable them again.
- Loop through all the child forms and enable them again.
What the code does not do...
This code does not check for the pre-enabled-state of controls and forms. Therefore, it will not remember what enable state the control/form was before, and set it back to that. This can be achieved pretty simply by having a collection of keys and values. But for now, this is the code. Hope it helps those who have the same issues as I did.
Point of interest
I learnt something new about MDI applications.
History
- 21. Dec. 2009: First post.
- 21. Dec. 2009 (later): Updated the article with some explanation of the code.