Couple of days ago I needed to deploy a WCF RESTfull service on IIS6. Before then I never deployed RESTfull service on IIS6 so it came up with quite configurations for me. While I was deploying this web service on IIS6, I as usual Goggled for some configuration related help, although I found, but most of the stuff were scattered. I mean I didn’t find all in a single post or a blog. So I thought why couldn’t do it.
In this post I developed a WCF RESTfull web service from scratch to hosting on web server and consuming by jQuery ajax. Here is the service contract. You’ll find UriTemplate with both with parameters and without parameter.
[ServiceContract(Name = "DeepASPService", Namespace = "deepasp.wordpress.com")]
interface IDeepASPService
{
[OperationContract]
[WebGet(UriTemplate = "/GetArticles?blogName={blogName}", ResponseFormat = WebMessageFormat.Json, BodyStyle = WebMessageBodyStyle.Bare)]
List<Article> GetAllArticles(string blogName);
[OperationContract]
[WebGet(UriTemplate = "/GetTagArticles?tagName={tagName}", ResponseFormat = WebMessageFormat.Json, BodyStyle = WebMessageBodyStyle.Bare)]
List<Article> GetArticlesByTagName(string tagName);
[OperationContract]
[WebGet(UriTemplate = "/GetArticle?Id={Id}", ResponseFormat = WebMessageFormat.Json, BodyStyle = WebMessageBodyStyle.Bare)]
Article GetArticleById(string Id);
[OperationContract]
[WebGet(UriTemplate = "/SaveArticle?authenticationToken={authenticationToken}&title={title}&body={body}&submittedBy={submittedBy}", ResponseFormat = WebMessageFormat.Json, BodyStyle = WebMessageBodyStyle.Bare)]
bool SaveArticle(string authenticationToken, string title, string body, string submittedBy);
}
Here is the Service implementing above contract. Most of the operations are only defined and not fully implemented as it didn’t need for this post. GetArticles operation returns a generic list in json format.
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class DeepASPService : IDeepASPService
{
public List<Article> GetAllArticles(string blogName)
{
return new List<Article>()
{
new Article { Id = Guid.NewGuid(), Name=blogName, Title = "WCF RESTfull web service.", Body = "Html body.", Comments=new List<Comment>()
{
new Comment { Id = Guid.NewGuid(), CommentedOn = DateTime.Now, DisplayName = "deepasp.wordpress.com", Email="no-emailId@deepasp.wordpress.com", Replies = null }
}},
new Article { Id = Guid.NewGuid(), Name=blogName,Title = "WCF RESTfull web service deploying on IIS 6.", Body = "Html body.", Comments=new List<Comment>()
{
new Comment { Id = Guid.NewGuid(), CommentedOn = DateTime.Now, DisplayName = "deepasp.wordpress.com", Email="no-emailId@deepasp.wordpress.com", Replies = new List<Comment> ()
{
new Comment { Id = Guid.NewGuid(), CommentedOn = DateTime.Now, DisplayName = "deepasp.wordpress.com", Email="no-emailId@deepasp.wordpress.com", Replies =null }
}
}
}},
};
}
public List<Article> GetArticlesByTagName(string tagName)
{
throw new NotImplementedException();
}
public Article GetArticleById(string Id)
{
throw new NotImplementedException();
}
public bool SaveArticle(string authenticationToken, string title, string body, string submittedBy)
{
if (AuthenticateRequest(authenticationToken))
{
}
return true;
}
private bool AuthenticateRequest(string authenticationToken)
{
return true;
}
}
In this sample application there are only two data contracts but you can have as many data contracts as you need.
DataContracts:
[Serializable]
[DataContract]
public class Article
{
[DataMember]
public Guid Id { get; set; }
[DataMember]
public string Name { get; set; }
[DataMember]
public string Title { get; set; }
[DataMember]
public string Body { get; set; }
[DataMember]
public List<Comment> Comments { get; set; }
}
[Serializable]
[DataContract]
public class Comment
{
[DataMember]
public Guid Id { get; set; }
[DataMember]
public string DisplayName { get; set; }
[DataMember]
public string Body { get; set; }
[DataMember]
public DateTime CommentedOn { get; set; }
[DataMember]
public string Email { get; set; }
[DataMember]
public List<Comment> Replies { get; set; }
}
Configurations:
Service.svc
Service="Namespace.serviceName" Factory="System.ServiceModel.Activation.WebServiceHostFactory"
web.config:
<configuration>
<system.web>
<compilation debug="true" targetFramework="4.0" />
</system.web>
<system.serviceModel>
<serviceHostingEnvironment multipleSiteBindingsEnabled="true" />
<services>
<service name="Namespace.ServiceName" behaviorConfiguration="ServiceBehavior">
<endpoint binding="webHttpBinding" contract="Namespace.InterfaceName"
behaviorConfiguration="webHttp"/>
</service>
</services>
<behaviors>
<serviceBehaviors>
<behavior name="ServiceBehavior" >
<serviceMetadata httpGetEnabled="true" />
<serviceDebug includeExceptionDetailInFaults="false" />
</behavior>
<behavior>
<!-- To avoid disclosing metadata information, set the value below to false and remove the metadata endpoint above before deployment -->
<serviceMetadata httpGetEnabled="true"/>
<!-- To receive exception details in faults for debugging purposes, set the value below to true. Set to false before deployment to avoid disclosing exception information -->
<serviceDebug includeExceptionDetailInFaults="True"/>
</behavior>
</serviceBehaviors>
<endpointBehaviors>
<behavior name="webHttp">
<webHttp/>
</behavior>
</endpointBehaviors>
</behaviors>
</system.serviceModel>
<system.webServer>
<modules runAllManagedModulesForAllRequests="true"/>
</system.webServer>
</configuration>
Now we will host this service on IIS. So here is the step by step procedure;
1-Right click on website go to New
2-Click Create virtual directory(in this sample code I created a virutal directory in an existing website)
3- A wizard screen will come up like;
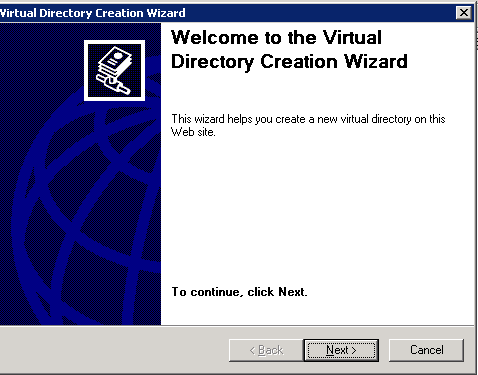
4-Click Next
5-In Alias textbox give any name for your service e.g. TestRESTfullService
6-Next and browse the path where your service is e.g. wwwroot\RESTfullWCFService
7-Next
8-Next
9-FINISH
Now although you have successfully deployed your service on IIS but hereafter configurations are required to run it properly.
1-Right click on the new virtual directory you created(e.g. TestRESTfullService)
2-Go to properties
3-By default this virtual directory will be shown as a website(globe logo will appear before it) so you need to convert this into an application.
4-Click on Create application button as showing at #1 in the below picture. After creating application your service icon will change from globe to gear.
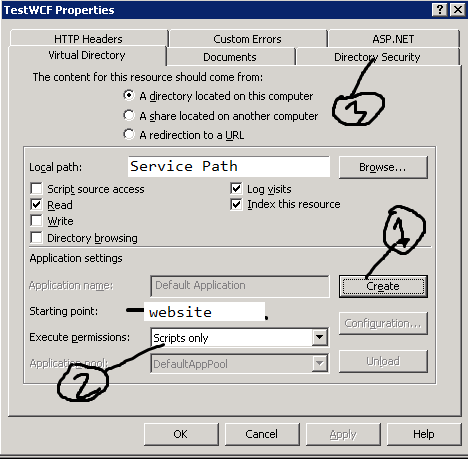
figure 2
5-Change execute permissions from None to Scripts only like the process 2 in the above picture
6- Click on Configuration button and you’ll see this screen;
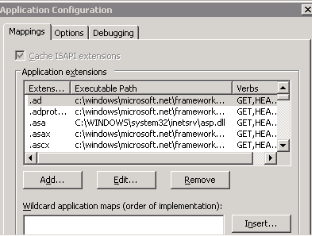
7-Click on Insert and you’ll see a small screen like this;
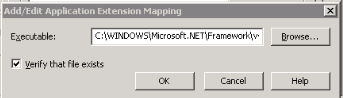
8-Click on Browse and select aspnet_isapi.dll from C:\WINDOWS\Microsoft.NET\Framework\v4.0.30319\aspnet_isapi.dll. If you don’t find v4.0.30319 folder and it means you don’t have dotnet framework 4 full version. So you’ll have to download it from microsoft website and install it. After installation complete you’ll find this so then select it.
9- DO NOT FORGET to uncheck “Verify that file exists” checkbox from the above screen.
10- Now click on Directory Security tab from figure 2.
11- In this Authentication Methods screen you will uncheck “Integrated windows authentication” checkbox.
12- Click OK.
13-Now go to ASP.Net tab from figure 2 and select ASP.Net version : 4.0.30313. (Sometimes a message will appear when version is changed so just press OK).
14- Now restart your IIS. It will lose all sessions of websites hosted on this server so be cautious, its better to do at night time.
14-That’s all you needed.You’ve done it !
Your WCF RESTfull service must be running
Now its time to consume your service. Here we will consume using jQuery ajax like;
function GetAllArticles() {
var url = "/TestRESTfullService/ServiceName.svc/GetArticles?blogName=deepasp";
$(function() {
$.ajax({
type: "GET",
url: url,
async: true,
dataType: "json",
success: function(articles) {
if (msg != null) {
document.getElementById("response").innerHTML = ConvertObjecToHtml(articles);
}
else{
document.getElementById("response").innerHTML ="No articles published yet on this blog";
}
},
error: function(e) {
alert("Error occured while processing service request" + e.responseText);
}
});
});
}
Here download the source code;
CodeProject
