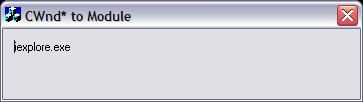
Introduction
Recently, our company decided to produce a time management application. It was not enough for us to just know when people clocked in and clocked out, we wanted to know what they did while they were there. We decided that we would produce a timekeeping application which would allow us to track exactly what the user is doing while logged in. Initially, we thought we would record the title of the active window every second. This provided us more information than we wanted to know as well as made it cumbersome to do reporting on the data. We then decided it would be best to record just the executable name. I looked around for an article that I had seen before on how to do this, but it was not designed to run once a second. So I wrote this function which allows you to easily convert a CWnd*
to the EXE name.
Using the code
The code is simple to use. The function GetWindowModuleName
is passed a CWnd*
and it returns the name of the executable.
#include "Psapi.h"
CString GetWindowModuleName(CWnd *pWnd)
{
HMODULE* lphModule;
char FileName[1024];
DWORD procid = 0;
DWORD modulesize = 0;
BOOL bInheritHandle = false;
if(pWnd != NULL){
GetWindowThreadProcessId(pWnd->m_hWnd,&procid);
HANDLE process = OpenProcess(PROCESS_ALL_ACCESS |
PROCESS_QUERY_INFORMATION, bInheritHandle,procid);
if(process != NULL){
lphModule = new HMODULE[1];
if(EnumProcessModules(process,lphModule,
(sizeof(HMODULE)),&modulesize) != 0){
GetModuleBaseName(process,lphModule[0],FileName,1024);
CloseHandle(process);
delete lphModule;
return FileName;
}
delete lphModule;
}
CloseHandle(process);
}
return "";
}
How does it work?
The function first calls the GetWindowThreadProcessId
to get the current process ID. From there, we open the process and enumerate the process modules. The trick here is to send the EnumProcessModules
function an array of HMODULE
of length one. This will pull only the first module of the process which is the executable. Do not forget to include "Psapi.h" or link to Psapi.Lib.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.