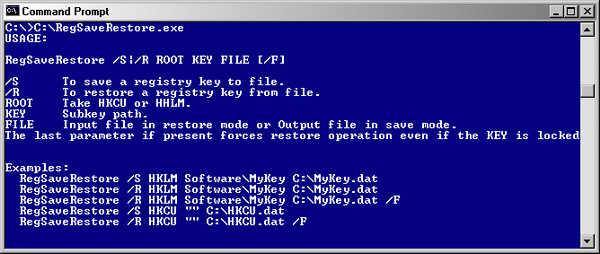
What's new in this update
Introduction
First of all, I apologize for my bad English. This article shows how to save and restore registry keys using the Windows API functions: RegSaveKey
and RegRestoreKey
respectively and provides a tool to achieve these two tasks.
According to MSDN, these two API functions require two privileges, namely SE_BACKUP_NAME
(SeBackupPrivilege
) and/or SE_RESTORE_NAME
(SeRestorePrivilege
) even if the current process token has administrator rights. As a consequence, the tool presented in this article should be used by an administrator account.
These are some situations where the article's attached utility program can be used:
- Profiles manager for only important parts of the registry that must be saved and restored and not necessarily the whole user registry.
- Printers manager to save and restore users' printer configurations in the OS migration process, for example.
- Software configuration. Instead of using .REG files by exporting registry keys and then importing them, we can save keys to files using this utility and when it's time to configure the software, we restore the produced output files to the registry.
How to use the tool
Syntax:
This is the tool syntax as indicated in the picture above:
RegSaveRestore /S|/R ROOT KEY FILE [/F]
/S To save a registry key to file.
/R To restore a registry key from file.
ROOT Take HKCU or HHLM.
KEY Subkey path.
FILE Input file in restore mode or Output file in save mode.
/F This parameter can be used optionnally to force restoring operation.
The tool returns 0 if success, otherwise it returns 1 without any consideration of the real error code.
Examples:
These are two simple examples to show how to use the tool. The first one saves the key HKLM\Software\MyKey (with all MyKey subkeys and values) to the file C:\MyKey.dat and the second one restores this file in force mode to the same key HKLM\Software\MyKey. Make sure that you are not editing by using Regedit or any other way; otherwise the program will fail by the Access denied error, since Regedit locks the key in edit mode.
Saving HKLM\Software\MyKey in C:\MyKey.dat: | RegSaveRestore /S HKLM Software\MyKey C:\MyKey.dat |
Restoring HKLM\Software\MyKey using C:\MyKey.dat: | RegSaveRestore /R HKLM Software\MyKey C:\MyKey.dat /F |
Saving HKLM\Software in C:\Software .dat: | RegSaveRestore /S HKLM Software C:\Software.dat |
Restoring HKLM\Software using C:\Software.dat: | RegSaveRestore /R HKLM Software C:\Software.dat /F |
Remark:
The tool does not provide any means to secure backup files for the restore phase. To be more precise, suppose that MyKet.dat has been provided by Example 1., and then the file has been changed incorrectly or corrupted, then when it comes the time to restore it, the tool will fail, and worst, the key HKLM\Software\MyKey will be deleted automatically when RegRestoreKey
is called. I hope that I will present in a future article an easy way to make such a task 99.99% secure! In order to make it secure and automatic, we have to answer two basic questions:
- Which file goes to which key?
- How to be sure that the file going to a corresponding key is the right one?
Using the code
The tool project is a console MFC application and it can be freely changed to MFC by making some minor changes. The function used to save the registry key to a file is:
BOOL SaveRegKeyPath(CString &Root, CString &SubKey, CString &OutFile)
{
BOOL ret=TRUE;
HKEY hKey=NULL;
DWORD d;
HKEY hRoot;
SetPrivilege(SE_BACKUP_NAME,TRUE);
hRoot=(Root.CompareNoCase(HKCU)==0)?HKEY_CURRENT_USER:HKEY_LOCAL_MACHINE;
if (RegOpenKeyEx(hRoot, SubKey,0,KEY_READ, &hKey)==ERROR_SUCCESS) {
if (IsFileExist(OutFile)){
if (DeleteFile(OutFile))
d=RegSaveKey(hKey,OutFile,NULL);
} else d=RegSaveKey(hKey,OutFile,NULL);
if (d!=ERROR_SUCCESS)
ret=FALSE;
RegCloseKey(hKey);
}
else {
if (IsKeyExist(hRoot,SubKey)==FALSE)
d=ERROR_FILE_NOT_FOUND;
else d=0;
ret=FALSE;
}
SetPrivilege(SE_BACKUP_NAME,FALSE);
return ret;
}
The function used to restore the registry key from a file is:
BOOL LoadRegKeyPath(CString &Root, CString &SubKey, CString &InFile, BOOL Force)
{
BOOL ret=TRUE;
HKEY hKey=NULL;
DWORD d;
HKEY hRoot;
SetPrivilege(SE_RESTORE_NAME,TRUE);
SetPrivilege(SE_BACKUP_NAME,TRUE);
hRoot=(Root.CompareNoCase(HKCU)==0)?HKEY_CURRENT_USER:HKEY_LOCAL_MACHINE;
if (!IsFileExist(InFile)) {
d=ERROR_FILE_NOT_FOUND;
PrintError(d);
ret=FALSE;
}
else {
HKEY hhKey;
char lpClass[80];
DWORD lpDisposition=0;
if (RegCreateKeyEx(hRoot,SubKey,0,lpClass, REG_OPTION_BACKUP_RESTORE,
KEY_ALL_ACCESS, NULL, &hhKey,
&lpDisposition)==ERROR_SUCCESS) {
d=RegRestoreKey(hhKey,InFile,
(Force==FALSE)?REG_NO_LAZY_FLUSH:REG_FORCE_RESTORE);
RegCloseKey(hhKey);
if (d!=ERROR_SUCCESS) {
PrintError(d);
ret=FALSE;
}
} else ret=FALSE;
}
SetPrivilege(SE_RESTORE_NAME,FALSE);
SetPrivilege(SE_BACKUP_NAME,FALSE);
return ret;
}
I invite readers to go into the source code to take a look at the intermediate functions SetPrivilege
, IsKeyExist
and IsFileExist
used in the code above.
Conclusion
I hope that you have learned some thing new in this article and the accompanying tool will be of help for you. As I said before, I hope that in a future article I will provide a secure method to use this tool. In fact, the article should just answer the two questions above.
History
- Version 1.0.0.1 - August 1, 2004
- Version 2.0.0.0 - November 16, 2005
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.