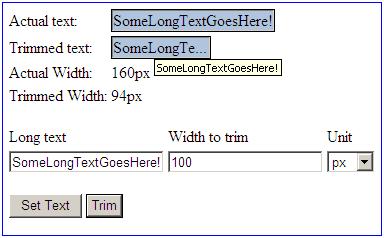
Introduction
This article describes how to trim an HTML text when the control's width is fixed. One problem with HTML controls holding text is that we can make either the height or width property to be fixed, leaving the other to grow. Sometimes when controls with long text grow, they lead to alignment issues.
There is a solution to this problem, displaying only a portion of the text, say, the first 50 characters. This solution has a problem when we fix the size of the control depending on some parameter each time the page is loaded.
So I had to do some R&D to find another solution. Finally I found out a way which serves the purpose: trimming the text to the given width, attaching "..." in the end, and showing the complete text in tool tip.
Background
The basic idea behind is to find out the width of the text which fits into the given control.
First, a span
control is created dynamically with properties same as the original one. Starting with the original text in it, the characters in the span
are reduced one by one until the width of the dynamic span
becomes lesser or equal to the desired width. Finally, this trimmed text is assigned to the original span
and the dynamically added control is removed.
Using the code
Creating a control dynamically is very simple. Create the object using the document.createElement("DIV")
method with the desired tag name as parameter, and call the objDiv.appendChild(objSpan)
method to append to a parent control, in this case, objDiv
.
var objDiv = document.createElement("DIV");
var objSpan = document.createElement("SPAN");
objDiv.appendChild(objSpan);
ctrl.offsetParent.appendChild(objDiv);
The next challenge is to find out the width of the text. The width of the text depends on the style of the control. So when the control is created, all the properties which influence the width are copied and the control is added to the parent control of the original span
control, that is, ctrl.offsetParent
. By this, all the properties which are not assigned but inherited from the parent tags are also copied. Then, we assign some text to objSpan
whose width is to be determined. Now, the width of objSpan
will give the actual width of the text on the browser.
The desired width can be given in any unit which will be converted into pixels easily. We can use spanConvert.style.width
to assign width in a specific unit. But, when we get spanConvert.offsetWidth
, we will get the equivalent width in pixels.
The next step is to trim the text which fits into the desired width. For this, one character from the end is removed each time until the width becomes lesser or equal to the desired width.
while(widthTemp > widthFinal && lengthText > 0)
{
lengthText--;
textTrimmed = textOrigional.substring(0, lengthText);
objSpan.innerHTML = textTrimmed;
widthTemp = objSpan.offsetWidth;
}
In the above code, textTrimmed
will have the trimmed text, and attaching "..." to it will give the text to be displayed on the screen.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.