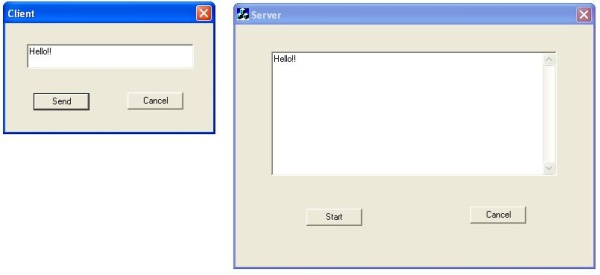
Introduction
This is a simple application demonstrating a UDP client server in MFC. There are many examples related to TCP, but very few for UDP. So, I thought of making a simple application and uploading it here.
Background
The main dialog acts as a server. The server will keep on waiting for data on a particular port, it receives the data and then displays it. The client is started on clicking a Start button of the main dialog. It can send data to the server. The server then receives the data and displays it.
Using the code
The main dialog class, CSimpleUDPDlg
, acts as a server. A thread is started in OnInitDialog()
of the main dialog to receive the data sent by the client.
Code snippets of CSimpleUDPDlg.cpp and CClientSend.cpp are shown below:
#include "afxsock.h"
HANDLE thr;
unsigned long id1;
UINT ReceiveData(LPVOID pParam)
{
CSimpleUDPDlg *dlg=(CSimpleUDPDlg*)pParam;
AfxSocketInit(NULL);
CSocket echoServer;
if (echoServer.Create(514, SOCK_DGRAM, NULL)== 0) {
AfxMessageBox("Create() failed");
}
for(;;) {
SOCKADDR_IN echoClntAddr;
int clntAddrLen = sizeof(echoClntAddr);
char echoBuffer[ECHOMAX];
int recvMsgSize = echoServer.ReceiveFrom(echoBuffer,
ECHOMAX, (SOCKADDR*)&echoClntAddr, &clntAddrLen, 0);
if (recvMsgSize < 0) {
AfxMessageBox("RecvFrom() failed");
}
echoBuffer[recvMsgSize]='\0';
dlg->m_edit.ReplaceSel(echoBuffer);
dlg->m_edit.ReplaceSel("\r\n");
}
BOOL CSimpleUDPDlg::OnInitDialog()
{
CDialog::OnInitDialog();
ASSERT((IDM_ABOUTBOX & 0xFFF0) == IDM_ABOUTBOX);
ASSERT(IDM_ABOUTBOX < 0xF000);
CMenu* pSysMenu = GetSystemMenu(FALSE);
if (pSysMenu != NULL)
{
CString strAboutMenu;
strAboutMenu.LoadString(IDS_ABOUTBOX);
if (!strAboutMenu.IsEmpty())
{
pSysMenu->AppendMenu(MF_SEPARATOR);
pSysMenu->AppendMenu(MF_STRING, IDM_ABOUTBOX, strAboutMenu);
}
}
SetIcon(m_hIcon, TRUE); SetIcon(m_hIcon, FALSE);
thr=CreateThread(NULL,0,(LPTHREAD_START_ROUTINE)ReceiveData,this,NULL,&id1);
return TRUE; }
void CSimpleUDPDlg::OnStart()
{
CClientSend send;
send.DoModal();
}
#include "afxsock.h"
void CClientSend::OnSend()
{
AfxSocketInit( NULL);
CString Buffer;
m_editsend.GetWindowText(Buffer);
int buflen=strlen(Buffer);
CSocket echoClient;
if (echoClient.Create(0,SOCK_DGRAM,NULL) == 0) {
AfxMessageBox("Create() failed");
}
if (echoClient.SendTo(Buffer, buflen,514,
(LPCSTR)"localhost", 0) != buflen) {
AfxMessageBox("SendTo() sent a different number of bytes than expected");
}
echoClient.Close();
}
Well, this is my first article, and I hope it would be helpful.