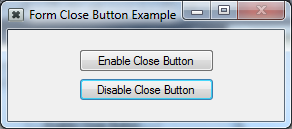
Introduction
The main purpose of this application is to enable and disable the "Close" button of any Windows Form on your application by using Windows APIs.
In some of my applications, I had to prevent users from clicking the close button to exit an application. Of course, there are various ways to exit an application. But in some cases,
clicking the close button may not be the nicest option.
The application uses user32.dll Windows API to invoke some methods. Of course, there are other methods that can be invoked in the same library in order
to perform different actions.
Using the Code
There are plenty of ways to enable and disable the close button in Windows Forms. This one is a simple and probably the most effective of all.
To manipulate the state of the close button in your form, as mentioned before, a Windows API named user32.dll
is needed. By including user32.dll in your project, you will be able to invoke the methods in the "user32.dll" library.
There are two main methods we need to use to change the state of the close button, which are: EnableMenuItem()
and GetSystemMenu()
.


As you can see, these methods are located in the user32.dll file.
After adding using System.Runtime.InteropServices;
to your using
section, you can start importing functions to your project.


After this step, it's time to create the methods and invoke them in your project. Now, you can invoke those methods in any way you want, like using it in a timer,
or any kind of other events. In the demo application, you'll notice that the button events call the custom created void
functions which contain other methods
for enabling and disabling the close button. The snippet below shows invoking the DLL methods.
In order to change the status of the close button, we need to tell the API the current and target addresses of the button's states. These three values represent
the states of the button. All of the values can be found on the internet, if you are interested in manipulating other controls.
internal const int SC_CLOSE = 0xF060;
internal const int MF_ENABLED = 0x00000000;
internal const int MF_GRAYED = 0x1;
internal const int MF_DISABLED = 0x00000002;
Now it's time to call our DLL's methods:
[DllImport("user32.dll")]
private static extern IntPtr GetSystemMenu(IntPtr HWNDValue, bool isRevert);
[DllImport("user32.dll")]
private static extern int EnableMenuItem(IntPtr tMenu, int targetItem, int targetStatus);
Here are two others that I didn't have a chance to try, but I don't see any reason for them to not work properly...
internal const int SC_MINIMIZE = 0xF020;
internal const int SC_MAXIMIZE = 0xF030;